Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial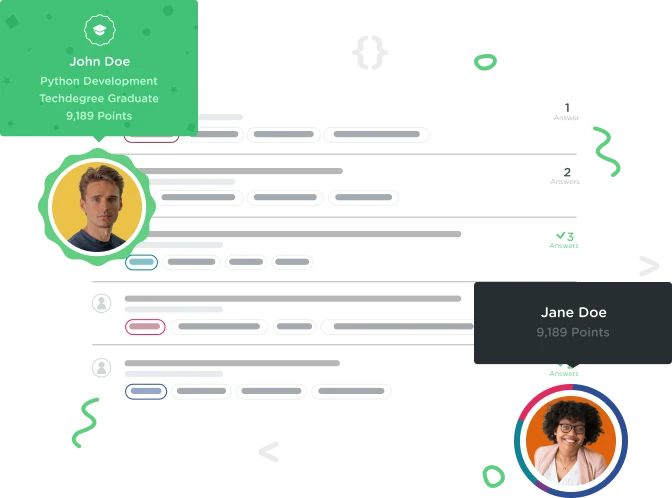

Dario Hunt
2,245 PointsRails destroy action destroying the wrong record
I posted a similar question earlier on stackoverflow and I thought that I had fixed this problem but I was wrong. I thought that the activity wasn't being deleted but turns out that it was deleting activity it was just the wrong one. I don't have this problem with any of my other models and this only happens on one of my apps. My forked app which has the exact same code works correctly. I don't know why this is happening.
projects_controller.rb
class ProjectsController < ApplicationController
before_filter :find_project, only: [:edit, :update, :destroy]
def create
params[:project][:about] = sanitize_redactor(params[:project][:about])
@project = current_member.projects.new(params[:project])
respond_to do |format|
if @project.save
current_member.create_activity(@project, 'created')
format.html { redirect_to @project }
format.json { render json: @project, status: :created, location: @project }
else
format.html { render action: "new" }
format.json { render json: @project.errors, alert: 'Please make sure all required fields are filled in and all fields are formatted correctly.' }
end
end
end
def destroy
@project = Project.find(params[:id])
@activity = Activity.find_by_targetable_id(params[:id])
if @activity
@activity.destroy
end
@project.destroy
respond_to do |format|
format.html { redirect_to profile_projects_path(current_member) }
format.json { head :no_content }
format.js
end
end
def find_project
@project = current_member.projects.find(params[:id])
end
end
member.rb
class Member < ActiveRecord::Base
def create_activity(item, action)
activity = activities.new
activity.targetable = item
activity.action = action
activity.save
activity
end
end
Migration
class CreateActivities < ActiveRecord::Migration
def change
create_table :activities do |t|
t.integer :member_id
t.string :action
t.integer :targetable_id
t.string :targetable_type
t.timestamps
end
add_index :activities, :member_id
add_index :activities, [:targetable_id, :targetable_type]
end
end
1 Answer

Dario Hunt
2,245 PointsI figured this out. I changed the way I did my destroy callbacks.
Changed the controller to this:
def destroy
@project = current_member.projects.find(params[:id])
@project.destroy
end
And added this to all the targetable models:
has_many :activities, as: :targetable, dependent: :destroy
I don't even remember why I had it set up like that, all my other polymorphic associations were set up this way.