Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial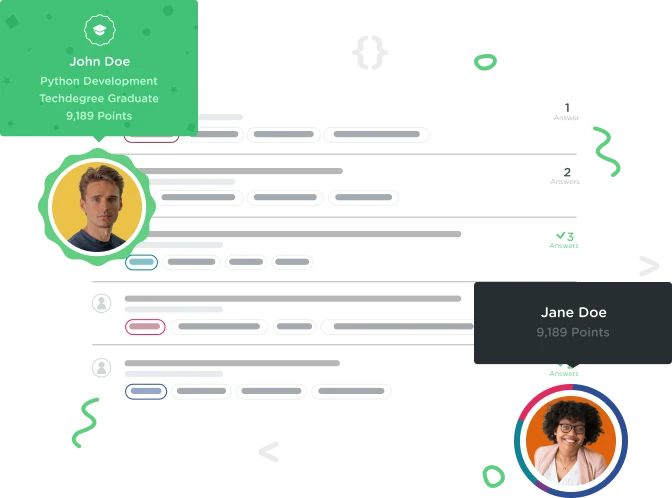

Sim L.
3,106 PointsQuiz: always prints out 0/10 even when answers are correct [Solved]
I hope I did the format right for posting on the forums. So I looked through the code and everything seems correct except for when it comes to the end of the quiz where it prints out how many answers are correct.
import datetime
import random
from questions import Add, Multiply
class Quiz:
questions = [] # list to hold questions
answers = [] # list to hold answers
def __init__(self):
question_types = (Add, Multiply) # brings in the types of questions
# generate 10 random questions with numbers from 1 to 10
for _ in range(10): # '_' is used to tell python to throw in whatever number, 1,5,7, w/e until it reaches 10.
num1 = random.randint(1, 10)
num2 = random.randint(1, 10)
question = random.choice(question_types)(num1, num2)
self.questions.append(question) # add these questions into self.questions
def take_quiz(self):
# log the start time
self.start_time = datetime.datetime.now()
# ask all of the questions
for question in self.questions: # loops through each question in the questions list
# log if they got the question right
self.answers.append(self.ask(question))
else:
# log end time
self.end_time = datetime.datetime.now()
# show a summary
return self.summary()
def ask(self, question):
correct = False
# log start time
questions_start = datetime.datetime.now()
# capture the answer
answer = input(question.text + " = ")
# check the answer
if answer == str(question.answer):
correct = True
# log the end time
questions_end = datetime.datetime.now()
# if the answer's right, send back True
# otherwise send back False
# send back elapsed time too
return correct, questions_end - questions_start
def total_correct(self):
# return total # of answers correct
total = 0
for answer in self.answers:
if answer[0]:
total += 1
return total
def summary(self):
# print how many you got right and the total # of questions. like 5/10
# if answer is correct:
print("Answers correct: {}/{}".format(
self.total_correct(), len(self.questions)
))
# print total time for the question: like 30 seconds
print("This quiz took you {} seconds total.".format(
(self.end_time-self.start_time).seconds
))
Quiz().take_quiz()
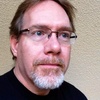
Chris Freeman
Treehouse Moderator 68,423 PointsGreat! I've changed title to [Solved]
4 Answers
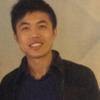
Eddie Tsai
18,473 PointsTry this below:
if int(answer) == question.answer:
correct = True
From the python 2.7 documentation, it says: Input is equivalent to eval(raw_input(prompt)). The expression argument is parsed and evaluated as a Python expression
I think this where the problem occurs.
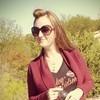
Mittsy Tidwell
11,420 PointsI'm having the same issue with mine. Do you know what from future you imported? I have tried all of the ones that I can find and none of them are working for me. When I copy and paste from my 2.7 file over to workspace it works perfectly. Thank you in advance!
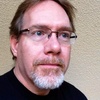
Chris Freeman
Treehouse Moderator 68,423 PointsHave you tried:
from __future__ import print_function
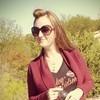
Mittsy Tidwell
11,420 PointsYes, I have tried this but it doesn't seem to be working for me. Maybe there is something small that I am overlooking. Here is my code:
from __future__ import print_function
import random
import datetime
from questions import Add, Multiply
class Quiz(object):
questions = []
answers = []
def __init__(self):
question_types = (Add, Multiply)
for _ in range(10):
num1 = random.randint(1, 10)
num2 = random.randint(1, 10)
question = random.choice(question_types)(num1, num2)
self.questions.append(question)
def take_quiz(self):
self.start_time = datetime.datetime.now()
for question in self.questions:
self.answers.append(self.ask(question))
else:
self.end_time = datetime.datetime.now()
return self.summary()
def ask(self, question):
correct = False
questions_start = datetime.datetime.now()
answer = input(question.text + ' = ')
if answer == str(question.answer):
correct = True
questions_end = datetime.datetime.now()
return correct, questions_end - questions_start
def total_correct(self):
total = 0
for answer in self.answers:
if answer[0]:
total += 1
return total
return total
def summary(self):
print('You got {} answers correct out of {}.'.format(self.total_correct(), len(self.questions)))
print('That took {} seconds'.format((self.end_time - self.start_time).seconds))
Quiz().take_quiz()
[MOD: added ```python formatting -cf]
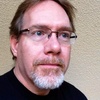
Chris Freeman
Treehouse Moderator 68,423 PointsCan you post the error you're seeing?
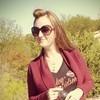
Mittsy Tidwell
11,420 PointsI'm not actually seeing an error. Everything seems to be working fine it just will not calculate the number of correct answers for me in python 2.7, but when I put it in python 3 it does it perfectly. This is what it looks like in the terminal when I run it:
C:\Users\mittsy\Google Drive\PROGRAMMING\PYTHON\TreeHouse\Dates_and_Times>python quiz.py
4 * 1 = 4
4 + 6 = 10
8 * 7 = 22
10 + 4 = 14
3 * 9 = 27
8 + 9 = 17
4 * 8 = 32
4 * 7 = 42
1 * 3 = 3
4 * 1 = 4
You got 0 answers correct out of 10.
That took 23 seconds
C:\Users\mittsy\Google Drive\PROGRAMMING\PYTHON\TreeHouse\Dates_and_Times>
[MOD: added ```bash formatting -cf]

Abhishek Kulkarni
4,405 PointsI am having the same issue. I am using python 3.7 though. Can't figure it out?Chris Freeman ?
Sim L.
3,106 PointsSim L.
3,106 PointsNevermind I figured out my problem. Python workspace was running in version 2 instead of version 3. Didn't notice until I started the next course.