Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial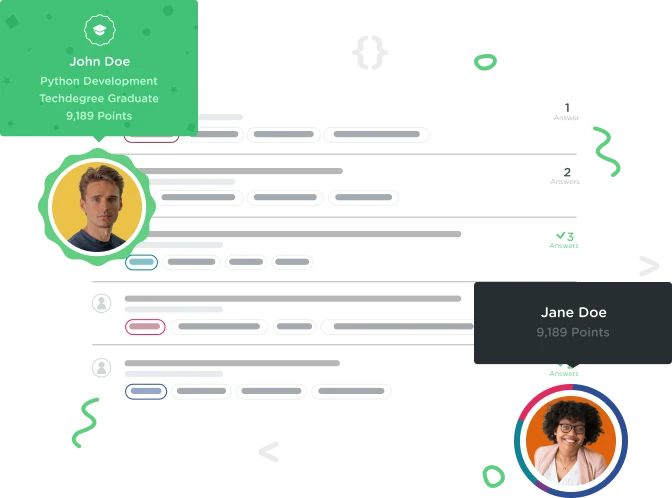

Chirstopher Herdeg
7,451 Pointsracer task 3
how to solve the task 3 problem in python OOP with the RaceCar and Laps question
class RaceCar:
def __init__(self, color, fuel_remaining,laps, **kwargs):
self.laps = 0
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
laps += 1
return fuel_remaining -= self.length * 0.125
1 Answer
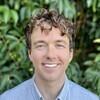
Asher Orr
Python Development Techdegree Graduate 9,410 PointsHi Chirstopher! Do you mean Task 2? I ask since the code you provided does not pass Task 2 in the challenge.
Regardless, I'll go over both questions with you.
Task 2: Add a laps attribute to the RaceCar class and set it to 0. Add a method named run_lap. It'll take a length argument. It should reduce the fuel_remaining attribute by the length argument multiplied by 0.125 (length * 0.125). Also, increment the laps attribute by 1 each time the run_lap method is called.
In your code, you are missing the laps attribute for the RaceCar class. Check out the example below- the Dog class has a class attribute called species. The value is "Canis familiaris".
class Dog:
species = "Canis familiaris"
def __init__(self, name, age):
self.name = name
self.age = age
Also, there is an issue with your run_lap method:
def run_lap(self, length):
laps += 1
return fuel_remaining -= self.length * 0.125
Remember that this method will be run on an instance of the RaceCar class. "laps" is a class attribute, so any instance of the RaceCar class will automatically have a laps value of 0. The init method defines the attributes when you make an instance. Like this:
my_instance = RaceCar("Red", 20)
In my_instance, the color is set to red. The fuel_remaining is set to 20 units. "laps" is a class attribute, so we don't need to define it upon making an instance. We know it is automatically set to 0.
To make your run_lap method work properly, you need to tell Python that the fuel_remaining and laps attribute on your instance are going to be changed whenever the run_lap method is called. They need a self. prefix attached to them.
Length is just an argument in your run_lap method, and not an attribute of the instance or class. It therefore does not need the self. prefix.
For task 3, all you need to do is set the laps attribute to 0 inside the init method.
I hope this helps. Let me know if you have any questions!