Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial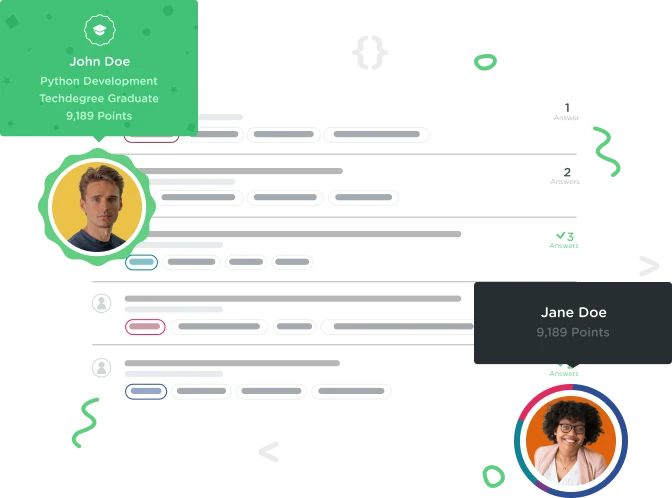
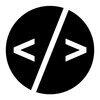
Alexis Perez
1,093 PointsRandom Number My Solution
// Collect 2 inputs (strings) from a user
const userLow = prompt ( `Random Number Generator!
Please type the LOWEST possible random number in the range of numbers starting from 1.` );
const userHigh = prompt ( `Please type the HIGHEST possible random number in the range of numbers starting from 1.` );
//Declare variables that will be store the actual numbers from the user input
let lowNumber;
let highNumber;
/*
Conditionals rules:
1- input cannot be number 0
2- input cannot be letters
3- lowest number cannot be higher than higher number
*/
if ( +userLow === 0 || +userHigh === 0 ) { // 1
alert("Do not use 0, please try again");
} else if ( isNaN(userLow) || isNaN(userHigh) ) { //2
alert("Do not use letters, please try again");
} else if ( +userLow >= +userHigh ){ //3
alert("LOWEST number is higher than HIGHEST number, please try again");
}else {
//Convert the input to a number
lowNumber = parseInt(userLow);
highNumber = parseInt(userHigh);
// Use Math.random() and the user's numbers to generate a random number
const randomNumber = Math.floor( (highNumber - lowNumber) * Math.random() + 1 + lowNumber );
// Create a message displaying the random number
document.querySelector("main").innerHTML = `<h1>Random Number Generator!</h1><h2>${randomNumber} is a random number between ${lowNumber} and ${highNumber}</h2>`;
}