Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial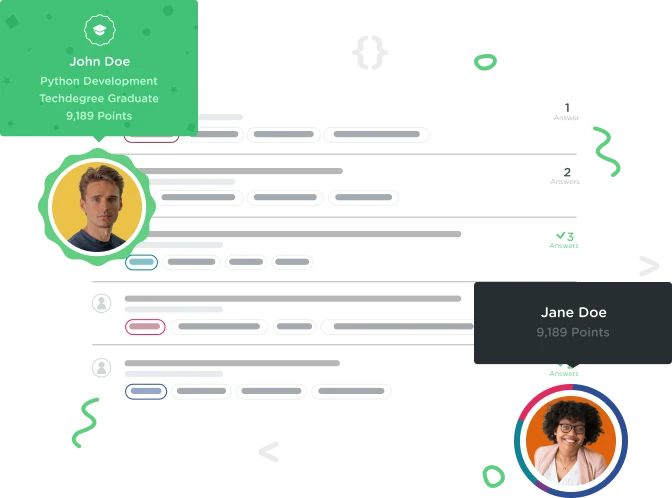

shaunsmith
12,145 PointsQuickFix.java Adding to LinkList?
I looked an a similar answer, but I'm not sure how I to add the title to a non-local LinkList?
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
import java.util.LinkedList;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
// TODO(2): Add the newly created video to the course videos as the second video.
Video video = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
LinkedList<Video> vid = new LinkedList<>();
vid.addAll(course.getVideos());
vid.add(video);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
}
public Map<String, Video> videosByTitle(Course course) {
return null;
}
}
2 Answers

shaunsmith
12,145 PointsI was close. Thank you!
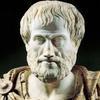
Florian Tönjes
Full Stack JavaScript Techdegree Graduate 50,856 PointsHey Shaun,
you can get a reference to the video list of the course object using its 'getVideos' getter method. Then you can simply add another video to it using the 'add' method.
[...]
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video video = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
course.getVideos().add(1, video);
[...]
}
Regards, Florian