Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial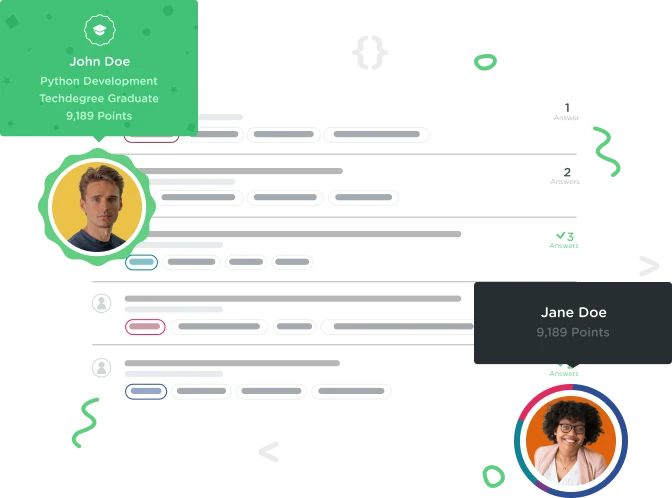

Thomas Katalenas
11,033 Pointsrandom_member function I think my answer is right!!
import random
def random_member(arg):
v = len(arg)
v = v-1
return random.choice(v)
question is:
Now, make random_member pick a random number between 0 and the length of the list, minus 1. So if the length was 5, get a number between 0 and 4.
Return the list item with that index
[MOD: added ```python markdown formatting -cf]
2 Answers
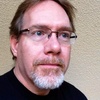
Chris Freeman
Treehouse Moderator 68,457 Pointsrandom.choice operates on a sequence (an object that has length). Using you are calling it with an int
.
Your code will pass if modified:
import random
def random_member(arg):
# v = len(arg)
# v = v-1
return random.choice(arg)
This code works, but the intent of the challenge is to use random.randint()
to create a random number and use it as an index into arg
:
import random
def random_member(arg):
# return len(arg) # Task 2
random_index = random.randint(0, len(arg))
return arg[random_index]

Thomas Katalenas
11,033 PointsThis one is off topic but python seems to be difficult to read the functions using help()
for example,
dir(random) shows a list of built in functions so I choose Random help(random.Random)
doesnt show much usefull information on how to use it so maybe I just chose one too advanced? my coments in ## just trying to figure out how would you use it....
class Random(random.Random)
Random number generator base class used by bound module functions.
|
| Used to instantiate instances of Random to get generators that don't
| share state.
|
| Class Random can also be subclassed if you want to use a different basic
| generator of your own devising: in that case, override the following
| methods: random(), seed(), getstate(), and setstate().
| Optionally, implement a getrandbits() method so that randrange()
| can cover arbitrarily large ranges.
|
| Method resolution order:
| Random
| _random.Random ## right here is syntax style on degraded obsolete modules???
| builtins.object
|
| Methods defined here:
|
| __getstate(self)
| # Issue 17489: Since __reduce_ was defined to fix #759889 this is no
| # longer called; we leave it here because it has been here since random was
| # rewritten back in 2001 and why risk breaking something.
| ## ah down here is obsolete.
| init(self, x=None)
| Initialize an instance.
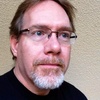
Chris Freeman
Treehouse Moderator 68,457 Pointsdir(random)
returns a list of attributes and methods from the random module:
>>> dir(random)
['BPF', 'LOG4', 'NV_MAGICCONST', 'RECIP_BPF', 'Random', 'SG_MAGICCONST',
'SystemRandom', 'TWOPI', '_BuiltinMethodType', '_MethodType', '_Sequence',
'_Set', '__all__', '__builtins__', '__cached__', '__doc__', '__file__', '__loader__',
'__name__', '__package__', '__spec__', '_acos', '_ceil', '_cos', '_e', '_exp', '_inst', '_log',
'_pi', '_random', '_sha512', '_sin', '_sqrt', '_test', '_test_generator', '_urandom',
'_warn', 'betavariate', 'choice', 'expovariate', 'gammavariate', 'gauss', 'getrandbits',
'getstate', 'lognormvariate', 'normalvariate', 'paretovariate', 'randint', 'random',
'randrange', 'sample', 'seed', 'setstate', 'shuffle', 'triangular', 'uniform',
'vonmisesvariate', 'weibullvariate']
Much of these are not intended to be used directly in your code. Typically modules will set an attribute __all__
to list the intended exposed methods:
>>> random.__all__
['Random', 'seed', 'random', 'uniform', 'randint', 'choice', 'sample',
'randrange', 'shuffle', 'normalvariate', 'lognormvariate', 'expovariate',
'vonmisesvariate', 'gammavariate', 'triangular', 'gauss', 'betavariate',
'paretovariate', 'weibullvariate', 'getstate', 'setstate', 'getrandbits',
'SystemRandom']
While Random
is on the list, it is a class used to create your own generators. Using help on random.Random
is taking you in the wrong direction.
