Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial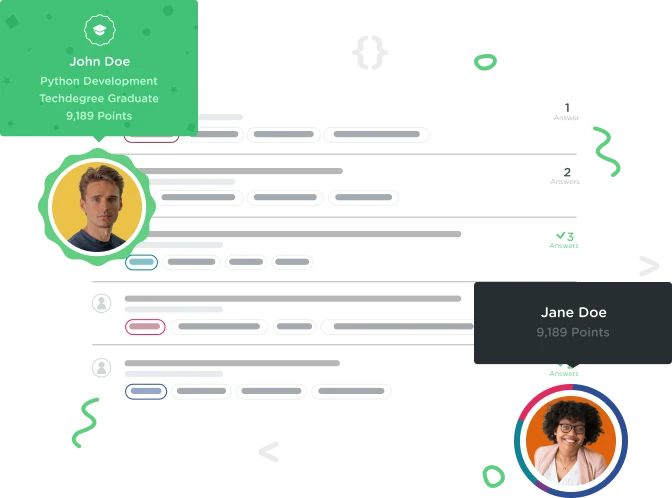
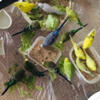
Katrine AMS Guirguis
2,587 PointsRaise an Exeption: python challenge
How do I do this??
def suggest(product_idea):
return product_idea + "inator"
product_idea = input("Did you write more than 3 characters?")
if more than 3 characters long != "yes":
raise ValueError("Are you sure you wrote more than 3?")
2 Answers
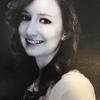
Megan Amendola
Treehouse TeacherHi, Katrine! Let me review a few things before I jump into the code.
- A string is made up of characters. There's a built-in function called
len()
that gives you the number of characters long a string is. You can pass in a string or a variable that is holding a string into the function to get the length of the string.
>>> len('string')
6
>>> test_string = 'string'
>>> len(test_string)
6
- You have access to math symbols when programming. You can add
+
, subtract-
, divide/
, and multiply*
. You can also check values based on<
less than,>
greater than,==
equals,<=
less than or equals,>=
greater than or equals.
>>> 5 > 3
True
>>> 3 < 5
True
>>> 5 == 3
False
- Whenever you see the keyword
if
you are probably going to need a conditional statement. A conditional statement checks if something isTrue
and if it is, does some logic. You can also add another conditional withelif
to see if something else is true. You can also end a conditional with anelse
to cover any other options.
num = 5
if num > 3:
# do something if num if greater than 3
elif num < 3:
# do something if num is less than 3
else:
# do something if neither of the above two conditions are True
# like if the num was 3 this logic would run because
# it doesn't fit under either of the above conditions
Now, let's look at the code challenge. The first two sentences are fluff so we can ignore them. The last portion has the important information. (Tip: Also, always stick to what the code challenge is asking) Can you please raise
a ValueError
if the product_idea
is less than 3 characters long? product_idea
is a string.
Breakdown:
-
product_idea
is a string - Why is this important? If it's a string, then we can use string methods to interact with it. Hint:len()
-
if the
product_idea
is less than 3 characters long - Ok, we have the tools to translate this into code, soif len(product_idea) < 3:
-
raise
aValueError
- This one you had -raise ValueError("Are you sure you wrote more than 3?")
Ok, now put it all together. This should all go inside of the given function.
def suggest(product_idea):
if len(product_idea) < 3:
raise ValueError("Are you sure you wrote more than 3?")
return product_idea + "inator"
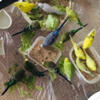
Katrine AMS Guirguis
2,587 PointsThank you!!