Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial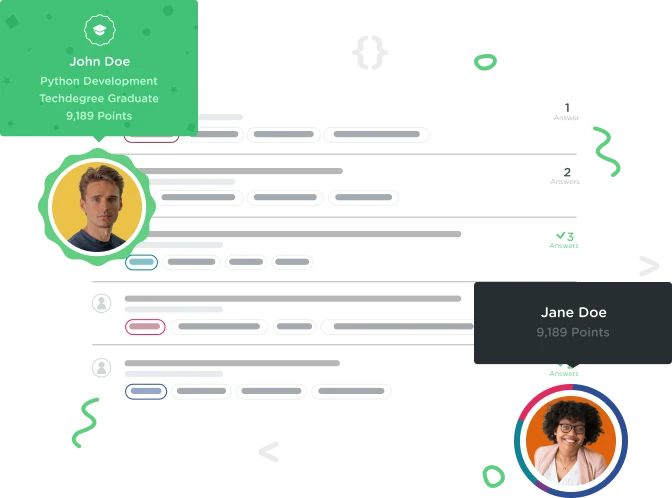
Justin Henderson
13,739 PointsRandom number generator
I am making a random number generator game. What would be the best function to use to generate output? I'm thinking of using a loop convention....? Thank you for your input :)
4 Answers
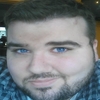
Marcus Parsons
15,719 PointsWell, you should give the user the option to choose whether or not they want to keep receiving results, especially if you want to do them via alert boxes as those can be especially annoying. You also need a way to break out of the while loop, otherwise, you might end up crashing the browser. So, to combine those two things, here's a simple solution:
//Set upper limit for results, this will define the maximum number a result can be
var upperLimit = 5000;
var response;
while (true) {
response = prompt("Want to see some results? Just hit enter or enter anything else/press cancel to quit!");
if (response === undefined || response === "") {
alert(Math.ceil(Math.random() * upperLimit));
}
else {
break;
}
}
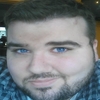
Marcus Parsons
15,719 PointsHere's a little page I made up that uses what I was talking to you about. It does not use document.write() because that should really be avoided at all costs. This little program spits out two results every second.
If you have any questions about it, I'll answer them for ya!
<!DOCTYPE html>
<html>
<head>
<title>Infinite Results</title>
<style>
#output {
margin-top: 50px;
}
#input {
width: 500px;
margin-right: 20px;
}
#stopButton {
margin-left: 20px;
}
</style>
</head>
<body>
<input type="text" id="input" placeholder="Enter a maximum random number. Press Go! to go and Stop! to stop."><button id="goButton">Go!</button><button id="stopButton">Stop!</button>
<div id="output"></div>
<script type="text/javascript">
var timer;
function printout(msg) {
document.getElementById('output').innerHTML += msg;
}
function getRandNumber(limit) {
return Math.ceil(Math.random() * limit);
}
document.getElementById('goButton').addEventListener('click', function () {
var input = document.getElementById('input');
if (input.value !== "" && !isNaN(input.value)) {
var upperLimit = parseInt(input.value, 10);
timer = setInterval(function () {
printout('<p>Result: ' + getRandNumber(upperLimit) + '</p>');
}, 500);
}
}, false);
document.getElementById('stopButton').addEventListener('click', function () {
clearInterval(timer);
}, false);
</script>
</body>
</html>
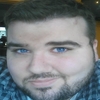
Marcus Parsons
15,719 PointsHey Justin,
It really depends on what you're doing with the numbers. Can you give us some more information about what you plan to do with the game? If it's top secret, then just kind of a brief summary is a-okay!
Justin Henderson
13,739 Pointslol Marcus Parsons, not top secret. I want the user to click the button and generate an infinite amount of results. Once the button is clicked an alert will produce the output of the function. Maybe generate some sort of onclick function?
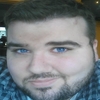
Marcus Parsons
15,719 PointsAre you going to display each result individually or total them up for one result?
Justin Henderson
13,739 Pointsindividually ideally... but I do like the idea of totaling them up. Let's just say individually for this instance though
Justin Henderson
13,739 PointsJustin Henderson
13,739 PointsThank you Marcus! This is very helpful, now I just have to add a button and an event listener to the code for some interactivity. Thanks again!