Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial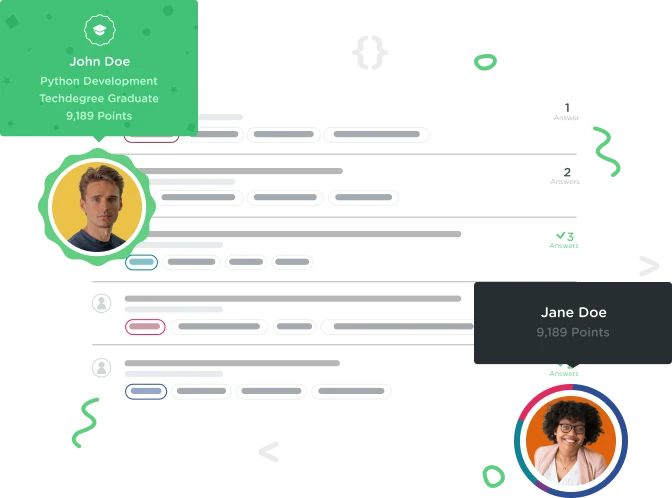

John Simoneau
Courses Plus Student 8,105 PointsQuestions about passing an argument to the function with Loop Conditions
I could be spacing out and not remembering something pointed out in an earlier video or I'm maybe missing it in this one somehow. But it seems like we just learned to pass arguments to a function AFTER the function. But in this video it seems to have jumped to storing the argument value in a variable before. So it's supposed to be
var upper = 2000;
var randomNumber = getRandomNumber(upper);
var guess;
var attempts = 0;
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
while ( guess !== randomNumber ) {
guess = getRandomNumber ( upper );
attempts += 1;
}
document.write("<p>The random number was: " + randomNumber + "</p>");
document.write("<p>It took the computer " + attempts + " attempts to get it right</p>");
But it seems in my head based off prior learnings in should be this
var randomNumber = getRandomNumber(upper);
var guess;
var attempts = 0;
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
getRandomNumber( 2000 );
while ( guess !== randomNumber ) {
guess = getRandomNumber ( upper );
attempts += 1;
}
document.write("<p>The random number was: " + randomNumber + "</p>");
document.write("<p>It took the computer " + attempts + " attempts to get it right</p>");
My way doesn't work of course but I'm just kinda curious why so I can wrap my head around this better.
Thanks
6 Answers
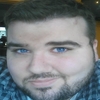
Marcus Parsons
15,719 PointsHey John,
There are only a couple reasons as to why your code doesn't work: 1) You didn't declare the variable upper
so it means randomNumber
will be undefined
. 2) When you are calling getRandomNumber
below the function declaration, you are not putting that result anywhere so the program has nowhere to put that information.
So, you could just combine the two and do that in the beginning:
var randomNumber = getRandomNumber(2000);
var guess;
var attempts = 0;
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
while ( guess !== randomNumber ) {
guess = getRandomNumber ( 2000 );
attempts += 1;
}
document.write("<p>The random number was: " + randomNumber + "</p>");
document.write("<p>It took the computer " + attempts + " attempts to get it right</p>");
It is very important to keep in mind that this means that you can't later manipulate what the upper limit is of the random number like you can if you set a variable for that value of 2000 (as in 1st example) instead of just statically placing that value there. It doesn't make for a dynamic experience to statically set a value like that.
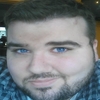
Marcus Parsons
15,719 PointsIn fact, a much better method (and keeps user experience in mind) is to allow the user to set a value they want like this:
var upper = parseInt(prompt("Give me a number! I'm hungry!"));
var randomNumber = getRandomNumber(upper);
var guess;
var attempts = 0;
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
while ( guess !== randomNumber ) {
guess = getRandomNumber ( upper );
attempts += 1;
}
document.write("<p>The random number was: " + randomNumber + "</p>");
document.write("<p>It took the computer " + attempts + " attempts to get it right</p>");

