Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial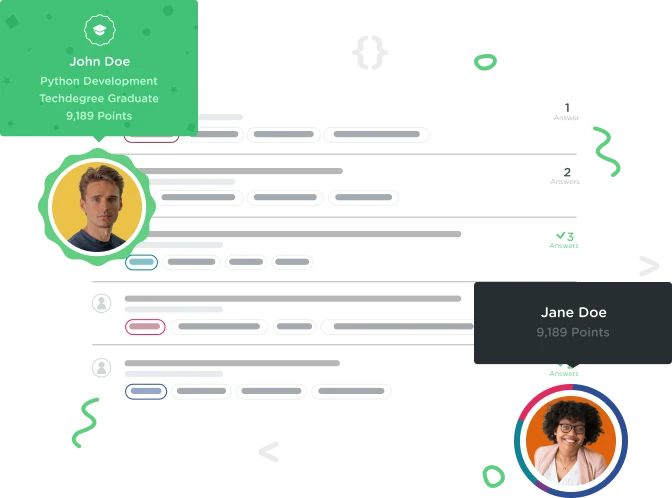

Stanislav Viner
210 Pointsquotes or no quotes inside two brackets?
Should property names/keys inside square brackets be in quotes - if the name is a string?
Example:
object[foo] = 'value';
OR
object['foo'] = 'value';
?
6 Answers

Markus Ylisiurunen
15,034 PointsHi!
If you use the bracket notation then you should use quotes. Here's a little example for you.
var object = {
'one': 1,
'two': 2
};
// you should do this
object['one'];
// and not this
object[one];
// but you could also do this
object.one;
Hope it helps!

Markus Ylisiurunen
15,034 PointsNo problem!
It would still be the same. So you have to use quotes with the bracket notation no matter what. Let me write another example to clear things up.
var object = {
myFriends: 10,
'my friends': 10
};
// the quotes only matter when the property name isn't a valid JavaScript variable name
object.myFriends;
object['myFriends'];
object['my friends'];
// you can't do this
object.my friends;
As you can see in the previous example the quotes around object's property names matter only if the property name is not a valid JavaScript variable name.
Hopefully this clears things up a little!

Stanislav Viner
210 PointsThis definitely clears things up! One of the quiz questions in this Object-Oriented JS tutorial threw me off. It asked which notation of Object/property was incorrect. I chose the one that seemed to me to be incorrect, but the system said that I got it wrong - but now that you've explained it, I see that I was right - it was var key = "name"; cat[key] ....that's the way the option was written, without quotes around the key property in the square brackets. But the system treated this as a correct notation?!
Anyway, thank you again!

Markus Ylisiurunen
15,034 PointsI get why you got confused now that you told the question. The way the system did it is actually a correct way too. The property of the object is not key
but it's actually name
. When you write key
inside the brackets without the quotes you actually reference to a variable. Let's write another example.
var key = 'name';
var object = {
name: 'John'
};
// now when you do this
object[key];
// it's exactly the same thing as doing this
object['name'];
This happens because key
is a variable which has a string name
. So basically you can think of it so that the variable key
gets replaced with the string name
. Sorry I might just be confusing you more.

Stanislav Viner
210 PointsSo in var key = 'name' is "name" a property because var key doesn't have a visible property like var object, so whatever var key is equal to, that value would automatically be its property?
i.e. would these two examples be interchangeable?
object[property] and var object = "property"

Markus Ylisiurunen
15,034 PointsI didn't quite get what you're trying to ask. We have two variables, key
and object
. Even though a string is technically an object too in Javascript, we can think of it just as a piece of text. In the previous example I just simply used the value of the variable key
as the property name of the object
to retrieve its value.
// this retrieves the property of the object
object['property'];
// this assigns a string to a variable called object
var object = 'property';
Btw check out the Markdown Cheatsheet to see how you can format code in these posts. It's a lot easier to see when it's properly formatted.

Stanislav Viner
210 PointsThanks, Markus!
Stanislav Viner
210 PointsStanislav Viner
210 PointsThanks, Markus!
Just one follow up question:
For this example...
var object = { 'one': 1, 'two': 2 };
What if one and two were without quotes ....would it still be object['one'] or would it be object[one] ?