Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial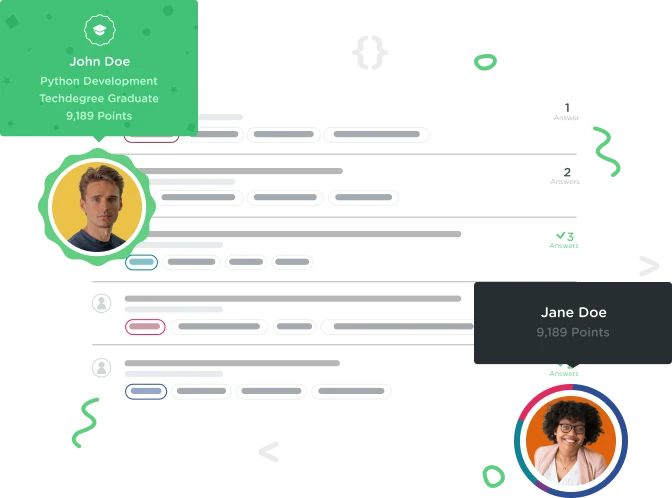

David Jeon
Courses Plus Student 573 Pointsrandom
I forgot how to write this code. Please help me
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
Dustin James
11,364 PointsSteven Parker, duly noted.
2 Answers

markmneimneh
14,132 PointsHello
the challenge is asking the following:
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
so you have a string "Treehouse" which nothing more than an iterable of chars. To prove thsi:
for letter in "Treehouse":
print(letter)
will produce
T
r
e
e
h
o
u
s
e
the length of the string is len("Treehouse")
print(len("Treehouse")) should result in 9
so now the challenge is asking you to create a function named random_item which you are going to pass a string as a param
def random_item(string):
in this function you want to grab a random number between 1 and the length of the length of the string
this should do it
print(random.randint(1,len(string)))
therefore, so far we have
def random_item(string):
a_random = random.randint(1,len(string))
we now just need to return the letter at position a_random
this would be return string[a_random]
so now now we have a full function
def random_item(string):
a_random = random.randint(1,len(string))
return string[a_random]
and to call it, I would do this
a_letter = random_item("TennesseeVolsAreGreat")
print(a_letter)
There you got it.
I hope that by walking you through the thought process, this will help you break down a larger problem into smaller logical steps through which you can solve much original problem.
please tweak the example I provided so meet the challenge requirements. For example, the challenge is expecting you to print from inside the function.
good luck
Dustin James
11,364 PointsThere is one issue with this code:
a_random = random.randint(0, len(string)-1)
This will give a random number between 0 and the number of indices in the iterable.
a_random = random.randint(1, len(string))
This will give a random number between 1 and the number of items in the iterable. With this code you will never get the first item in the iterable and you will possibly get an error for an index being out of range.
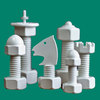
Steven Parker
230,274 PointsMark, Did you intend for that entire thing to be shown as one large block of code?

markmneimneh
14,132 Pointshi ...
@Dustin James ... yes, it should be 0 , not 1... thanks for catching.
@Steven Parker ... yes, but as a walk through on problem solving ... not a bock of run'able code ... please read the initial request.
thanks
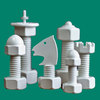
Steven Parker
230,274 PointsI get the content ... it was the formatting that was rather unconventional.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsIt doesn't look like you've written any code yet. There's a partial example in the comments. Plus the challenge tells you what function you need to call to get a random number. Surely you can get at least that far.
Please give it your best "good faith" attempt, and then post your code if you still need some help.
If you're totally lost, it might be a good time to go back and review some of the videos.