I suggest help(random.randint)
or help(random.choice)
:
>>> help(random.randint)
Help on method randint in module random:
randint(a, b) method of random.Random instance
Return random integer in range [a, b], including both end points.
>>> help(random.choice)
Help on method choice in module random:
choice(seq) method of random.Random instance
Choose a random element from a non-empty sequence.
From help(random)
:
>>> help(random)
Help on module random:
NAME
random - Random variable generators.
MODULE REFERENCE
http://docs.python.org/3.4/library/random
The following documentation is automatically generated from the Python
source files. It may be incomplete, incorrect or include features that
are considered implementation detail and may vary between Python
implementations. When in doubt, consult the module reference at the
location listed above.
DESCRIPTION
integers
--------
uniform within range
sequences
---------
pick random element
pick random sample
generate random permutation
distributions on the real line:
------------------------------
uniform
triangular
normal (Gaussian)
lognormal
negative exponential
gamma
beta
pareto
Weibull
distributions on the circle (angles 0 to 2pi)
---------------------------------------------
circular uniform
von Mises
General notes on the underlying Mersenne Twister core generator:
* The period is 2**19937-1.
* It is one of the most extensively tested generators in existence.
* The random() method is implemented in C, executes in a single Python step,
and is, therefore, threadsafe.
CLASSES
_random.Random(builtins.object)
Random
SystemRandom
class Random(_random.Random)
| Random number generator base class used by bound module functions.
|
| Used to instantiate instances of Random to get generators that don't
| share state.
|
| Class Random can also be subclassed if you want to use a different basic
| generator of your own devising: in that case, override the following
| methods: random(), seed(), getstate(), and setstate().
| Optionally, implement a getrandbits() method so that randrange()
| can cover arbitrarily large ranges.
|
| Method resolution order:
| Random
| _random.Random
| builtins.object
|
| Methods defined here:
|
| __getstate__(self)
| # Issue 17489: Since __reduce__ was defined to fix #759889 this is no
| # longer called; we leave it here because it has been here since random was
| # rewritten back in 2001 and why risk breaking something.
|
| __init__(self, x=None)
| Initialize an instance.
|
| Optional argument x controls seeding, as for Random.seed().
|
| __reduce__(self)
|
| __setstate__(self, state)
|
| betavariate(self, alpha, beta)
| Beta distribution.
|
| Conditions on the parameters are alpha > 0 and beta > 0.
| Returned values range between 0 and 1.
|
| choice(self, seq)
| Choose a random element from a non-empty sequence.
|
| expovariate(self, lambd)
| Exponential distribution.
|
| lambd is 1.0 divided by the desired mean. It should be
| nonzero. (The parameter would be called "lambda", but that is
| a reserved word in Python.) Returned values range from 0 to
| positive infinity if lambd is positive, and from negative
| infinity to 0 if lambd is negative.
|
| gammavariate(self, alpha, beta)
| Gamma distribution. Not the gamma function!
|
| Conditions on the parameters are alpha > 0 and beta > 0.
|
| The probability distribution function is:
|
| x ** (alpha - 1) * math.exp(-x / beta)
| pdf(x) = --------------------------------------
| math.gamma(alpha) * beta ** alpha
|
| gauss(self, mu, sigma)
| Gaussian distribution.
|
| mu is the mean, and sigma is the standard deviation. This is
| slightly faster than the normalvariate() function.
|
| Not thread-safe without a lock around calls.
|
| getstate(self)
| Return internal state; can be passed to setstate() later.
|
| lognormvariate(self, mu, sigma)
| Log normal distribution.
|
| If you take the natural logarithm of this distribution, you'll get a
| normal distribution with mean mu and standard deviation sigma.