John Simoneau
Courses Plus Student 8,105 PointsHmm... I think I get it. It's just, in an earlier video he had us doing this
function goToCoffeeShop(drink) {
alert( drink + ' is on the way!' );
}
goToCoffeeShop("Iced Tea");
Which I didn't think declared "drink" but yet it seemed to work and was going off the instructors instructions. So I thought in this case you'd apply that same principal and it would work but it obviously didn't...LOL. But I don't recall him teaching any different since or really explaining that for us to know.
Ok, back to re-reading your post to see if I can wrap my head around it. I understand your codes and why they work. They make sense. I just can't 100% understand why mine didn't work based off the code in this post that we learned a few lessons ago.

John Simoneau
Courses Plus Student 8,105 PointsBut if I turn the tables and do the coffee shop argument the way the random number argument works... it doesn't work. Like so
var drink = "Iced Tea";
function goToCoffeeShop(drink) {
alert( drink + ' is on the way!' );
}
I guess I'm just not figuring out the difference of why it works to call the argument from a defined variable in some instances but not in others. Or why you can pass the argument for the parameter directly from calling the function in some instances but not others.
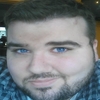
Marcus Parsons
15,719 PointsAnytime you are using a function, the variables that are declared in the function call only apply to that function. Things get really tricky whenever you declare variables outside of the function and have the same named ones inside a function call.
Precedence is always given to the same named variable inside a function call. What that means is that in your example:
var drink = "Iced Tea";
function goToCoffeeShop(drink) {
alert( drink + ' is on the way!' );
}
goToCoffeeShop();
//Added extra alert to show that drink is still defined
alert(drink + ' is on the way!');
drink
is undefined
in the function because the compiler looks at the variable defined by the function and uses that for the variable within the function. Since drink
was not defined when it was called, it is undefined
. So, the first alert says "undefined is on the way!".
The second alert is going to use the variable drink
defined outside of the function because you aren't calling any function for its value. So, the second alert will say "Iced Tea is on the way!".
Just remember that any time you declare a variable in a function call, it is only valid for that function! Its value cannot be used elsewhere in the code.
Does that help?
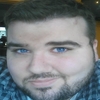
Marcus Parsons
15,719 PointsAnother example is this:
function showme(upper) {
var upper = 10;
alert(upper);
}
showme(20);
Which value do you think this function will show: 10 or 20? Well, it is going to show you 10 because you declared a new variable called upper within the function and that takes precedence over the called variable.
So, order of precedence for same named variables in regards to values of variables in functions goes like this (from the one used first to last): Variable declared inside function -> Variable in function call -> Variable declared outside function.
Now, taking a look at your code:
var randomNumber = getRandomNumber(upper);
You are calling this function, so that means the compiler is trying to put the value of a variable named upper
into the function getRandomNumber
but since there is no variable named upper
declared outside the function, it will pass in the value undefined
.

