Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial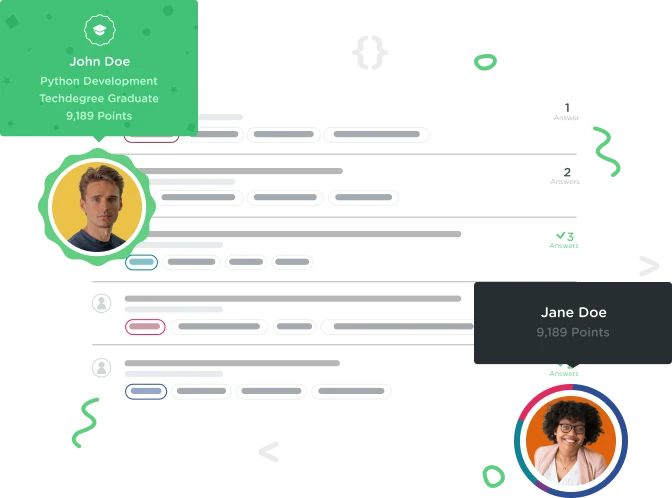
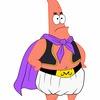
<noob />
17,062 PointsQuestions about this video.
Hello!, How exactly the toString() method works?, in earlier courses when we wanted for example to get the name of the autor we would call treet.getAuthor() and the same goes for all the other field members(description etc). I dont understand how the toString() method works, it somehow placed the autor and the description without even calling them, Someone can explain to me how exactly this works?
is it because : " Yeah, but it wasn't explained that apparently the printf() in the main() calls the toString() and that is how ("%s", treet) gets substituted with the overridden toString() method from the Treet class."
i came across this answer but i still dont fully understand how it is implemented, here is what i think and im not sure im correct, in our file the toString() takes the author,description and date from our treet instance?:
Treet treet = new Treet(
"craigdennis",
"java is fun and intersting" +
", i also like js",
new Date(1321849732000L)
);
and if we look here:
System.out.printf("This is a new Treet: %s %n", treet);
we have a placeholder(%s) and we fill it with treet, and treet is automatically filled somehow from the toString() method that takes is info from our treet instance?
Another important question i have is why and when we will want to use the keyword "Override" Craig intoduced in this video?, what exactly this keyword done?
any insights regarding to that matters will be very much appreciated!
3 Answers
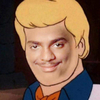
Daniel Turato
Java Web Development Techdegree Graduate 30,124 PointstoString() is a default method on each Object. By default, when you try to output the object it will call this default toString method and it will usually output the class name followed by it's hash code. This isn't really a useful output so you can Override the method so you can output your own information.
Overriding means that you take away any of the code that was in the method that a class has inherited and replace it with your own. And each class always inherits some default methods with one of them being toString().
An example of overridng a toString() would be like this :
@Override
public String toString() {
return ""
}
Now, usually in the toString method, you would output all your field members that are important and need to be seen for user. This is just an easy way to see the class from an outside perspective. But effectively , you can put whatever you want in the toString() method as you can see, it just needs to return a string of anything.
As a side note, you don't have to use the annotation @Override but it is good practice to do so as it helps optimize the java compiler (you don't have to worry about this). But regardless if you use the annotation @Override above the toString method, java will always call this method when you want to output an object.
Hope this helps, if you have any further questions then just comment them below.
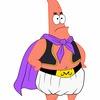
<noob />
17,062 PointsHi. so toString() method is a default value for all objects? when we create : Treet treet = new Treet() and passing our name and description , the toString method automatically print something to the console even if we didn’t specifically called an object? and in this video we did it because it is an easy way to output the information is our object instance treet?
what do u mean by saying “Overriding means that you take away any of the code that was in the method that a class has inherited and replace it with your own”? in your example u used the toString method to print “, does toString method overrides even simple printf command? i still don’t fully understand how the method knew to output our information from the treet instance. thanks for ur help
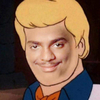
Daniel Turato
Java Web Development Techdegree Graduate 30,124 PointsCorrect, the toString() method is a default method on every Object. To see other default methods, look here -> https://docs.oracle.com/javase/7/docs/api/java/lang/Object.html
In your case, when you create a new Treet(), regardless if you override the toString() method, Treet will include one. The output from the toString() method will only show if you output the object or you output the toString() method. E.g :
Treet treet = new Treet();
// Both lines below will output the same string
System.out.println(treet);
System.out.println(treet.toString());
And again, you are correct when you say that you only overrided the toString() method in the video as it's an easy default way to output all the information of your Treet in one go.
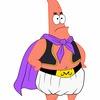
<noob />
17,062 Pointsthanks for u answer, so we will only get the method if we print it as u did , and it doesn’t matter we call the method or not because either way it’s a default method. now where and when i should use the override method and not specifically call a method?
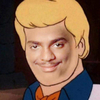
Daniel Turato
Java Web Development Techdegree Graduate 30,124 PointsI'm not sure I fully understand what you're asking but I'll try to explain it how I think you're seeing it.
You are correct, that you will only gain access to the toString() return string when you either print out the object or store/print the return value of the (class).toString().
It doesn't matter if we override it or not as each class will always have the toString() method . It's our choice if we want to override it and if we do, it is usually to output our class fields in a formatted way.
If you want to store the result of the toString() method, then you want to directly call it like this
Treet treet = new Treet();
String result = treet.toString();
But if you just want to output the result straight to the console, then do this
Treet treet = new Treet();
System.out.println(treet);