Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial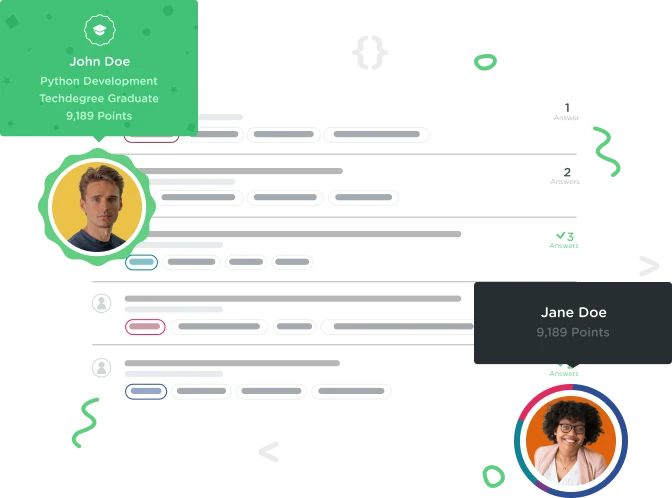

Enyang Mercy
Courses Plus Student 2,339 PointsQuickFix Challenge 1
Help with the codes please Creat a new video add the newly created video to course videos
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.TreeMap;
import java.util.List;
import java.util.Arrays;
import java.util.Map;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
// TODO(2): Add the newly created video to the course videos as the second video.
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
}
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> byTitle = new TreeMap<String, Video>();
ArrayList<Video> courseList = new ArrayList<Video>();
for (Video video : course.getVideos()) {
byTitle.put(video.getTitle(), video);
}
return byTitle;
}
}
2 Answers
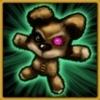
Dave Harker
Courses Plus Student 15,510 PointsHi Enyang,
Considering the Task 1 parts are not filled in ... I'll assume it is that Task you want help with :)
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
// Instantiate a new Video object (I called it newVideo) and pass required name into the constructor
Video newVideo = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
// As the course has been passed in, we call the getVideos() method from that object to get the list
// Now we have the video list we add the newVideo object to it in position 1 (2nd video as lists use a 0 based index)
course.getVideos().add(1, newVideo);
}
And you're set. Hope I explained that OK :) Happy coding.
Dave.

Enyang Mercy
Courses Plus Student 2,339 PointsAwesome !!!! Thank you