Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial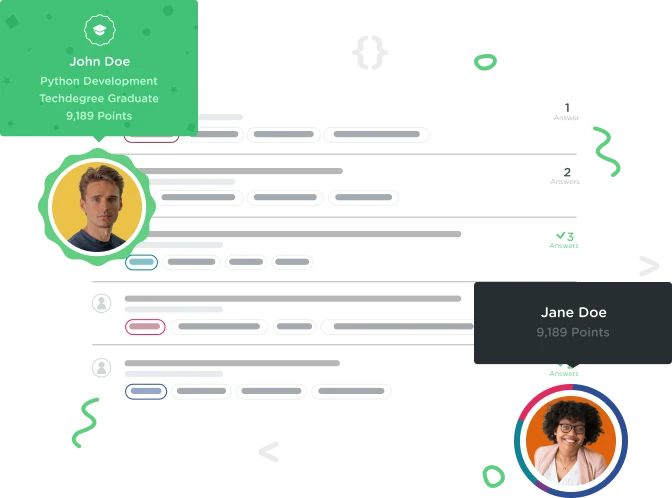
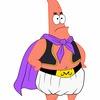
<noob />
17,062 Pointsquestions about the export method
Hello!
public void exportTo(String fileName)
{
try (
FileOutputStream fos = new FileOutputStream(fileName);
PrintWriter writer = new PrintWriter(fos);
) {
for(Song song : mSongs)
{
writer.printf("%s|%s|%s%n",
song.getArtist(),song.getTitle(),song.getVideoUrl());
}
} catch(IOException ioe)
{
System.out.printf("Problem saving %s %n",fileName);
ioe.printStackTrace();
}
}
in the export method we are making a new file?, and using the PrintWriter object to print the contents of the file to a new file? then we loop on each of the songs and write their artist name, song title and video url as displayed in the code? Anyone can explain to me what excatly those lines does?
FileOutputStream fos = new FileOutputStream(fileName);
PrintWriter writer = new PrintWriter(fos);
2 Answers
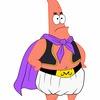
<noob />
17,062 Points
Courtney Wilson
8,031 PointsYes to all three questions except we aren't necessarily creating a new file but if the file doesn't already exist, then one will be created. In Karaoke.java, the importFrom() method is called first, so that is when the file is created. This is the code that creates the file (from the importFrom() method):
FileInputStream fis = new FileInputStream(fileName);
It's only created if the file is not found however, which is the case when we first run the Karaoke program.
This line of code:
FileOutputStream fos = new FileOutputStream(fileName);
is creating a new FileOutputStream object, which is an output stream that writes data (in the form of bytes) to a file. The name of the file it will write to is passed in as a String, fileName. Since the file has been created, it will open this file.
The second line:
PrintWriter writer = new PrintWriter(fos);
is creating a PrintWriter object, which allows us to print formatted data to the file using printf(). The constructor we use to create the PrintWriter is:
PrintWriter(OutputStream out)
As you can see, it takes an object of type OutputStream (or of any class that extends the OutputStream class) as a parameter. FileOutputStream extends OutputStream so we use the FileOutputStream variable we created, fos, and pass it in to the constructor. So now, we can use the printf() method to write our formatted string to the file.