Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial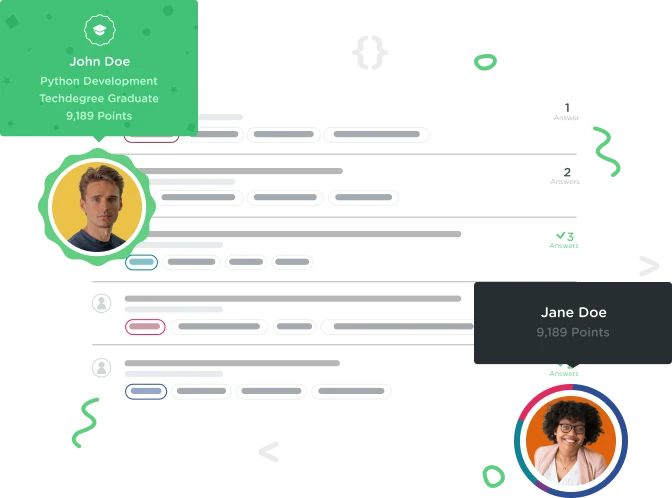
Andrew Falkowski
708 PointsRandomizer
I want to make something that displays a random string from an array. I have been out of practice and I want to start up again. I would just need some markdown and that would suffice. This will be incorporated into a website.
1 Answer
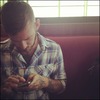
Erik McClintock
45,783 PointsAndrew,
This could be done rather easily using Math.random(), constraining it to generate a number between 0 and your array's highest index value. You could build a function that generates the number and then displays the appropriate string from your array based on the randomized index value.
Hope this helps!
Erik
Andrew Falkowski
708 PointsAndrew Falkowski
708 PointsWhat if it isn't a number I want to display, would Math.random() still work? For example the string is: ("A", "A Sharp", "B").
Erik McClintock
45,783 PointsErik McClintock
45,783 PointsAndrew,
Yes; Math.random() will generate a number which you can store in a variable, and then you pass that variable in for the index of your array.
So, for example, whereas normally if you wanted to print the second value (at index 1) of an array, you could do the following:
Using Math.random(), you would instead first generate a random number and store it in a variable, and then instead of passing 1 (or 0 or 2 or whatever) into your array's index, you would pass the variable.
You're not using Math.random() to display a number, you're simply using it to generate a random number (which is what you're looking for) to be able to tell the array "Hey, I want you to give me a random string, located at the index that Math.random() generated for me".
Does this make any more sense?
Erik
Andrew Falkowski
708 PointsAndrew Falkowski
708 PointsYes it does thanks, though I want it to display the string on the webpage for the user. Could you give me some help with that?
Erik McClintock
45,783 PointsErik McClintock
45,783 PointsAndrew,
For that, you'll want to look into DOM access and manipulation, via JavaScript methods such as getElementById(), and then altering that element's innerHTML.
Erik