Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial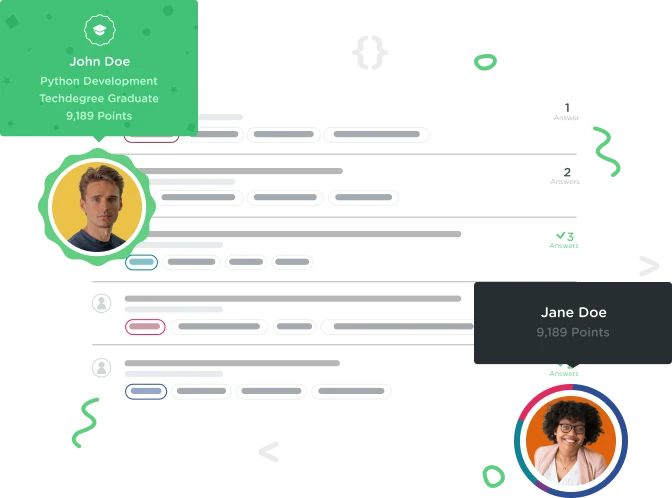

Pankaj Gupta
3,469 Pointsracecar.py - need help
In Python, attributes defined on the class, but not an instance, are universal. So if you change the value of the attribute, any instance that doesn't have it set explicitly will have its value changed, too!
For example, right now, if we made a RaceCar instance named red_car, then did RaceCar.laps = 10, red_car.laps would be 10!
To prevent this, be sure to set the laps attribute inside of your init method (it doesn't have to be a keyword argument, though). If you already did it, just hit that "run" button and you're good to go!
class RaceCar:
laps = 0
def __init__(self, color, fuel_remaining, laps, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
self.laps = laps
def run_lap(self, length):
self.laps += 1
self.fuel_remaining -= length * 0.125
9 Answers

Omid Ashtari
4,813 Pointsstuck in the same place, help please :)
class RaceCar:
def __init__(self,color,fuel_remaining, **kwargs)
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = 0
for (key,value) in kwargs.items():
setattr(self,key,value)
def run_lap(self,length):
self.fuel_remaining -= length*0.125
self.laps += 1

Omid Ashtari
4,813 PointsARGH :( ...thank you very much

Christian Mangeng
15,970 PointsHi Jay,
your run_lap method is missing the length parameter:
def run_lap(self, length):
Also, you dont't need a length attribute in the RaceCar's init method. It should be laps.

Christian Mangeng
15,970 PointsHi Pankaj,
two things here:
1) You now need to add the laps attribute and its value assigned to the init method of the class. This is to avoid defining a universal value for laps.
def __init__(self, color, fuel_remaining, laps=0, **kwargs)
self.laps = laps
self.color = color
self.fuel_remaining = fuel_remaining
2) You have "self.laps = laps" two times in the init method, so remove the one below the for loop
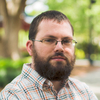
Kenneth Love
Treehouse Guest Teacher(you don't need laps
in the __init__
's arguments :D)

Christian Mangeng
15,970 PointsHi Kenneth,
thank you for the comment. I see, so it's better to do it like that?
def __init__(self, color, fuel_remaining, **kwargs)
self.laps = 0
self.color = color
self.fuel_remaining = fuel_remaining

Jay Norris
14,824 PointsHere's my best guess, but it's still just saying "Bummer, Try Again!!":
class RaceCar:
def __init__(self, color, fuel_remaining, laps=0, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.length = length
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self):
self.fuel_remaining -= length * 0.125
self.laps += 1

Chris Christensen
9,281 PointsHi Jay,
Not sure if you figured it out yet but the only thing you are missing is the attributing setting for laps.
def __init__(self, color, fuel_remaining, laps=0, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.length = length
self.laps = laps
Happy coding!
Chris
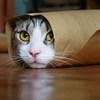
Herman Brummer
6,414 PointsHI Guys,
What does this actually mean?
"In Python, attributes defined on the class, but not an instance, are universal. So if you change the value of the attribute, any instance that doesn't have it set explicitly will have its value changed, too!"
Damn been trying to faff out what this means, but I just don't get it.
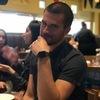
Joshua Almanza
1,646 PointsNot sure if this is still relevant to you 2-1/2 months later, but this is the example Kenneth gave near the beginning of the classes lesson about the thief with the sneaky attribute.
If he sets the class default to sneaky = True, then every thief in the class will be sneaky. When creating an instance of the class, he can set them individually to False, but if he doesn't, they'll all be sneaky by default. Same is true if he were to set them to False at the class level. if he wanted only a few thieves to be sneaky, then he would set them to True will creating the instance.
Hope that helped :)

vitaly gve
951 Pointsclass RaceCar:
def __init__(self, color, fuel_remaining, laps = 0, **kwargs)
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.fuel_remaining -= length*0.125
self.laps += 1
red_car = RaceCar(color='red', fuel_remaining=50, laps=10)

bright chibuike
3,245 Pointstry this code it will do the task: class RaceCar:
def __init__ (self,color,fuel_remaining=23,laps=0, **kwargs):
self.laps = laps
self.color =color
self.fuel_remaining = fuel_remaining
for key,value in kwargs.items():
setattr(self,key,value)
def run_lap(self, length):
self.fuel_remaining -= length*0.125
self.laps += 1
mike =RaceCar(color ="white" ,fuel_remaining =24)

vitaly gve
951 Pointshere is the right answer for me: class RaceCar: def init(self, color, fuel_remaining, laps=0, **kwargs): self.color = color self.fuel_remaining = fuel_remaining self.laps = laps for key, value in kwargs.items(): return setattr(self, key, value)
Christian Mangeng
15,970 PointsChristian Mangeng
15,970 PointsThe colon after the closing parenthesis in the init method is missing.