Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial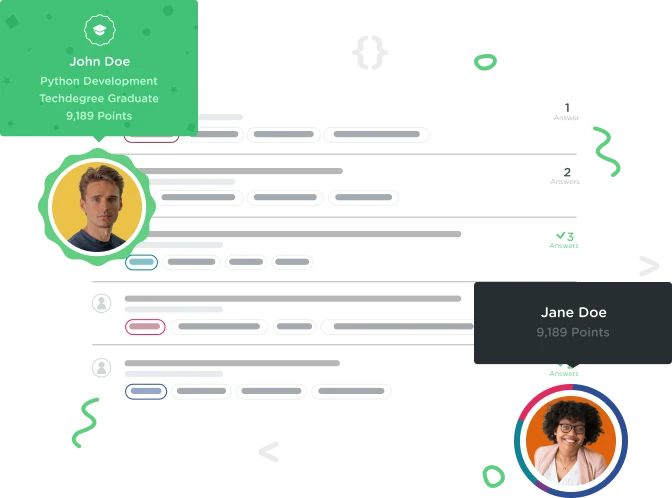

Brian York
6,213 Pointsrandom.randint and index
This challenge seems easy, but I cannot figure it out. Can somebody tell me what I am doing wrong?
Here is the challenge prompt: "You've seen how random.choice() works. It gets a random member from an iterable (like a list or a string). I want you to try and reproduce it yourself. First, import the random library. Then create a function named random_item that takes a single argument, an iterable. Then use random.randint() to get a random number between 0 and the length of the iterable, minus one. Return the iterable member that's at your random number's index. Check the file for an example."
Thanks in advance.
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
import random
def random_item("Argument"):
a = random.randint(0, 7)
return "Argument"[a]
3 Answers
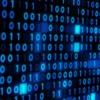
Alexander Davison
65,469 PointsThere are two major in your code:
- You can't use quotes for variable (or parameter) names. You use quotes only for strings.
- You code will only work if the length of the list is length of 7. You need to actually find the length of the function by using the
len
function. (Make sure to subtract the value oflen
by 1 because computers start counting at zero!)
If these hints don't help enough, please reply and I'll provide more. Before you do that, please at least try! Writing code on your own is the best way to learn coding. Also, when you reply, please provide the new code snippet you tried after reading this. Thanks!
I hope this helps. ~Alex

Khaled Zahran
Courses Plus Student 1,944 PointsYou can not use " " in a def unless you are assigning. You do not have to print but it is useful for testing as I have done below then you can remove. Also you want a to go the length of iterable - 1. Hope this helps.
import random
def random_item(iterable = "treehouse"): a = random.randint(0, len(iterable)-1) print(a) print(iterable[a]) return iterable[a]
random_item("treehouse")

Brian York
6,213 PointsThank you both. I got tripped up, because the example in line 2 is """
random_item("Treehouse")
""" but that isn't defining the function, it is, I assume, showing it being called. Thus, "Treehouse" is the argument not the name of an argument. I also changed """ a = random.randint(0, 7) """ to """ a = random.randint(0, len(argument) - 1) """
Thanks again!
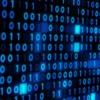
Alexander Davison
65,469 PointsCan you give either of us a "Best Answer" so that your question is marked as answered? Thank you. ~Alex