Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial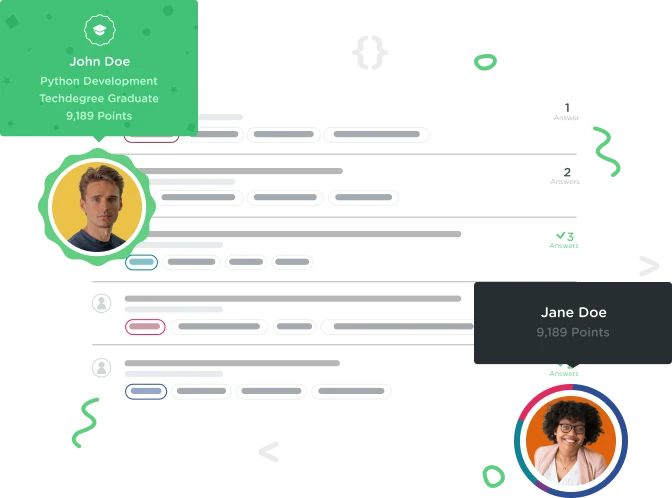

kevin cleary
4,690 Pointsrandom.choice rebuild
this is what the challenge is: You've seen how random.choice() works. It gets a random member from an iterable (like a list or a string). I want you to try and reproduce it yourself. First, import the random library. Then create a function named random_item that takes a single argument, an iterable. Then use random.randint() to get a random number between 0 and the length of the iterable, minus one. Return the iterable member that's at your random number's index.
its says that my function does not return the correct value
attached is my code, not sure if I'm going about it right or not. It is late, and I've been on treehouse all day.
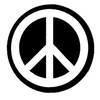
john larson
16,594 PointsMine was completely different, but it passed
import random
str = "string"
def random_item(str):
len_of_str = len(str)
rand_los = random.randint(0, len_of_str)
rand_los -=1
print(rand_los)
return str[rand_los]
I guess I broke it down a lot more cause I get confused easily.
4 Answers
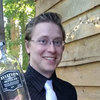
Evan Demaris
64,262 PointsHi kevin,
As the code didn't attach, I can't see what you tried. However, you could complete the challenge with the below code;
import random
def random_item(iterable):
return iterable[random.randint(0,len(iterable)-1)]
This returns the iterable argument, at the index of a random integer between 0 and one less than the length of the iterable argument.
Let me know if you have any questions about that solution. Also, if you copy and paste the code you tried I can let you know what's going wrong there.
Hope that helps!

kevin cleary
4,690 PointsThank you, that worked wonderfully. My code was almost there:
import random
def random_item(itterable):
return random.randint(0, len(iterable) - 1)
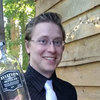
Evan Demaris
64,262 PointsYou're right, that's just one word away from being the same way I did it!

Freddy Venegas
1,226 Points"Return the iterable member that's at your random number's index."
This was confusing me for a bit, then I read your solution and also the line above from the challenge, then it made complete sense!
Thank you for this example.
By the way, I was doing this:
def random_item(thestring):
return random.randint(0, len(thestring) -1)
random_item("somethingrandom"):
which was returning only the index but not the member in that index.

Australia Kimbrough
781 PointsIm curious to know return iterable[random.randit(0, len(iterable)-1)] is in brackets. I never saw this in previous examples so I thought return iterable(random.randit(0, len(iterable)-1)) would work. Not sure why this works or when to know when and when not to use the brackets?
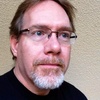
Chris Freeman
Treehouse Moderator 68,441 PointsUsing iterable[ ... ]
with square brackets is used to lookup an item in the iterable by an index number.
Using iterable( ... )
with parens would be used if iterable
was called as a function. It's not a function so this doesn't work in this case.

