Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial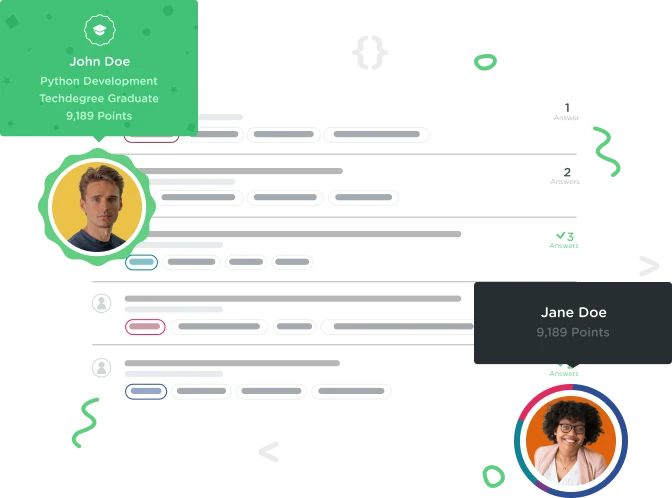
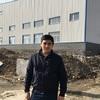
ali mur
UX Design Techdegree Student 23,377 PointsRandom Color Generator with a button click
I am trying to create a random color generator with a button click. After i click the button the color changes once, so i need to put it in a loop. Please help me on this,
HTML
<h1>This is a border H1</h1>
</div>
<button id="mybutton">CHANGE DIV COLOR </button>
JS
const mydiv=document.querySelector('div');
const mybutton=document.getElementById('mybutton');
const rannumber1=Math.floor(Math.random() * 256 );
const rannumber2=Math.floor(Math.random() * 256 );
const rannumber3=Math.floor(Math.random() * 256 );
const ranrgb="rgb("+rannumber1+","+rannumber2+","+rannumber3+")";
mybutton.addEventListener('click', () => {
mydiv.style.backgroundColor=ranrgb;
} );```
5 Answers

Michal Zuber
3,472 PointsYour code executes only once because random color is generated once. You declared your constants outside event so it's only generated once page is loaded. First click of button gives your div color and next clicks keeps changing color of the div for the same div over and over again so you can't see it. Solution is to put your colors inside click event so random color is generated each time someone click the button. Second thing is I don't see a reason to use const instead of var. So here's your code:
const mydiv=document.querySelector('div');
const mybutton=document.getElementById('mybutton');
mybutton.addEventListener('click', () => {
var rannumber1=Math.floor(Math.random() * 256 );
var rannumber2=Math.floor(Math.random() * 256 );
var rannumber3=Math.floor(Math.random() * 256 );
var ranrgb="rgb("+rannumber1+","+rannumber2+","+rannumber3+")";
mydiv.style.backgroundColor=ranrgb; }); ```
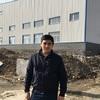
ali mur
UX Design Techdegree Student 23,377 PointsI have edited it. I am trying to get a new color, everytime I click the button.
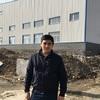
ali mur
UX Design Techdegree Student 23,377 Pointsjust thx
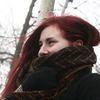
Ella Ruokokoski
20,881 Pointshere's an alternative solution with a function that creates a six-digit hexadecimal color code
const mydiv=document.querySelector('div');
const mybutton=document.getElementById('mybutton');
function randmColor() {
let color = "#";
for(let i = 0; i < 6; i++){
color += Math.floor((Math.random() * 16)).toString(16);
}
return color;
}
mybutton.addEventListener('click', () => {
mydiv.style.backgroundColor=randmColor();
});
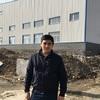
ali mur
UX Design Techdegree Student 23,377 PointsElla, what does toString method do?
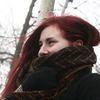
Ella Ruokokoski
20,881 PointsIt converts a number to a string. The number inside the parenthesis is the base or radix used when coverting a number to string. It is optional but in this case there needs to be a base set so we will get a hexadecimal. The base of the hexadecimal system is 16, so that is the attribute given to the toString() method.
Michal Zuber
3,472 PointsMichal Zuber
3,472 PointsIs this your full code? Cause it looks like your button is missing. Also you should edit your post and format your code properly, it will be way easier to read it.