Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial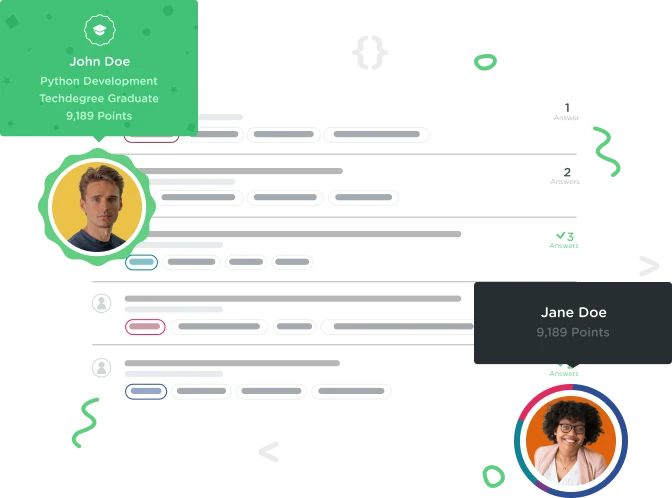
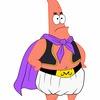
<noob />
17,062 Pointsquestions about challnage 4
def most_courses(dict):
max_teacher = ""
max_count = 0
for teacher, courses in dict.items():
if len(courses) > max_count:
max_count = len(courses)
max_teacher = teacher
return max_teacher
i saw evreyone used .items() method even though Kenneth does not teach it so i check what this method does and it let us loop over a key,value pairs. however this solution passes but why? we check if the length of the current value is greater than max_count, but max_count is 0 what is the purpose of it?, i understand the solution but not the logic behind it, we assign max_teacher to the "key" that hold the most "values"
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(dict):
return len(dict)
def num_courses(dict):
total = 0
for x in dict.values():
total += len(x)
return total
def courses(dict):
my_list = []
for x in dict.values():
my_list.extend(x)
return my_list
def most_courses(dict):
max_teacher = ""
max_count = 0
for teacher, courses in dict.items():
if len(courses) > max_count:
max_count = len(courses)
max_teacher = teacher
return max_teacher
4 Answers

Tabatha Trahan
21,422 Pointsmax_count was declared and initialized to 0 so you could use it in your for loop. Because it is set to 0, the length of the first list you encounter should be greater than 0, so max_value will now point to this new value. You keep checking the length of the lists against max_value, which is the greatest number of courses so far. If you didn't set it to 0 initially, you wouldn't be able to compare it to anything in your for loop for the first list.
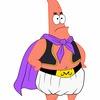
<noob />
17,062 PointsI understand this part now thanks, but one more question in this line: max_teacher = teacher i do not set the max_count to the key, how does "teacher" hold the key with the most values?

Tabatha Trahan
21,422 PointsIt's because you set teacher to the key each time through the for loop. You start out saying:
for teacher, courses in dict.items():
so for each time through the loop, teacher gets set to the key, and courses gets set to the corresponding list value. So if you look at the sample dictionary in the comments of the code, the first teacher would be Andrew Chalkley, and the corresponding value would be a list of his classes. The next iteration of the loop would have teacher point to the next key, which is Kenneth Love, and the courses variable would be pointing to Kenneth's list of courses.
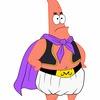
<noob />
17,062 PointsSo, your basically saying that after:
if len(courses) > max_count:
max_count = len(courses)
teacher will hold the greatest key with the greatest number of values because max_count will contain the number of the courses for the passed in key,value pair and the 1 of the keys will hold the highest number of values becuase max_count got assigned in his iteration?
I hope u understand

Tabatha Trahan
21,422 PointsYou got it. It's all about iterating through the dictionary and checking the length of the list assigned to the current value of courses, and then checking against max_count (which is looking at the greatest length so far). When max_count gets assigned a new length, the variable teacher is assigned the new value of the corresponding key. Then you have the teacher name (key) with the greatest number of courses (len(value)).
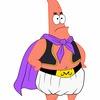
<noob />
17,062 PointsThanks Tabatha :]