Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial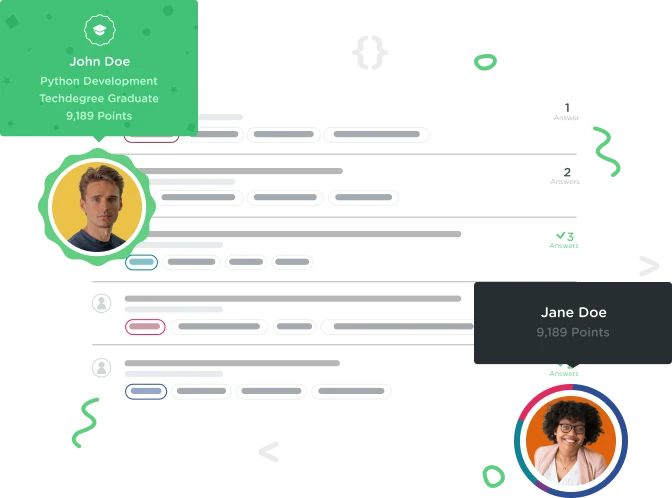

Elie Haykal
8,163 PointsRandom Number Duplicates
Hello,
What is the most efficient way of disallowing the random number generator to generate two consecutive numbers which are the same ?
I created another variable which holds the previously generated number. Once a number is generated, the newly generated number and the variable are compared to see if the number is the same.
I am able to detect it but how can I stop it from occurring.
Thanks
4 Answers
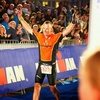
Steve Hunter
57,712 PointsYeah - that would work. Alternatively, generate two random numbers and perform some mathematical function with them; that makes the chances of two being the same smaller.
Alternatively, you can generate two random numbers:
Random rNumber = new Random();
int first = rNumber.nextInt(10);
int second = rNumber.nextInt(10);
if (first == second) {
//do something to break the equality
first++;
second--;
}
I don't know what your requirement is or why two numbers being the same is a problem. But there's simple ways round that, as above.
Steve.

Elie Haykal
8,163 PointsHi Steve,
Thanks for the answer. Yes I am aware of the fact that I am unable to influence the random generation of numbers but what if after we generate the second number then compare it to the previous one. If they are the same then maybe call for another generated number until we get a unique number.
That is what I practically had in mind,
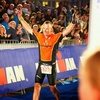
Steve Hunter
57,712 PointsIt is a random number generator. By definition, the production of the next number is not influenced by the value of any other number, including the previous one.
Steve.
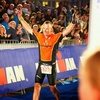
Steve Hunter
57,712 PointsSurprising how often it occurs - this logs "Matched!" when the next number is the same as the last.
int lastRandom = 0;
Random randomGenerator = new Random();
for(int i = 0; i < 1000; i++){
int nextRandom = randomGenerator.nextInt(100);
if(lastRandom == nextRandom){
System.out.println("Matched!");
// generate another number
nextRandom = randomGenerator.nextInt(100);
// or just increment
nextRandom++;
}
lastRandom = nextRandom;
System.out.printf("%s ", lastRandom);
}