Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial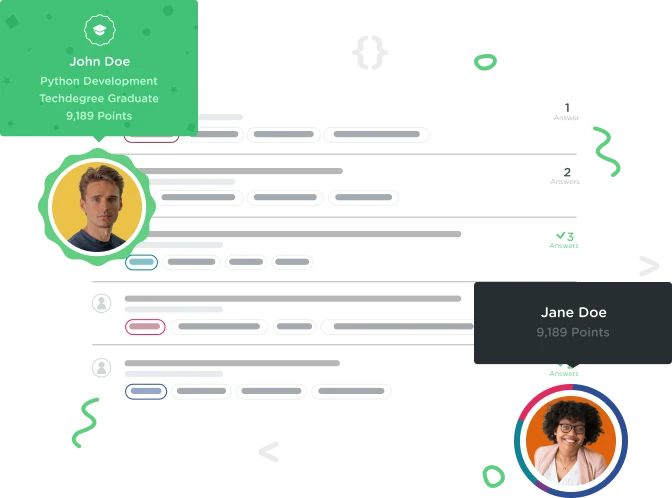
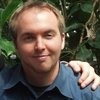
Eric Conklin
8,350 PointsQuiz challenge from arrays javascript section
/*Variables*/
var questionAnswer = [
['Who is the president?', 'Barack Obama'],
['What is the 3rd month of the year?', 'March'],
['What color is the sky?', 'Blue']
]
var questionsRight;
var questionsRightList;
var questionsWrong;
var questionsWrongList;
var answer1 = prompt(questionAnswer[0][0]);
var answer2 = prompt(questionAnswer[1][0]);
var answer3 = prompt(questionAnswer[2][0]);
var answer1Right;
var answer2Right;
var answer3Right;
var answer1Wrong;
var answer2Wrong;
var answer3Wrong;
/* If else loops for answers */
if (answer1 = questionAnswer[0][1]){
var questionsRight += 1;
var answer1Right = questionAnswer[0][0];
} else {
var questionsWrong += 1;
var answer1Wrong = questionAnswer[0][0];
}
if (answer2 = questionAnswer[1][1]){
var questionsRight +=1;
var answer2Right = questionAnswer[1][0];
} else {
var questionsWrong += 1;
var answer2Wrong = questionAnswer[1][0];
}
if (answer3 = questionAnswer[2][1]){
var questionsRight +=1;
var answer3Right = questionAnswer[2][0];
} else {
var questionsWrong += 1;
var answer3Wrong = questionAnswer[2][0];
}
var questionsRightList = [answer1Right, answer2Right, answer3Right];
var questionsWrongList = [answer1Wrong, answer2Wrong, answer3Wrong];
Unexpected token at line 25. What is wrong with the += operator the way I'm using it? Help...
3 Answers

elk6
22,916 PointsHi Eric,
You are declaring your var over and over. You only need to use the var keyword for the first time you delcare it. When changing the value you only need to write it's name followed by += ( in this case ).
Also, if you put your code inside 3 backticks ( ) your code will be a lot easier to follow on the forum. So 3 times
in front and 3 times ` at the back of your code.
Hope this helps.
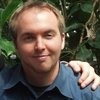
Eric Conklin
8,350 PointsElian, it was my understanding that in order to access a global variable, I had to redeclare it within the for loop. Is this incorrect?
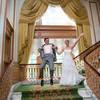
Colin Bell
29,679 Pointsit was my understanding that in order to access a global variable, I had to redeclare it within the for loop. Is this incorrect?
Nope. A global variable is accessible anywhere. So you can declare it once in the global scope, then use it in any loop, function, array, etc.
You're also doing a lot of work that a for
loop can do for you. You don't need to declare a variable for each separate question in the global scope. Plus, if you were to add more questions to your array, you'd have to keep adding variables for questionsRight, questionsWrong, etc.
The global variables you need to declare are :
- The
questions
variable with your questions and answers - Setting your initial
questionsRight
andquestionsWrong
variables to 0. - Setting
questiongsRightList
&questiongsWrongList
to empty arrays.
var questions = [
['Who is the president?', 'Barack Obama'],
['What is the 3rd month of the year?', 'March'],
['What color is the sky?', 'Blue']
];
var questionsRight = 0;
var questionsWrong = 0;
var questionsRightList = [];
var questionsWrongList = [];
/* Don't NEED to declare the following right away, but it's good practice to go ahead
and declare the variables you're going to use. Notice you don't need to assign any
default value to them */
var question;
var answer;
var response;
var html;
function print (message) {
document.write(message);
}
for (var i=0; i < questions.length; i++) {
// Get the first item from array[i] and set it as question
question = questions[i][0];
// Get the second item from array[i] and set it as answer
answer = questions[i][1];
// Get input from the user and set it to the response variable
response = prompt(question);
if (response === answer) {
//questionsRight is initially 0. If response === answer questionsRight = 0+1
questionsRight += 1;
// Push the question into the questionsRightList array if answered correctly.
questionsRightList.push(question);
}
else {
//questionsWrong is initially 0. If response === answer questionsWrong = 0+1
questionsWrong += 1;
// Push the question into the questionsRightList array if answered correctly.
questionsWrongList.push(question);
}
}
print(questionsRight + " " + questionsRightList + "; " + questionsWrong + " " + questionsWrongList );
Colin Bell
29,679 PointsColin Bell
29,679 PointsI made the code a little easier to read.