| mu can have any value, and sigma must be greater than zero.
|
| normalvariate(self, mu, sigma)
| Normal distribution.
|
| mu is the mean, and sigma is the standard deviation.
|
| paretovariate(self, alpha)
| Pareto distribution. alpha is the shape parameter.
|
| randint(self, a, b)
| Return random integer in range [a, b], including both end points.
|
| randrange(self, start, stop=None, step=1, _int=<class 'int'>)
| Choose a random item from range(start, stop[, step]).
|
| This fixes the problem with randint() which includes the
| endpoint; in Python this is usually not what you want.
|
| sample(self, population, k)
| Chooses k unique random elements from a population sequence or set.
|
| Returns a new list containing elements from the population while
| leaving the original population unchanged. The resulting list is
| in selection order so that all sub-slices will also be valid random
| samples. This allows raffle winners (the sample) to be partitioned
| into grand prize and second place winners (the subslices).
|
| Members of the population need not be hashable or unique. If the
| population contains repeats, then each occurrence is a possible
| selection in the sample.
|
| To choose a sample in a range of integers, use range as an argument.
| This is especially fast and space efficient for sampling from a
| large population: sample(range(10000000), 60)
|
| seed(self, a=None, version=2)
| Initialize internal state from hashable object.
|
| None or no argument seeds from current time or from an operating
| system specific randomness source if available.
|
| For version 2 (the default), all of the bits are used if *a* is a str,
| bytes, or bytearray. For version 1, the hash() of *a* is used instead.
|
| If *a* is an int, all bits are used.
|
| setstate(self, state)
| Restore internal state from object returned by getstate().
|
| shuffle(self, x, random=None)
| Shuffle list x in place, and return None.
|
| Optional argument random is a 0-argument function returning a
| random float in [0.0, 1.0); if it is the default None, the
| standard random.random will be used.
|
| triangular(self, low=0.0, high=1.0, mode=None)
| Triangular distribution.
|
| Continuous distribution bounded by given lower and upper limits,
| and having a given mode value in-between.
|
| http://en.wikipedia.org/wiki/Triangular_distribution
|
| uniform(self, a, b)
| Get a random number in the range [a, b) or [a, b] depending on rounding.
|
| vonmisesvariate(self, mu, kappa)
| Circular data distribution.
|
| mu is the mean angle, expressed in radians between 0 and 2*pi, and
| kappa is the concentration parameter, which must be greater than or
| equal to zero. If kappa is equal to zero, this distribution reduces
| to a uniform random angle over the range 0 to 2*pi.
|
| weibullvariate(self, alpha, beta)
| Weibull distribution.
|
| alpha is the scale parameter and beta is the shape parameter.
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| __dict__
| dictionary for instance variables (if defined)
|
| __weakref__
| list of weak references to the object (if defined)
|
| ----------------------------------------------------------------------
| Data and other attributes defined here:
|
| VERSION = 3
|
| ----------------------------------------------------------------------
| Methods inherited from _random.Random:
|
| __getattribute__(self, name, /)
| Return getattr(self, name).
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
|
| getrandbits(...)
| getrandbits(k) -> x. Generates an int with k random bits.
|
| random(...)
| random() -> x in the interval [0, 1).
class SystemRandom(Random)
| Alternate random number generator using sources provided
| by the operating system (such as /dev/urandom on Unix or
| CryptGenRandom on Windows).
|
| Not available on all systems (see os.urandom() for details).
|
| Method resolution order:
| SystemRandom
| Random
| _random.Random
| builtins.object
|
| Methods defined here:
|
| getrandbits(self, k)
| getrandbits(k) -> x. Generates an int with k random bits.
|
| getstate = _notimplemented(self, *args, **kwds)
|
| random(self)
| Get the next random number in the range [0.0, 1.0).
|
| seed(self, *args, **kwds)
| Stub method. Not used for a system random number generator.
|
| setstate = _notimplemented(self, *args, **kwds)
|
| ----------------------------------------------------------------------
| Methods inherited from Random:
|
| __getstate__(self)
| # Issue 17489: Since __reduce__ was defined to fix #759889 this is no
| # longer called; we leave it here because it has been here since random was
| # rewritten back in 2001 and why risk breaking something.
|
| __init__(self, x=None)
| Initialize an instance.
|
| Optional argument x controls seeding, as for Random.seed().
