Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial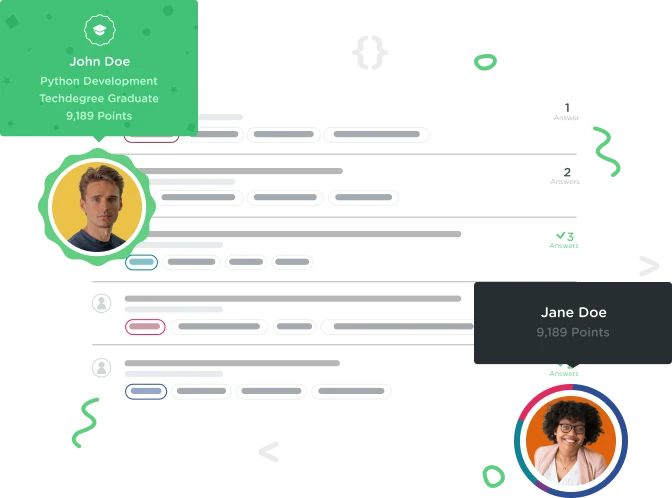
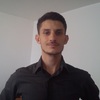
sorin vasiliu
6,228 PointsRandom Number Challenge, part II - Quick Question!
Hey guys, so this is my code for the RN part II :
function randomNumber( lower, upper ) {
if ( isNaN(lower) === true || isNaN(upper) === true ) {
throw new Error('One of the arguments is Not-A-Number! The function accepts only Numbers!');
} else {
var randomNum = Math.floor(Math.random() * (upper - lower + 1)) + lower;
return document.write('<p><strong>Your number is ' + randomNum + ' !!</strong></p>');
}
}
Is the ""throw new Error"" part written correct ? I ask this because when I test the script with NaN it doesn't give any error messages. Not OnScreen nor in the console. It just doesn't run, that's it. Am I doing it wright ?
Second: If I call the function out 5 times, and the first call of the functions contains a NaN, then the script stops there and doesn't go to the other 4 functions below that one, is that normal? Or should it give a error message for the first and then go on to the rest...
Thanks!
3 Answers

Daniel Newman
Courses Plus Student 10,715 PointsMy personal recommendation: isNaN(undefined); //true
But you can compare NaN !== NaN; // true I think you probably did not defined variables or missprint variable name or I did not get your question clear.
About this code and NaN test that never throw an exception:
if ( isNaN(lower) === true || isNaN(upper) === true )
If you pass lower as NaN it will throw you to the else statement If you will do the same with upper it will also throw you to the else statement Because ( true || false ) or ( false || true ) are both equal true
Why did not you make "console.log()" of your opperator to debug process?
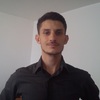
sorin vasiliu
6,228 PointsHi,
I'm still getting the hang of this so please bare with me if I seem not to understand.
So this is the code that works:
function randomNumber( lower, upper ) {
if ( isNaN(lower) === true || isNaN(upper) === true ) {
throw new Error('The app accepts only numbers! One of the args is not a number!')
} else {
var randomNum = Math.floor(Math.random() * (upper - lower + 1)) + lower;
return document.write('<p><strong>Your number is ' + randomNum + ' !!</strong></p>');
}
}
randomNumber(1, 200);
randomNumber('two', 10);
//randomNumber('chicken', 120);
//randomNumber(' ', 90);
//randomNumber('dope', 20);
//randomNumber(' ', 20);
//randomNumber(12, 400);
Now it runs good and it gives the correct Error message in the console. With this code if ( isNaN(lower) === true || isNaN(upper) === true ) {
throw new Error('The app accepts only numbers! One of the args is not a number!')
}
I want the program to check if either lower or upper is a NaN, and if it is, to display that error message. And the 'else' statement to run only if both the "if" statements are false. (both are numbers).
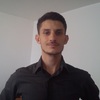
sorin vasiliu
6,228 PointsDunno why I did not use console.log :P Skipped my mind that part. But I've seen the solution now and it seems to be in order. This NaN function is pretty tricky from what I've seen on the web.... Thanks for your help!

Daniel Newman
Courses Plus Student 10,715 PointsI think you can keep your code shorter
if ( isNaN(lower) || isNaN(upper) ) {
function randomNumber( lower, upper ) {
console.log( isNaN(lower) || isNaN(upper) );
}
randomNumber(1, 200); //false
randomNumber('two', 10); //true
randomNumber('chicken', 120); //true
randomNumber(' ', 90); //false
randomNumber('dope', 20); //true
randomNumber(' ', 20); //false
randomNumber(12, 400); //false
And if you want to throw error in case when they are both NaN you should use AND (&&) but not OR (||).

johnnyesper
5,935 Pointsfunction getRandomNumber( lower, upper ) {
if ( isNaN(lower) === true || isNaN(upper) === true ){
throw new Error("Error dude, not a number");
} else{
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
}
console.log( getRandomNumber( 32, 24 ) ); console.log( getRandomNumber( 1, 100 ) ); console.log( getRandomNumber( 200, 'five hundred' ) ); console.log( getRandomNumber( 1000, 20000 ) ); console.log( getRandomNumber( 50, 100 ) );
Cati lucian
10,119 PointsCati lucian
10,119 PointsSalut Sorin !
if ( isNaN(lower) === true || isNaN(upper) === true )
nu este necesar " === true " pt ca isNaN in sine iti intoarce boolean