Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial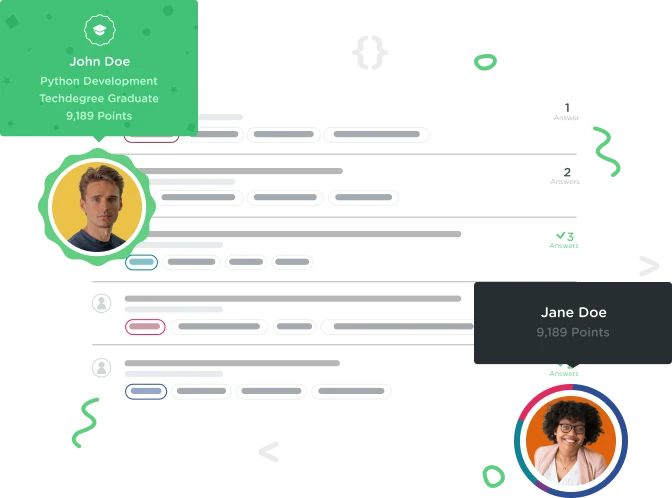
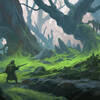
Caleb Newell
12,562 Pointsrace car
Vrroom!
OK, now let's add a method named run_lap. It'll take a length argument. It should reduce the fuel_remaining attribute by length multiplied by 0.125.
Oh, and add a laps attribute to the class, set to 0, and increment it each time the run_lap method is called.
class RaceCar():
def __init__(self, color, fuel_remaining, laps=0, **kwargs):
self.color = color
self.laps = laps
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length, **kwargs):
self.fuel_remaining = (fuel_remaining - 0.125) * length
laps = laps + 1
4 Answers

Unsubscribed User
8,947 PointsPerhaps my previous answer was a bit confusing. My second paragraph was meant as an example, not as something that should be part of an if statement in your code. Let's go back to the code in your initial question. The only line you need to change is:
self.fuel_remaining = (fuel_remaining - 0.125) * length
And let's focus on what is to the right of the equal to sign:
(fuel_remaining - 0.125) * length
Now, if we assume that you have 1 (litre) of fuel. We know that 0.125 litre of fuel is used each lap. So two laps should use 0.25 litres of fuel. Thus, after two laps you would have 1 - 0.25 = 0.75. Now, if we use this in your code:
self.fuel_remaining = (fuel_remaining - 0.125) * length
self.fuel_remaining = (1 - 0.125) * 2
self.fuel_remaining = 0.75 *2
self.fuel_remaining = 1.5
But that can't be right. You have more fuel than when you started!
However (and the right answer):
self.fuel_remaining = fuel_remaining - (0.125 * length)
self.fuel_remaining = 1 - (0.125 * 2)
self.fuel_remaining = 1 - 0.25
self.fuel_remaining = 0.75

Unsubscribed User
8,947 Points(fuel_remaining - 0.125) * length
If fuel_remaining = 1 and length = 2, you will have more fuel remaining after two laps than before you ran the laps. Consider changing the above equation.
You need to multiply length with 0.125 and subtract the result from fuel_remaining.
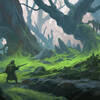
Caleb Newell
12,562 Pointsevery thing else in my code still works, but i still do not understand the equation. A little more help would be thanked!
(by the way, does this look right?)
class RaceCar():
def __init__(self, color, fuel_remaining, laps=0, **kwargs):
self.color = color
self.laps = laps
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length, fuel_remaining, **kwargs):
if fuel_remaining == 1 and length == 2():
length * 0.125 - fuel_remaining

Unsubscribed User
8,947 PointsAs I said, go back to your initial code, and change the run_lap method by moving the parenthesis:
def run_lap(self, length, **kwargs):
self.fuel_remaining = fuel_remaining - (0.125 * length)
laps = laps + 1
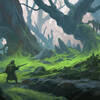
Caleb Newell
12,562 PointsYep. I got it. Thanks for taking the time out of your schedule and helping me!

Unsubscribed User
8,947 PointsNo problem! If you don't already, I recommend testing the challenges (where possible) in an IDE such as MS Visual Studio Code or Atom. Being able to test different arguments helps a lot!

Kyle Bateman
3,642 PointsI seem to have the exact same code as what is shown in this community board, but I cannot get it to work. Can anyone help me?
class RaceCar:
def __init__(self, color, fuel_remaining, laps=0, **kwargs):
self.color = color
self.laps = laps
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length, **kwargs):
self.fuel_remaining = fuel_remaining - (0.125 * length)
laps = laps + 1

Kyle Bateman
3,642 PointsI figured it out...the last line was incorrect. Should have been:
self.laps = laps + 1
or
self.laps += 1
Caleb Newell
12,562 PointsCaleb Newell
12,562 PointsThank you for explaining this.