John Simoneau
Courses Plus Student 8,105 PointsFirst off, I REALLY appreciate your help! I know how frustrating explaining this over and over again must be but I am getting it. But I do have an odd-ball question which will likely make you roll your eyes...
Ok, based off everything said and going back to the first of the post. His teaching example:
var upper = 2000;
var randomNumber = getRandomNumber(upper);
var guess;
var attempts = 0;
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
while ( guess !== randomNumber ) {
guess = getRandomNumber ( upper );
attempts += 1;
}
document.write("<p>The random number was: " + randomNumber + "</p>");
document.write("<p>It took the computer " + attempts + " attempts to get it right</p>");
upper is defined in the var outside the function. What is the purpose of re-stating "upper" everywhere? Does it really need to be in these two places below?
var upper = 2000;
var randomNumber = getRandomNumber(upper);
var guess;
var attempts = 0;
function getRandomNumber(upper) { // HERE
return Math.floor( Math.random() * upper ) + 1;
}
while ( guess !== randomNumber ) {
guess = getRandomNumber ( upper ); // AND HERE
attempts += 1;
}
document.write("<p>The random number was: " + randomNumber + "</p>");
document.write("<p>It took the computer " + attempts + " attempts to get it right</p>");
When I remove those values upper values leaving just the ()'s everything seems to still work. I'm not sure what good they do since upper is being called in the getRandomNumber which seems to feed to the rest of everything.
If this is a complicated answer then don't worry about it. I'm sure I'll figure it out soon enough or re-learn it. But if it's not technically necessary but just keeps things tidy I would be interested to know.
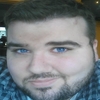
Marcus Parsons
15,719 PointsI love helping, for whatever reason, so it's all good, John! lol But, I see what you're getting confused by. In this example, you really could leave off those upper values because you're using the value from the variable. But those are two very different references.
This one:
function getRandomNumber(upper) { // HERE
return Math.floor( Math.random() * upper ) + 1;
}
uses whatever value you pass into it as a substitute for the word upper
and has nothing to do with the variable upper
. It makes the function more dynamic such that you could pass in any value you wanted instead of just that variable upper
.
This one:
guess = getRandomNumber ( upper ); // AND HERE
is passing in the value of the variable upper into the function getRandomNumber
.
If you really wanted to eliminate your confusion, call the variable something different in the function call like upperLimit
so that it will all now read:
var upper = 2000;
var randomNumber = getRandomNumber(upper);
var guess;
var attempts = 0;
function getRandomNumber(upperLimit) {
return Math.floor( Math.random() * upperLimit ) + 1;
}
while ( guess !== randomNumber ) {
guess = getRandomNumber ( upper ); // AND HERE
attempts += 1;
}
document.write("<p>The random number was: " + randomNumber + "</p>");
document.write("<p>It took the computer " + attempts + " attempts to get it right</p>");
You can avoid confusing yourself by just using different variable names, but you should do some practicing with this code to solidify the difference in those variables.

John Simoneau
Courses Plus Student 8,105 PointsOk, you said to practice so you asked for it...LOL. After playing around and reading your notes this is what I found. Which... if it's correct... has my mind straightened out...LOL. Though you say that the functions upper has nothing to do with the variable upper which is where it seems to get it's value from so that didn't make 100% sense. Either way... am I on the right path here to understanding things now or WAY off?
var computersMaxNumberForInitialLimit = 10;
var randomNumber = getRandomNumber(computersMaxNumberForInitialLimit);//gets value of 10 from the above var & sends the arguement to the getRandomNumber parameter for the function to use. Then stores the answer right back here in this var.
var computersGuessRange = 100000;//gets value of 10 from the above var & sends the arguement to the getRandomNumber parameter for the function to use. If I delete this var and change the guess = getRandomNumber to include this instead --> ( computersMaxNumberforInitialLimit) --> then it will keep the computers max guessing range to 10 as well which matches the computers initial range. Doing that saves from creating a useless secondary var with the value of 10 or having the computer guessing in an overly large range like it is now which is kinda unfair to it :p
var guess;
var attempts = 0;
function getRandomNumber(upperLimit) {
return Math.floor( Math.random() * upperLimit ) + 1;
}
while ( guess !== randomNumber ) {
guess = getRandomNumber ( computersGuessRange );//This is our guess. It currently sends the value of the computersGuessRange var to the to the getRandomNumber function and returns the response. If it's not the number being thought of (in the randomNumber var) it adds +1 below to the attempts property and tries again.
attempts += 1;
}
document.write("<p>The random number was: " + randomNumber + "</p>");
document.write("<p>It took the computer " + attempts + " attempts to get it right</p>");
At the end of this I think figuring out which post of yours above to reward 'best answer' too for my original question might be harder then figuring out Javascript...LOL. Too bad you can't select a series of posts as the best answer.
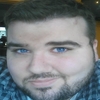
Marcus Parsons
15,719 PointsWhat you did with that above code is not what was being done in the original code, just so you're aware. Did you copy and paste this from somewhere because in your comment for the computersGuessRange
you mention that it is useless to create and use, which you are absolutely right. You probably notice that it now takes a factor of 100 times more tries for the computer to guess the number because the random number generated is from 0-10 but the guess variable can get a random number from 0-10,000! lol.
Just to reiterate the difference between these two again:
This upper
is a variable with a defined value:
var upper = 2000;
This upper
is merely a placeholder for values that are passed into the function. It has no correlation to the variable upper:
function getRandomNumber(upper) {
return Math.floor(Math.random() * upper) + 1;
}