|
| __reduce__(self)
|
| __setstate__(self, state)
|
| betavariate(self, alpha, beta)
| Beta distribution.
|
| Conditions on the parameters are alpha > 0 and beta > 0.
| Returned values range between 0 and 1.
|
| choice(self, seq)
| Choose a random element from a non-empty sequence.
|
| expovariate(self, lambd)
| Exponential distribution.
|
| lambd is 1.0 divided by the desired mean. It should be
| nonzero. (The parameter would be called "lambda", but that is
| a reserved word in Python.) Returned values range from 0 to
| positive infinity if lambd is positive, and from negative
| infinity to 0 if lambd is negative.
|
| gammavariate(self, alpha, beta)
| Gamma distribution. Not the gamma function!
|
| Conditions on the parameters are alpha > 0 and beta > 0.
|
| The probability distribution function is:
|
| x ** (alpha - 1) * math.exp(-x / beta)
| pdf(x) = --------------------------------------
| math.gamma(alpha) * beta ** alpha
|
| gauss(self, mu, sigma)
| Gaussian distribution.
|
| mu is the mean, and sigma is the standard deviation. This is
| slightly faster than the normalvariate() function.
|
| Not thread-safe without a lock around calls.
|
| lognormvariate(self, mu, sigma)
| Log normal distribution.
|
| If you take the natural logarithm of this distribution, you'll get a
| normal distribution with mean mu and standard deviation sigma.
| mu can have any value, and sigma must be greater than zero.
|
| normalvariate(self, mu, sigma)
| Normal distribution.
|
| mu is the mean, and sigma is the standard deviation.
|
| paretovariate(self, alpha)
| Pareto distribution. alpha is the shape parameter.
|
| randint(self, a, b)
| Return random integer in range [a, b], including both end points.
|
| randrange(self, start, stop=None, step=1, _int=<class 'int'>)
| Choose a random item from range(start, stop[, step]).
|
| This fixes the problem with randint() which includes the
| endpoint; in Python this is usually not what you want.
|
| sample(self, population, k)
| Chooses k unique random elements from a population sequence or set.
|
| Returns a new list containing elements from the population while
| leaving the original population unchanged. The resulting list is
| in selection order so that all sub-slices will also be valid random
| samples. This allows raffle winners (the sample) to be partitioned
| into grand prize and second place winners (the subslices).
|
| Members of the population need not be hashable or unique. If the
| population contains repeats, then each occurrence is a possible
| selection in the sample.
|
| To choose a sample in a range of integers, use range as an argument.
| This is especially fast and space efficient for sampling from a
| large population: sample(range(10000000), 60)
|
| shuffle(self, x, random=None)
| Shuffle list x in place, and return None.
|
| Optional argument random is a 0-argument function returning a
| random float in [0.0, 1.0); if it is the default None, the
| standard random.random will be used.
|
| triangular(self, low=0.0, high=1.0, mode=None)
| Triangular distribution.
|
| Continuous distribution bounded by given lower and upper limits,
| and having a given mode value in-between.
|
| http://en.wikipedia.org/wiki/Triangular_distribution
|
| uniform(self, a, b)
| Get a random number in the range [a, b) or [a, b] depending on rounding.
|
| vonmisesvariate(self, mu, kappa)
| Circular data distribution.
|
| mu is the mean angle, expressed in radians between 0 and 2*pi, and
| kappa is the concentration parameter, which must be greater than or
| equal to zero. If kappa is equal to zero, this distribution reduces
| to a uniform random angle over the range 0 to 2*pi.
|
| weibullvariate(self, alpha, beta)
| Weibull distribution.
|
| alpha is the scale parameter and beta is the shape parameter.
|
| ----------------------------------------------------------------------
| Data descriptors inherited from Random:
|
| __dict__
| dictionary for instance variables (if defined)
|
| __weakref__
| list of weak references to the object (if defined)
|
| ----------------------------------------------------------------------
| Data and other attributes inherited from Random:
|
| VERSION = 3
|
| ----------------------------------------------------------------------
| Methods inherited from _random.Random:
|
| __getattribute__(self, name, /)
| Return getattr(self, name).
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
FUNCTIONS
betavariate(alpha, beta) method of Random instance
Beta distribution.
Conditions on the parameters are alpha > 0 and beta > 0.
