Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial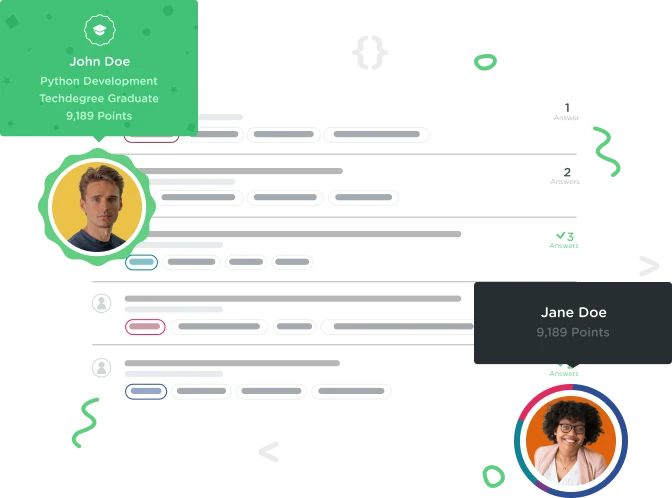
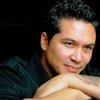
Chuck Robertson
3,408 PointsRather than using prompt, how can we collect input from a user via a form in the HTML...then display results and score?
I want to build some very simple games. Quizzes will work...how do I build a function that evaluates user input from an HTML form against a set value? I would need a mixture of different form controls like radio buttons for yes or no responses, input fields, and check boxes.
2 Answers
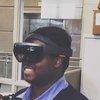
Guled Ahmed
12,806 PointsBuilding games with prompt can be quite boring, but I got a solution for you that might spice things up with forms. Here's a simple little quiz game I built as an example for you. The game is simple just complete the word given to you. First I start with the form:
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Simple Quiz Game</title>
</head>
<body>
<form action="
">
<label for="name">Finish the word: Appl</label>
<br>
<input type="text" id="question1">
<button onclick="submitInfo()">Submit</button>
</form>
<script src="myscript.js"></script>
</body>
</html>
Notice that the input tag has an id of "question1" I'll be targeting that element with Javascripts document.getElementById method which targets elements in the DOM. And also notice the onclick event object binded to the button element. This is used to call a the function submitInfo within our script containing the code for the function.
myscript.js
function submitInfo(){
var question1 = document.getElementById("question1").value;
if(question1 == "Apple"){
confirm("You got it!");
}
}
Above is the Javascript file in which contains my code. Here I placed my submitInfo function which is activated by the button element in my HTML file. As explained earlier, I attached the event object onclick="function call goes here" to the ** button** tag in order to allow the function to be called once the button is clicked.
Now lets discuss the Javascript code. I store the users value in the variable question1. The way I obtain the value from the input tag is to target the input tag with the document.getElementById function, and within the function I specify that I want to target the question1 id associated with the input tag. Once targeted I use the value property in Javascript to obtain the text value placed within the input tag. I then use an if statement to evaluate the variable to see if the user got the right answer. I hope this gave you some inspiration on making games without the use of prompts.
Have a good day. :)

Jason Reitsch
4,698 PointsCool. Just curious why you included it and left that extra long blank space. :) Thanks!
Chuck Robertson
3,408 PointsChuck Robertson
3,408 PointsThis is great! Thanks so much! What I am hoping to see is an example of each input type. For example: a radio button (possibly a boolean "TRUE" or "FALSE" ), a check box, a text field, and a drop down box for multiple options. I want to build a game that trains the user in something like a skill set. I have seen examples of these separately but having trouble putting them all together and outputing a score. Also would like to output that score directly to a div instead of a text area. Any further guidance is much appreciated!
Guled Ahmed
12,806 PointsGuled Ahmed
12,806 PointsHello Chuck, I made a little game with all the specifications you've mentioned. I hope this helps. I also left links that will help in terms of how I extracted the values from each input tag:
Math Quiz Game (with code)
W3School Tutorials
Jason Reitsch
4,698 PointsJason Reitsch
4,698 PointsWhy did you include the action attribute in the form element and enclose a long empty space in quotes like that? Just curious if there's a reason for that.
Guled Ahmed
12,806 PointsGuled Ahmed
12,806 PointsHello Jason, the form action tag is used to specify where to send form data when the user submits the data using a submit button. I didn't need to send data back to a file so I left it blank. I could have removed it totally, but I'm use to typing everything out. There is more information on this here.