Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial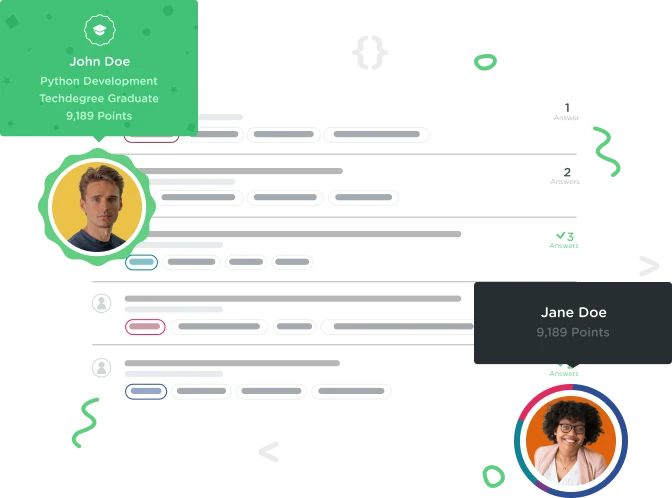
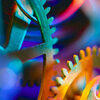
ja5on
10,338 Pointsrandom formula
Math.floor(Math.random() * (6 - 1 + 1)) + 1;
gets changed to
Math.floor(Math.random() * (highNum - lowNum + 1)) + lower;
I cannot ever memorise where highnum or lownum go, it just doesnt compute for me, i
spent weeks trying to get it.
What do i do now?
3 Answers
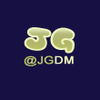
Jonathan Grieve
Treehouse Moderator 91,253 PointsThe best way to memorise the problem is to keep attempting. We all learn best in programming by doing things. This way you'll be more empowered to tacklem new problems as they come. And at the end of the day that's what coding is all about.
Anyway let me try and get your head around this for you. I confused myself in my previous answer to you.
function getRandonNumber(lower, upper = 100) {
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
This is one example solution... the one as defined in the solution video. :)
It uses a function definition as an example rather than an arrow function and takes 2 parameters. One is the lowest range of random numbers and the other is the highest, with a default value.
Inside it returns the formula. It defines Math.floor()
which itself contains a range between the upper lower and the lower number. We're saying +1 in the formular becuse we want to ensure we get to the highest range of the random number if it is generated.... by default, the methods don't do it. And then we add the value of lower
to get the calculated lower value range.
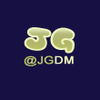
Jonathan Grieve
Treehouse Moderator 91,253 PointsIt seems like you're getting some practice in with functions, which is why you're changing the integers into variable names like highNum
and lowNum
.
function randomNumber(highNum, lowNum = 100) => {
return Math.floor(Math.random() * (highNum - lowNum + 1)) + nowNum;
}
Then pass the numbers, (the integers) into your function call.
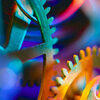
ja5on
10,338 Pointsfunction randomNumber(highNum, lowNum = 100) => { return Math.floor(Math.random() * (highNum - lowNum + 1)) + nowNum; } So if I call:- randomNumber(10, 50); it means 50 - 10 gives a difference of 40 40 + ?
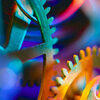
ja5on
10,338 PointsI think this question is all wrong
I want to know how I can memorize/understand the formula
Victor Stanciu
Full Stack JavaScript Techdegree Student 11,196 PointsVictor Stanciu
Full Stack JavaScript Techdegree Student 11,196 PointsThis is how I managed to understand and learn the formula. Think about mathematical intervals https://en.wikipedia.org/wiki/Interval_(mathematics). First, you need to remember a few things:
Math.random()
generates a number in the half-open interval[0,1)
(means numberX
can take a value from0
up to0.9999
.... , but never1
).Math.floor()
takes the random number, say10.4567..
and takes the first integer lower that that number (in this case,10
).[a , b) - c = [a - c , b - c)
. Simple!First and most simple example is getting a random number between lets say
1
and10
. There a three steps to achieve that.[0,1)
by10
, getting a new half-open interval[0,10)
meaningX
cand get a value from0
up to,9.9999...
, but never10
.1
to the interval, and get[1,11)
, meaning X can get a value from1
up to10.99...
but never11
.Math.floor()
comes in handy (see the example above).Take note that the addition of
1
is the last mathematical step to getting the desired half-open interval!!Now, lets say you want a random number between
3
and7
. For this, you obviously can't just use the exact same steps from above. I find the easiest way to be the reverse-engineering of the desired interval, meaning: We want the interval[3,7)
and need to do some basic math operations to get to the interval[0,1)
. Keep in mind the wayMath.floor()
works!! This means we are actually working with the interval[3,8)
(just like in the above example, were we got interval[1,11)
).[3,8)
substract the lower limit of the interval, meaning3
, like this:[3,8) - 3 = [0,5)
.[0,5)
to the upper limit, meaning5
, to get theMath.random()
interval:[0,5) / 5 = [0,1)
.I hope this helps! Good luck in your coding adventures!