Returned values range between 0 and 1.
choice(seq) method of Random instance
Choose a random element from a non-empty sequence.
expovariate(lambd) method of Random instance
Exponential distribution.
lambd is 1.0 divided by the desired mean. It should be
nonzero. (The parameter would be called "lambda", but that is
a reserved word in Python.) Returned values range from 0 to
positive infinity if lambd is positive, and from negative
infinity to 0 if lambd is negative.
gammavariate(alpha, beta) method of Random instance
Gamma distribution. Not the gamma function!
Conditions on the parameters are alpha > 0 and beta > 0.
The probability distribution function is:
x ** (alpha - 1) * math.exp(-x / beta)
pdf(x) = --------------------------------------
math.gamma(alpha) * beta ** alpha
gauss(mu, sigma) method of Random instance
Gaussian distribution.
mu is the mean, and sigma is the standard deviation. This is
slightly faster than the normalvariate() function.
Not thread-safe without a lock around calls.
getrandbits(...) method of Random instance
getrandbits(k) -> x. Generates an int with k random bits.
getstate() method of Random instance
Return internal state; can be passed to setstate() later.
lognormvariate(mu, sigma) method of Random instance
Log normal distribution.
If you take the natural logarithm of this distribution, you'll get a
normal distribution with mean mu and standard deviation sigma.
mu can have any value, and sigma must be greater than zero.
normalvariate(mu, sigma) method of Random instance
Normal distribution.
mu is the mean, and sigma is the standard deviation.
paretovariate(alpha) method of Random instance
Pareto distribution. alpha is the shape parameter.
randint(a, b) method of Random instance
Return random integer in range [a, b], including both end points.
random(...) method of Random instance
random() -> x in the interval [0, 1).
randrange(start, stop=None, step=1, _int=<class 'int'>) method of Random instance
Choose a random item from range(start, stop[, step]).
This fixes the problem with randint() which includes the
endpoint; in Python this is usually not what you want.
sample(population, k) method of Random instance
Chooses k unique random elements from a population sequence or set.
Returns a new list containing elements from the population while
leaving the original population unchanged. The resulting list is
in selection order so that all sub-slices will also be valid random
samples. This allows raffle winners (the sample) to be partitioned
into grand prize and second place winners (the subslices).
Members of the population need not be hashable or unique. If the
population contains repeats, then each occurrence is a possible
selection in the sample.
To choose a sample in a range of integers, use range as an argument.
This is especially fast and space efficient for sampling from a
large population: sample(range(10000000), 60)
seed(a=None, version=2) method of Random instance
Initialize internal state from hashable object.
None or no argument seeds from current time or from an operating
system specific randomness source if available.
For version 2 (the default), all of the bits are used if *a* is a str,
bytes, or bytearray. For version 1, the hash() of *a* is used instead.
If *a* is an int, all bits are used.
setstate(state) method of Random instance
Restore internal state from object returned by getstate().
shuffle(x, random=None) method of Random instance
Shuffle list x in place, and return None.
Optional argument random is a 0-argument function returning a
random float in [0.0, 1.0); if it is the default None, the
standard random.random will be used.
triangular(low=0.0, high=1.0, mode=None) method of Random instance
Triangular distribution.
Continuous distribution bounded by given lower and upper limits,
and having a given mode value in-between.
http://en.wikipedia.org/wiki/Triangular_distribution
uniform(a, b) method of Random instance
Get a random number in the range [a, b) or [a, b] depending on rounding.
vonmisesvariate(mu, kappa) method of Random instance
Circular data distribution.
mu is the mean angle, expressed in radians between 0 and 2*pi, and
kappa is the concentration parameter, which must be greater than or
equal to zero. If kappa is equal to zero, this distribution reduces
to a uniform random angle over the range 0 to 2*pi.
weibullvariate(alpha, beta) method of Random instance
Weibull distribution.
alpha is the scale parameter and beta is the shape parameter.
DATA
__all__ = ['Random', 'seed', 'random', 'uniform', 'randint', 'choice',...
FILE
/usr/lib/python3.4/random.py
Thomas Katalenas
11,033 PointsThomas Katalenas
11,033 Pointsah yes, I didn't read the directions right the last line said Return the list item with that index so that means I take a random number and then use that to index back into the list
so
and it is correct!!
Very nice directions! geez I can't believe missed it first time. welp gotta go CYA