Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial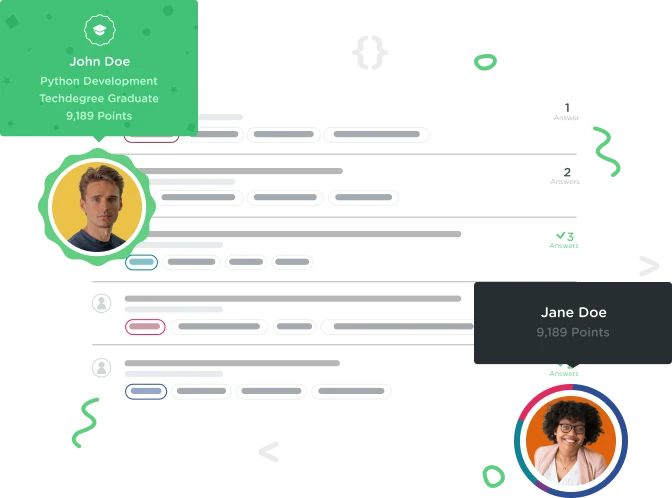

Vince Leung
4,730 PointsRandom number function
Did i put in the wrong formula or made a mistake on my parameters? I seem to be getting a number that isnt in the range of my minimum or maximum number.
Any help would be appreciated THANKS!
function calc(minN ,maxN){
var randomN = Math.floor(Math.random() * (maxN - minN + 1)) + minN;
return randomN;
}
var lower = prompt("Please enter first minimum number");
var upper = prompt("Please enter second maximum number");
alert ("This is your new number " + calc(lower, upper));
5 Answers

Vince Leung
4,730 PointsI appreciate it guys :) Thanks!

Vince Leung
4,730 PointsFor some reason, still spitting out wrong results. It's not producing a number between the two prompted numbers.
Not sure...
function calc(minN ,maxN){
return Math.floor(Math.random() * (maxN - minN + 1) + minN);
}
var lower = prompt("Please enter first minimum number");
var upper = prompt("Please enter second maximum number");
alert ("This is your new number " + calc(lower, upper));
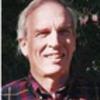
jcorum
71,830 PointsVince, I copied your code, pasted it into an online JavaScript tester (https://jsfiddle.net/) and ran it a dozen times. Each time I get a number between the min and max. You might want to try that, just to see if you get the same results.

Vince Leung
4,730 PointsHmm, it seemes like the formula was actually right. I don't know how your jsfiddle worked, because my codepen didnt work. The formula i had was actually correct as explained here: http://stackoverflow.com/questions/1527803/generating-random-whole-numbers-in-javascript-in-a-specific-range .
I'm not 100% sure sure if this was right solution but it worked... i change my prompt to have the parseInt(), as they were strings, so that could be reasoning i was getting these weird numbers.
Here is my solution and seems to be working: http://codepen.io/leungvi8/pen/NrPxZq
I appreciate your help jcorum :)!
Vince
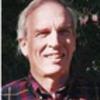
jcorum
71,830 PointsVince, you have one of the parentheses in the wrong place in your formula. Try this instead:
function calc(minN ,maxN){
return Math.floor(Math.random() * (maxN - minN + 1) + minN);
}
Aaron Martone
3,290 PointsAaron Martone
3,290 PointsAs an aside, there is no point in creating a variable in the function just to immediately return it in the next line. As in jcorum's example, you simply return the expression. It is evaluated first and then sent to
return
which returns the value, without the unnecessary need to create a variable (uses memory) since it is only scoped to the function and not available elsewhere.