Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial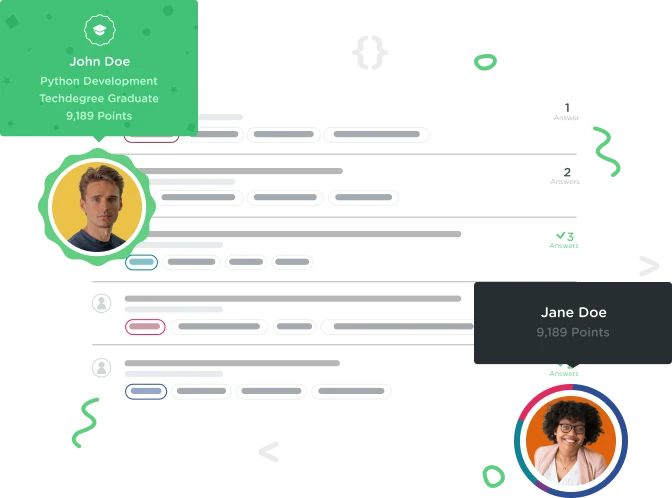

Aled Evans
3,163 PointsRandom Background Color
Hello all, could someone please help me here? I have a div called
<div class="posts">
and part of the css is
#posts:before {
content: "";
position: absolute;
background-color: #39ADD1;
}
Now I would like to get the background colour to change randomly every time the page is loaded, but it will only load certain colours (specified beforehand).
How would I do this?
Regards
5 Answers
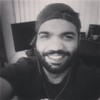
Armin Broubakarian
15,316 PointsMy apologizes I thought you requested complete random colors. Here is your answer without having to rely on jQuery . Pure raw JavaScript and nothing more:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf=8">
<style type="text/css">
#posts {
width: 90%;
height: 700px;
margin: auto
}
</style>
</head>
<body onload="return ran_col()">
<div id="posts">
</div>
<script type="text/javascript">
function ran_col() { //function name
var color = '#'; // hexadecimal starting symbol
var letters = ['000000','FF0000','00FF00','0000FF','FFFF00','00FFFF','FF00FF','C0C0C0']; //Set your colors here
color += letters[Math.floor(Math.random() * letters.length)];
document.getElementById('posts').style.background = color; // Setting the random color on your div element.
}
</script>
</body>
</html>
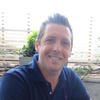
Gareth Gomersall
2,890 PointsCould some one help me with this? I'm trying to use the following:
window.onload = function(){
function rand_color() {
var color = '#';
var letters = ['000000','FF0000','00FF00','0000FF','FFFF00','00FFFF','FF00FF','C0C0C0'];
color += letters[Math.floor(Math.random() * letters.length)];
document.getElementsByClassName('main')[0].style.background = color;
}
rand_color();
}
calling it in my html like this:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Bali Chonk</title>
<link rel="stylesheet" href="css/style.css">
<script type="text/javascript" href="js/script.js"></script>
</head>
<body class="main">
<div>
<h2>Some text to go here for Bali Chonk</h2>
</div>
</body>
</html>
but it doesn't seem to work, any help would be greatly appreciated
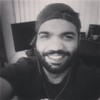
Armin Broubakarian
15,316 PointsHi Aled,
I was not sure if you wanted to use your div with a class or id. In your css it looks like you are calling in id and not a class. So in the mark-up I changed the class to an id.
I would start off by writing a function using JavaScript like this:
function ran_col() { //function name
var color = '#'; // hexadecimal starting symbol
var letters = '0123456789ABCDEF'.split(''); //hexadecimal color letters
//For loop that will create random hexadecimal value.
for (var i = 0; i < 6; i++) {
color += letters[Math.round(Math.random() * 15)];
}
document.getElementById('posts').style.background = color; // Setting the random color on your div element.
}
On your make up you want to call your JavaScript function "onload" in your body:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf=8">
<style type="text/css">
#posts {
width: 90%;
height: 700px;
margin: auto
}
</style>
</head>
<body onload="return ran_col()">
<div id="posts">
</div>
</body>
</html>