Michael Moore
7,121 PointsThis was what I came up with in the workshop coding window.
import random
def random_item(iterable):
random_number = random.randint(0, len(iterable)-1)
return iterable[random_number]
#print(random_number)
#print(iterable[random_number])
random_item("abcdefghijklmnop")

Mathew V L
2,910 PointsHi Micheal,
Could you explain why you used brackets for this instead of Parenthesis? "return iterable[random_number]"
This was the reason I didn't get it but I still don't know why brackets are used instead. Thanks

Michael Moore
7,121 PointsSo when I define random_number, I am saying choose a random number between 0 and the length of iteratable - 1, which is the length of the alphabet, 26, minus one, making it 25. It chooses a random number between 0 and 25 as a result. Then it is treating the string as an array, or dictionary I think, it's been a minute since I've used python. So imagine when I pass in "abcdefgh", it is turned into ["a", "b", "c"...] and so on. So it chooses a random number, let say the random number was 2 for the sake of me not having to type a million letters out. So "a" = 0, "b" = 1, "c" = 2 in the dictionary. So iterable[2] would be the string "c".
Had I used parentheses, it would have been like I was trying to call a function called iterable, and passing in the number 2,like so:
return iterable(2)
That would try to return the result of a function called iterable after passing in the number two, and that function doesn't exist, so the program would error out. See how I called the function random_item with parentheses and passed in the alphabet at the bottom of the program in order to start the program?
I'm doing this on my phone at 3 a.m. So I might not have explained it the best way, but I hope you get an idea of what I did.

Mathew V L
2,910 PointsThanks Micheal, I literally spent all night thinking about this especially since () is not needed in print or return to use it o.0
Thanks for the quick reply especially at 3AM!!!!!!
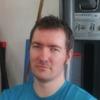
Benny Shtark
743 Pointsvery difficult challenge, no idea how to solve it myself without copy/paste from internet..
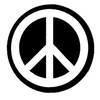
john larson
16,594 PointsI'm with you Benny. Plenty of times I reverse engineer stuff. If I can't get it, I look it up...and try and figure out why it works. Take notes and hopefully remember the concepts for next time

Gabriel Reed
560 PointsIt's no wonder online learning platforms have, at best, a 3 to 5% completion rate.
Learning new things is entirely dependent on repetition, and Treehouse just doesn't have it. Seems to be geared towards people who already know a programming language. You listening, Treehouse? Stop adding new languages and courses without perfecting the ones you already have. Your Python course, considering it's the fastest growing language in the world, should be the most comprehensive course you have. It's being neglected.
This platform is turning out to be incredibly disappointing for $25 a month. Not once prior to this exercise have they covered putting 'iterable' inside of brackets. They are moving too fast. There's not enough exercises to insure you have a solid understanding before moving on.
THEY NEED A PLETHORA OF MULTIPLE CHOICE QUESTIONS. I'm serious, like hundreds of them. We can't pull things out of thin air. And finally, no one on earth is better at making something seem trivial than Kenneth- listening to him makes me feel like I'm in Sunday school. It's literally painful, and the topics he chooses for his examples make me want to shoot myself. I can't follow anyone who's talking about apples, oranges and kitty cats.
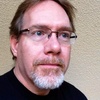
Chris Freeman
Treehouse Moderator 68,441 PointsGabriel Reed, Thank you for your feedback. Treehouse is continuously expand content to help students learn. Adding more questions is a great suggestion. I will pass it along!

Michael Moore
7,121 PointsI beat my head on a wall for a good 6 months before I started to catch on when I began programming, then it was like I was beating my head on a wall with thin cushion. It still hurt, but not as much.
Keep at it, you will have "ahah" moments where things just click all of a sudden. Play with it. Make variables, in this example, like variable = "abcdefg", then print(variable[5]} and see what turns up. Then try throwing some simple addition and subtraction in there. Try to get a feel for what the new concept is doing.
Trust me, I feel your pain. It is a slow, frustrating, agonizing process, but once you get the hang of this, and any other concept, you will understand that concept in every other language. The first language, or languages, are always the hardest.
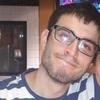
Alejandro Mesa
9,813 PointsWhy this doesn't work?
import random
def random_item(iterable):
return iterable.index(random.randint(0, len(iterable) - 1))
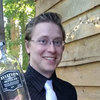
Evan Demaris
64,262 PointsHi Alejandro,
the index() function returns the number index at which a provided argument is found in the subject it's been chained to.
So your code would be returning the number index at which the random number was found in the iterable - which would throw an exception for any iterable which didn't include its own length inside itself as a value.
Hope that helps!
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsSorry the code attachment isn't working correctly. Please attach manually. Thanks!