John Simoneau
Courses Plus Student 8,105 PointsLOL..... No, I didn't copy/paste it from somewhere. You told me to do some practicing with this code to solidify the difference in the variables. So the code I pasted was my practice. It's the same code basically but I changed names and added that useless var intentionally. I was just dissecting each thing, breaking it down, and mapping it so I could understand it better. I wouldn't ever write a real code that way...LOL. But if you deleted that var and changed all the odd-ball things back to match the original code it would be the same as the original code.
The useless var I added so I could change the computers top range guessing number so that I knew which things were controlling what exactly. It was only being left in that code for me to show that I know what it is and does and how if I deleted that var and changed everything back to upper like the original code then everything would have the proper matching top range value. Basically I was doing what you did with changing two of them to be named upperLimit but I took it a step further so I could understand how to control the beast even a little bit more (though useless technically)...LOL.
Yes, I 100% understand that upper in the original code and the oddball things I named those in my code was just a placeholder. But in the original code there is 5 words with the name upper including the var which holds the key value we need for the guessing games top number for the range. I understand that the word upper in the function isn't asking the var upper for the value. It's just a placeholder and the value for that is being sent from elsewhere. But the var upper is the only place that holds the top range value that the function is using so somehow that value from the var upper is being sent to the function. All I was trying to do was find where the value from the var upper was being sent from to the function (which is channeled through the placeholder upper which is just a coincidental naming choice) because it has to be getting sent there somehow. Otherwise there wouldn't be an upper range number being used or controlled like it is.
My code was perhaps an insane funky way to map it but since it was for my own personal notes and exploring I didn't care. I tried to add comments in so you'd understand what I was doing but I guess it wasn't readable like it was in my head which is fine. The point is I think I fully understand the code now. In my mind I have no questions that I have it figured out and it's thanks to all your help, rewatching the videos over and over again, and testing each piece of the code a million ways.
If I'm actually correct might be a different story...LOL. But at least my brain feels it's correct about it at this point which is a step closer then it was when I started the thread...LOL.
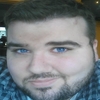
Marcus Parsons
15,719 PointsI apologize if my comment came off as rude as that was not my intent. I am glad that you are understanding everything better! Happy Coding! :)

John Simoneau
Courses Plus Student 8,105 PointsOh no! Not at all, your comment wasn't the slightest bit rude. I didn't take it that way at all and I wasn't giving a sarcastic response. You've been awesome and gone WAY out of your way to help me understand all this. I was just trying to re-explain what I did there because I know it didn't read like i was intending. But at the end let you know that no matter how poorly I'm wording things... I actually think I understand it finally thanks to your help.
I could be wrong in my understanding but I really don't think so now.
Thanks!
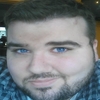
Marcus Parsons
15,719 PointsYou're very welcome, John, and I feel like you do have a much better grasp on the concepts presented here. Honestly, I don't even know ya, but I'm proud of ya! Heck yea! :) Good luck in your future endeavors!

John Simoneau
Courses Plus Student 8,105 PointsOuch, sorry about the long comments. I thought they would wrap and I don't see a markup code for that. Might need to copy/paste to a text editor for an easier read :(
John Simoneau
Courses Plus Student 8,105 PointsJohn Simoneau
Courses Plus Student 8,105 PointsOr I would have thought something like this would work but doesn't