Aled Evans
3,163 PointsThanks for replying, but I couldn't see where I would be able to enter a list of colours to use :)
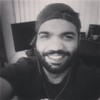
Armin Broubakarian
15,316 PointsMy apologizes I thought you requested complete random colors. Here is your answer without having to rely on jQuery . Pure raw JavaScript and nothing more:
,,,
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf=8"> <style type="text/css"> #posts { width: 90%; height: 700px; margin: auto } </style> </head> <body onload="return get_random_color()"> <div id="posts"> </div> <script type="text/javascript"> function get_random_color() { //function name var color = '#'; // hexadecimal starting symbol var letters = ['000000','FF0000','00FF00','0000FF','FFFF00','00FFFF','FF00FF','C0C0C0']; //Set your colors here color += letters[Math.floor(Math.random() * letters.length)]; document.getElementById('posts').style.background = color; // Setting the random color on your div element. } </script> </body> </html>
,,,
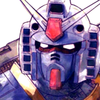
Guled A.
10,605 PointsYou may need to know Jquery to do this, but I will explain the syntax: First the demo
$(document).ready(function(){
var colors = ['#e67e22', '#3498db', '#9b59b6', '#e74c3c', '#2c3e50'];
$('body').css({'background': '' + colors[Math.floor(Math.random() * colors.length)]});
});
First you make an array with all the colors you want to load when I reload the page.
var colors = ['#e67e22', '#3498db', '#9b59b6', '#e74c3c', '#2c3e50'];
Then you use a method in Jquery to focuses on the elements css attributes specifically the background. I chose the bode element, but you can change it to which ever element you want. The method goes through each of the colors I've specified and puts them on the screen when reloaded randomly.
$('body').css({'background': '' + colors[Math.floor(Math.random() * colors.length)]});
For your example. The syntax would look like this.
$(document).ready(function(){
var colors = ['#e67e22', '#3498db', '#9b59b6', '#e74c3c', '#2c3e50'];
$('#post').css({'background': '' + colors[Math.floor(Math.random() * colors.length)]});
});

Aled Evans
3,163 PointsThanks for the help, it works perfectly. :)

Joel Ekyeah
239 PointsHi there,
I've copied and pasted the example above and configured for my use and it now looks like this:
<script type="text/javascript">
function get_random_color() { //function name
var color = '#'; // hexadecimal starting symbol
var letters = ['000000','FF0000','00FF00','0000FF','FFFF00','00FFFF','FF00FF','C0C0C0']; //Set your colors here
color += letters[Math.floor(Math.random() * letters.length)];
document.getElementById('header').style.borderBottom = color; // Setting the random color on your div element.
}
</script>
```html<body onload="return get_random_color()">
</body>
My issue is that whenever the page loads the borderBottom of my header disappears. Does anybody know why this happens and how could i fix it?
Thanks in advance for your help.
Aled Evans
3,163 PointsAled Evans
3,163 PointsThat is even better. Thanks for the help :)
Armin Broubakarian
15,316 PointsArmin Broubakarian
15,316 PointsAnytime, happy to help. Cheers!
Guled A.
10,605 PointsGuled A.
10,605 PointsGood implementation. Over the past few weeks I've been using Jquery, I'm quite use to it. Nice work. Raw Javascript is the way to go.
Aled Evans
3,163 PointsAled Evans
3,163 PointsSorry one more question! How can I get it to add the same background colour to more than one div. So #post and #blog? - Thanks
Guled A.
10,605 PointsGuled A.
10,605 PointsI believe you'd have to target that div just like what was done in Armins code with the post div.
document.getElementById('blog').style.background = color; // Setting the random color on your div element.
They both should display the same colors.
Aled Evans
3,163 PointsAled Evans
3,163 PointsPerfect that worked like charm on CodePen. Thank you for the help the both of you.
Armin Broubakarian
15,316 PointsArmin Broubakarian
15,316 PointsYup Guled A is correct. Here is a sample:
Aled Evans
3,163 PointsAled Evans
3,163 PointsSorry guys, I need some more help? It won't work!
Okay so here is my whole code for the header of my Wordpress site?
and
document.getElementByClassName('sidemar')
Can I not add a ":" to the ID name and not use "ByClassName" for ".sidebar" What seems to be the problem?
Regards
Aled
Armin Broubakarian
15,316 PointsArmin Broubakarian
15,316 PointsI don't think You can use ":before" the way you have it in your code.
document.getElementById('posts:before').style.background = color;
also you are missing the 's' in document.getElementsByClassName. Try something like this:
document.getElementsByClassName('sidebar')[0].style.background = color;
Here is your entire code. I took out all the things that were referencing to other files to make it work locally on mine. Just compare it with yours and start adding in the other lines and see what is causing it to not work.
Here is the working code I made for you:
I hope this helps.
Caleb Shook
2,357 PointsCaleb Shook
2,357 PointsDude. Sick code.
var swag = "Good Job!"
console.log(swag)