Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial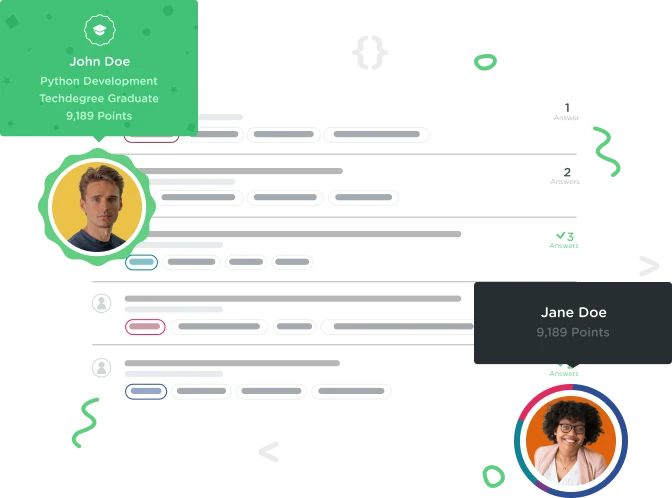

Elena Paraschiv
9,938 Pointsquiz arrays
<?php
$movies[] = array(
"title" => "Back to the Future",
"year" => 1985
);
$movies[] = array(
"title" => "The Empire Strikes Back",
"year" => 1980
);
foreach($movies as $movie) {
echo "-" . $movie["title"] . "-";
}
?>
What will this block of PHP code display?
I picked - The Empire Strikes back because I thought only the second array is executed. Can someone explain why both of the titles are displayed. Thank you kindly
2 Answers

Tim Vasnelis
9,499 Points$movies[] = array(
"title" => "Back to the Future",
"year" => 1985
);
$films[] = array(
"title" => "The Empire Strikes Back",
"year" => 1980
);
In that case you would have two separate and unique arrays, $movies and $films.
foreach($movies as $movie) {
echo "-" . $movie["title"] . "-";
}
would output "-Back to the Future-"
foreach($films as $film) {
echo "-" . $film["title"] . "-";
}
would output "-The Empire Strikes Back-"
You could overwrite the value in movies by:
$movies[0] = array(
"title" => "The Empire Strikes Back",
"year" => 1980
);

Tim Vasnelis
9,499 PointsThere is only one array, $movies. The first bit of code:
$movies[] = array(
"title" => "Back to the Future",
"year" => 1983
);
adds Back to the Future to the end of the $movies array. Since the array is empty, it is added it at index 0 by default.
the next bit of code
$movies[] = array(
"title" => "The Empire Strikes Back",
"year" => 1980
);
adds The Empire Strikes Back to the end of the $movies array. The index is automatically incremented to 1. This does not overwrite the first element.
There is no second array. So, when you loop through the array with the foreach loop, both values are displayed with the "-" delimiter.

Elena Paraschiv
9,938 PointsOk. So as I understand because $movies is repeated it means it is the same array and the index is incremented with the second title value. But if the first array would be $movies and the second array $films, then $films would overwrite $movies. Did I get it right?
Elena Paraschiv
9,938 PointsElena Paraschiv
9,938 PointsI got it. Thank you Timothy. You rock!