Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial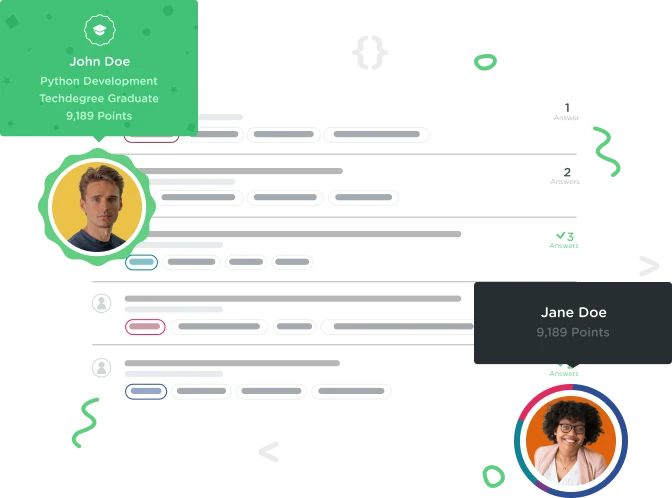

Brian Maimone
Courses Plus Student 1,644 PointsQuestions on Karaoke byArtist method. Would appreciate feedback.
I've used //, ?? on code lines where I have the questions.
The code:
package com.teamtreehouse.model;
import java.util.List;
import java.util.ArrayList;
import java.util.Set;
import java.util.Map;
import java.util.HashMap;
public class SongBook {
private List<Song> mSongs;//?? // dont have initialize Song bc in same package
public SongBook() { //constructor takes nothing
mSongs = new ArrayList<Song>();//initializing list of songs,
// dont have initialize Song bc in same package
}
public void addSong(Song song) {//pass in a song
mSongs.add(song);
}
public int getSongCount() {
return mSongs.size();
}
//FIXME: This should be cached!
private Map<String, List<Song>> byArtist() {// method creating Map of artists to songs
Map<String, List<Song>> byArtist = new HashMap<>();// How, where does the Song object with 3 string variables, artist, title, video url get stored into a list data structure?
for (Song song : mSongs) { // for each song currently in mSongs list
List<Song> artistSongs = byArtist.get(song.getArtist());// How can we get the list from the key value out of byArtist map when we haven't fully declared artistSongs as a new ArrayList, which we then do 2 lines down?
if (artistSongs == null) {// see if yet to find an artist, if not make new one
artistSongs = new ArrayList<>();//
byArtist.put(song.getArtist(), artistSongs);//put into map the artist name and new list we made but we have artistSongs ArrayList evaluating to null at this point??
}
artistSongs.add(song);// how does List add method put an objects' string variables into a comma separated list sequence?
}
return byArtist;
}
public Set<String> getArtists() {
return byArtist().keySet();
}
public List<Song> getSongsForArtist(String artistName) {
return byArtist().get(artistName);
}
}
package com.teamtreehouse.model;
public class Song {
private String mArtist;
private String mTitle;
private String mVideoUrl;
public Song(String artist, String title, String videoUrl){
mArtist = artist;
mTitle = title;
mVideoUrl = videoUrl;
}
public String getTitle() {
return mTitle;
}
public String getArtist() {
return mArtist;
}
public String getVideoUrl() {
return mVideoUrl;
}
@Override
public String toString() {
return String.format("Song: %s by %s", mTitle, mArtist);
}
}
2 Answers

Craig Dennis
Treehouse TeacherOn the second line of the method where we create the new Map: How, where does the public Song object/constructor with 3 string parameters, artist, title, video url get stored into the list data structure?
The addSong
adds songs to the mSongs list. That line is just creating a new HashMap
.
On the fourth line of method: How can we create the list using a key element (out of byArtist map) when we haven't declared artistSongs as a new ArrayList yet, we do it 2 lines down?
Remember that we are just stating that what comes out of the Map
is a List
. Since the first time through, it will return null
because that is how get works. It returns null
if it doesn't exist. We use that nugget of information in the if
statement. Remember this is happening in a for-each
loop, so it is going to happen numerous times, if it exists, a list is returned, otherwise, a null
is returned.
On seventh line: We are putting artist name into map and new list we made but we have artistSongs ArrayList evaluating to null at this point??
We are making a new list if it doesn't exist here:
if (artistSongs == null) {// see if yet to find an artist, if not make new one
artistSongs = new ArrayList<>();//
Your comment looks like you understood that. But see how artistSongs is being initialized there?
On eigth line: Worded differently but same as first question. Is way to visualize how a List add function puts an objects' string parameters into a comma separated sequence?
Hmmmm... not sure exactly what you are asking. The variable song
there is an instance of the class Song
, which was constructed and then added to the mSongs
List
of the KaraokeMachine
. The KaraokeMachine.importFrom
takes the comma separated list of songs and adds those Song
objects.
That make more sense?

Brian Maimone
Courses Plus Student 1,644 PointsAll questions are on the byArtist method. On the second line of the method where we create the new Map: How, where does the public Song object/constructor with 3 string parameters, artist, title, video url get stored into the list data structure?
On the fourth line of method: How can we create the list using a key element (out of byArtist map) when we haven't declared artistSongs as a new ArrayList yet, we do it 2 lines down?
On seventh line: We are putting artist name into map and new list we made but we have artistSongs ArrayList evaluating to null at this point??
On eigth line: Worded differently but same as first question. Is way to visualize how a List add function puts an objects' string parameters into a comma separated sequence?
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherHi Brian!
I wrapped your code in 3 backticks and the name of the file to make things pretty.
Can you help me figure out your specific questions? I'm assuming the lines that start with How?
We can go one at a time in here.