Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial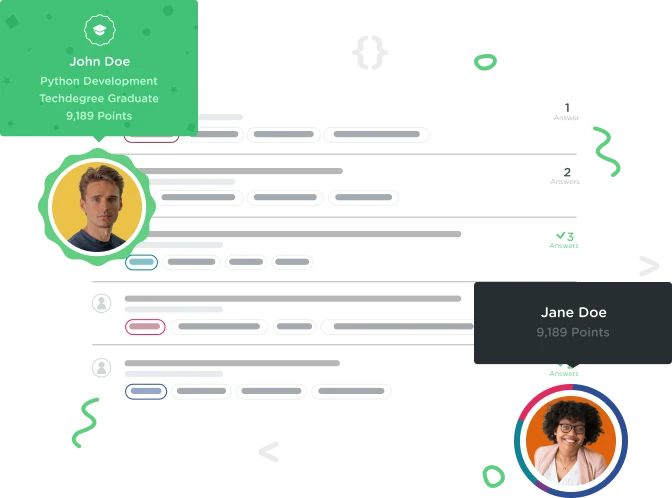

Alwan Mortada
1,598 PointsRandom Number Challenge, Part II
function random (lower, upper){ if ( isNaN(lower) && isNaN(upper) ){ return Math.floor(Math.random() * (upper - lower + 1)) + lower; } else { return alert(" is not a number"); } } console.log(random( 34, 45)); console.log(random( "nine",30));
I'm getting undefined random.js:10 undefined random.js:11
How come !??
2 Answers
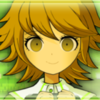
Kenneth Black
23,140 PointsIt's returning undefined because you can't return a console.log. It will run the console log first, but then register an empty return field. in this case, you can just omit the console log and just return " is not a number".
As for the function, isNaN stands for "is Not a Number", so you actually want to check if isNaN is false for the first condition if you want to make sure they both are numbers. the ! operator will allow us to check for false values.
So, what you want is:
function random(lower, upper) {
if (!isNaN(lower) && !isNaN(upper)) {
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
} else {
return "Entry is not a number"
}
}
console.log(random(34, 45)); //returns the random number, going through the first gate
console.log(random("nine", 30)); //returns "Entry is not a number"

Alwan Mortada
1,598 PointsThank you sir!!!!