Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial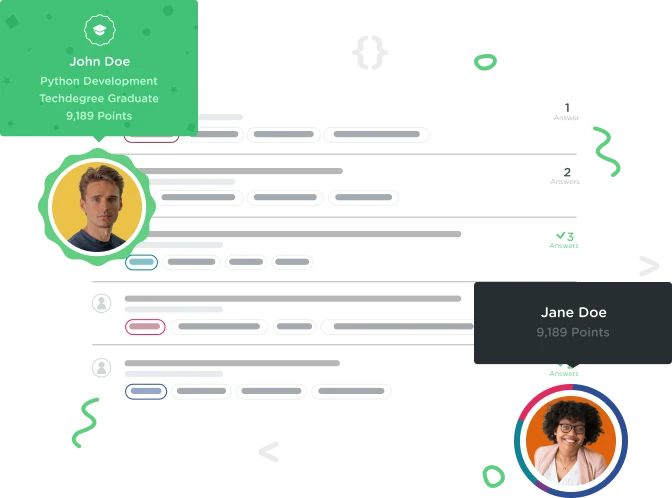

Patrick Rice
11,017 PointsQuestions on a couple parts of the code
Please see my questions in the code comments...thanks!
var message = '';
var student;
var search; // why do we put some variables up here but not others? (such as report)
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Please enter a student name: "); // why do we not use var here like we did in getStudentReport?
if (search === null || search.toLowerCase() === 'quit') {
break;
} // why do we not use else here? can else be implied if left blank in JavaScript?
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name === search ) {
message = getStudentReport ( student );
print(message);
}
}
}
2 Answers
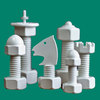
Steven Parker
229,732 PointsI'll answer your questions individually:
- why do we put some variables up here but not others? (such as report)
Variables that are used only inside a function are declared there to limit their scope. Those variables cannot be accessed from outside the function, and will not interfere with global variables or functions, even if they share the same name. This is a coding "best practice".
- why do we not use var here like we did in getStudentReport?
A variable should only be declared once, but it can be assigned any number of times. Since "search" was already declared in the global scope (on line 3), it is only being assigned here.
- why do we not use else here? can else be implied if left blank in JavaScript?
No "else" is needed at this point because the "break" when the condition is true diverts the program flow to after the loop. So in essence, everything inside the loop following the "if" block is already in the "else" situation since it will only run if the condition was not true.
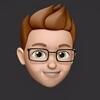
Brandon VanCamp
Front End Web Development Techdegree Graduate 30,954 PointsHello Patrick Rice,
To work with JavaScript efficiently, one of the first things we need to understand is the concept of variable scope. The scope of a variable is controlled by the location of the variable declaration, and defines the part of the program where a particular variable is accessible.
i added comments to go along with yours as followed..
<script>
var message = '';
var student;
var search; // why do we put some variables up here but not others? (such as report)
// This (search) variable is declared in global scope. Therefore accessible from anywhere in your code.
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// (report) Since declared within that function is only accessible from that function and any nested functions.
function getStudentReport( student ) {
// this (report) variable is declared in local scope. only accessed in this function.
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Please enter a student name: "); // why do we not use var here like we did in getStudentReport?
// We are starting a while loop here, so we can loop threw the prompt method. until it is we say quit.
if (search === null || search.toLowerCase() === 'quit') { // when we say quit
break;// stop the loop if the previous statement is true.
} // why do we not use else here? can else be implied if left blank in JavaScript?
// we want to loop threw all the students entered in the search variable. and print the information we entered in the prompt.
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name === search ) {
message = getStudentReport ( student );
print(message);
}
}
}
</script>
Let me know if that helps or not!

Patrick Rice
11,017 PointsHelpful, thanks for you input
Patrick Rice
11,017 PointsPatrick Rice
11,017 PointsThanks - this is helpful.