Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial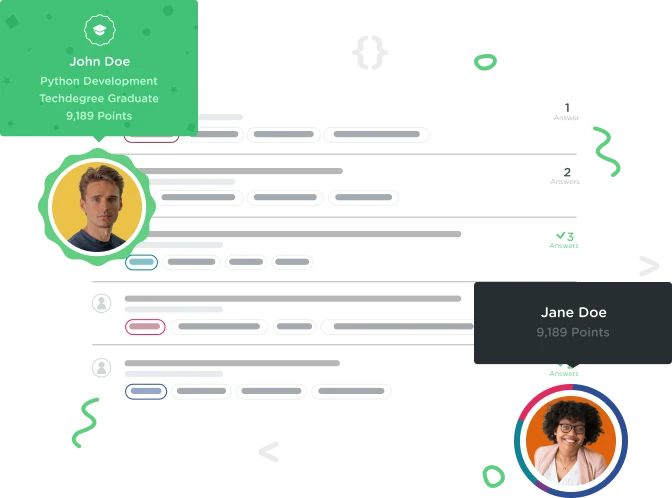
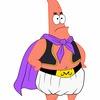
<noob />
17,062 PointsQuestions about lists and when to use them and more questions about other things.
Hi, So in this video craig added lists to our code. I have critical questions about lists that i dont know yet...
- in this code:
public List<String> getWordsPrefixedWith(String prefix)
{
List<String> results = new ArrayList<String>();
for(String word : getWords())
{
if(word.startsWith(prefix))
{
results.add(word);
}
}
return results;
}
i struggle to understand what exactly this line does :
List<String> results = new ArrayList<String>();
I understand that List is an interface and in order to to use list we need to call ArrayList am i right? but what excatly we are doing here?, as far as i understand we create a list of strings named results but i dont understand what new ArrayList<String>(); is doing, is it a convention that we must follow in order to create a list?
2) I saw in other question in the forum that ArrayList is a type of list that let us add and remove from the list, and Arrays.asList dont let us add and remove from the list. But i still dont understand why and when we should use Arrays.as List, in our case craig change the getWords() to return list instead of an array :
public List<String> getWords() {
String[] words = mDescription.toLowerCase().split("[^\\w#@']+");
return Arrays.asList(words);
}
so my question is :we use Arrays.asList in order to make an exising array a list?, because that is excatly what we are doing here.
Steven Parker ANTONY MUCHIRI Eric McKibbin Daniel Turato yanikapitanov
any insights regrading that matter will be appriciated...
2 Answers

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsHi Noob,
Thanks for the mention, please excuse my bad English.
List<String> results = new ArrayList<String>();
Teacher Craig used the above line as a standard way to define and initialize the results list. This way Craig is able to add items to results list. If you comment this line of code with "//" the compiler with show an error - "Cannot resolve symbol 'results' ". Show you need to first inform the compiler that "hey, look results is a list of type String" and this line does exactly that. Put differently you cannot use a list to add items to it if it doesn't exist or created already
You are right - List is an Interface but it cannot be instantiated directly ( i.e. you can't define it as List<String> myList = new List() ) This is where ArrayList class come in to implement the List Interface.
Now Arrays.asList() method of java.util.Arrays class acts as bridge between the standard Array and Java Collection Framework. Arrays.asList() method returns a fixed-size list meaning you cannot add or remove elements. This method is really useful when you wish to manually insert elements to a List and saves you time instead of calling .add() everytime you want to insert and element to a Collection List.
However it has a downside in that the array won't dynamic and cannot be increased/decreased in size . As an indication that the code is trying to modify a non-resizable or unmodifiable collection, UnsupportedOperationException is thrown if you try to add or remove elements.
To add/remove elements you need to wrap your list in a new ArrayList e.g.
List<String> myList = new ArrayList<>(Arrays.asList("me","them","him","her", "you"));
This new ArrayList is implemented as a resizable array and you can add, get, set and remove elements.
Cheers
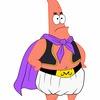