Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial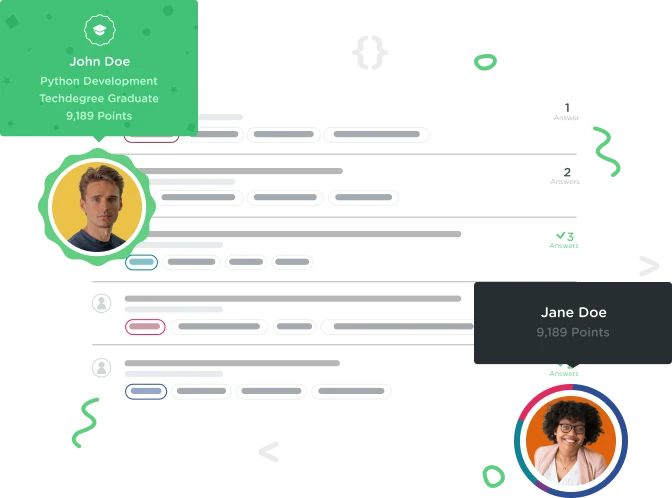

Fanny Villarosa
2,026 Pointsrandom number generator
in generating a random number from 1 to 6 and having to add + 1 at the end, can't we just change it to *7 instead of *6? such as:
Math.floor(Math.random() * 7)
instead of
Math.floor(Math.random() * 6) + 1
5 Answers

Aakash Srivastava
5,415 PointsHey Fanny Villarosa , you can do , but it totally depends on whether you want to get zero or not.
If you want to get result inclusive of zero , then you can go with
Math.floor(Math.random() * 7)
because ,
math.random()
return a random number between 0 (inclusive) and 1 (exclusive) .
But , if you don't want zero i,e exclusive of zero , then in order to set minimun value of 1 , you have to add it in the last like this :
Math.floor(Math.random() * 6) + 1
Notice that , the upper limit of both the methods are same but the lower limit is different.
In the unit , it ask you numbers from 1 to 6 , so you have to go with second method
Hope this helped :)

Sergio Leon
12,594 PointsAs Sunny has told you, you can do it, but there is the possibility of getting a 0.
There would be cases where you want to keep that possibility, for example, if you want to generate a random number to use it to get items from an array. In that case, you wouldn't need to add a +1.
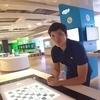
Marco Cornejo
3,412 PointsMath.floor(Math.random() * 7)
//Possible Results: 0, 1, 2, 3, 4, 5, 6, 7
Math.floor(Math.random() * 6) + 1
//Possible Results: 1, 2, 3, 4, 5, 6
The instructor is simulating a Dice Roll, since you can never roll a 6-sided dice and get either a 0 or a 7, the logic of multiplying by 7 is flawed.
Math.floor() truncates the number, and since Math.random gives a number between 0.00 and 0.9999~, flooring it would always result in a 0.
The multiplication *6 is used before flooring, this way if random gives us 0.5552, we multiply it by 6, equal to 3.3312, fooring it leaves us with 3.
There is a really small chance, but still possible, that Math.Random gives us a 0.00, which if we multiply it, we still have a 0, and since 0 has no place in a dice, we have to add the '+1' to our algorithm.
The highest number we can receive is a 0.9999, multiply it by 6 and we have 5.9994, floor it and we have a 5. +1 equals to 6. That '+1' is meaningful and really important in this specific algorithm.
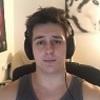
ale8k
8,299 PointsA lot of the answers here are overly complicated...
Math.floor(Math.random() * 7) // if you look at this, you're multiplying the Math.random (a number between 0 and 0.999) so just increase it by 1 again doesn't prevent us from multiplying it by 0 :).
Math.floor(Math.random() * 6) + 1 // this however is adding one after the expression "(expression)" of Math.random so it's //actually adding +1 to the end result or Math.floor in this case as well as the end result. And because Math.random //generates between 0 - 0.999 * 6 think of it like this (0 - 0.999 * 6) + 1 = between 1 and 1 * 6 :).

Fanny Villarosa
2,026 PointsThank you all for your input!