Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial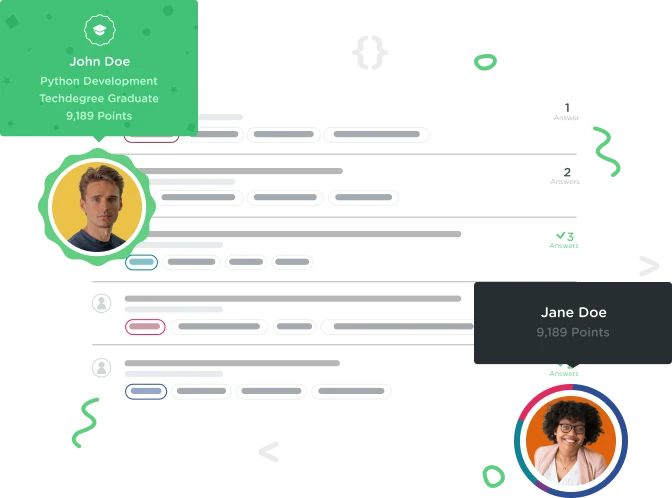

Alva Achorn
1,581 Pointsraise
Do I need to use a try/except sequence?
def suggest(product_idea):
return product_idea + "inator"
product_idea = input("Input your product idea: ")
if len(product_idea) < 3:
raise ValueError("Insufficient Characters")
1 Answer
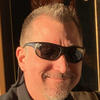
Peter Vann
36,427 PointsHi Alva!
No. But to pass the challenge/task, you need all your code to be part of the function. (So you don't need the input statement - although in the real world it might make sense to use one.)
This passes:
def suggest(product_idea):
if len(product_idea) < 3 :
raise ValueError ("Insufficient Characters")
return product_idea + "inator"
BTW, I tested the code here:
https://www.katacoda.com/courses/python/playground
(Run with >>> python3 app.py, for best results...)
Using:
def suggest(product_idea):
if len(product_idea) < 3 :
raise ValueError ("Insufficient Characters")
return product_idea + "inator"
print( suggest("TM") )
Which prints out:
Traceback (most recent call last):
File "app.py", line 6, in <module>
print( suggest("TM") )
File "app.py", line 3, in suggest
raise ValueError ("Insufficient Characters")
(As it should!!! In other words, it raised the ValueError as expected.)
This, however
def suggest(product_idea):
if len(product_idea) < 3 :
raise ValueError ("Insufficient Characters")
return product_idea + "inator"
print( suggest("Term") )
Prints out:
Terminator
Also correct/expected...
You could also do this:
def suggest(product_idea):
if len(product_idea) < 3 :
raise ValueError ("Insufficient Characters")
return product_idea + "inator"
product_name = input("What should we call your product?: ")
print( suggest(product_name) )
Which seems more like how you intended it to behave!?!!
I hope that helps.
Stay safe and happy coding!