Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial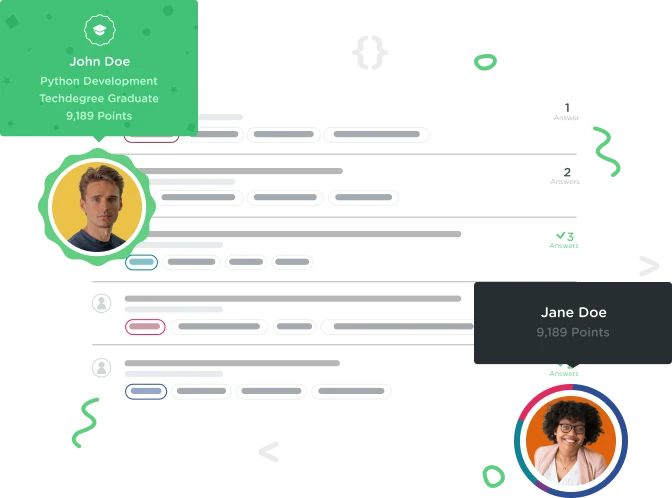

Ted Clayton
Courses Plus Student 206 PointsReached end of file while parsing?
Can someone look at my code and see why it is failing
import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here!
*/
//__Name__ is a __adjective__ __noun__. They are always __adverb__ __verb__.
String ageAsString = console.readLine("How old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
//Insert exit code
console.printf("Sorry you must be at least 13 to use this program.\n");
System.exit(0);
}
String name = console.readLine("Enter a name: ");
String adjective = console.readLine("Enter an adjective: ");
String noun;
boolean isInvalidWord;
do {
noun = console.readLine("Enter a noun: ");
isInvalidWord = (noun.equalsIgnoreCase("dork") ||
noun.equalsIgnoreCase("jerk");
if (isInvalidWord) {
noun.equalsIgnoreCase("moneychaser")){
console.printf("That God Dern language isn't allowed. Try Again . \n\n");
}
} while(isInvalidWord);
String adverb = console.readLine("Enter an adjective: ");
String verb = console.readLine("Enter a verb ending in -ing: ");
console.printf("Your TreeStory:\n--------------\n");
console.printf("%s is a %s %s. ", name, adjective, noun);
if (noun.equals("dork")) {
console.printf("That God Dern language isn't allowed. Exiting. \n\n");
System.exit(0);
}
console.printf("They are always %s %s. \n", adverb, verb);
}
}
Moderator Edit: Added Markdown to the post so the code will be properly formatted and readable in the Community. When posting code, please refer the the Markdown Cheatsheet for proper markdown to be used.
1 Answer
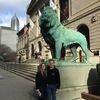
Matthew Beiswenger
6,814 PointsOkay so I'm going to start off by saying that you should try to format your code better. I know that the moderator edited the markdown so I don't know what it looked like before, but the I think the formatting and organization is the main issue. I reformatted your code for you to look like this:
import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here!
*/
//__Name__ is a __adjective__ __noun__. They are always __adverb__ __verb__.
String ageAsString = console.readLine("How old are you?");
int age = Integer.parseInt(ageAsString);
if (age < 13)
{
//Insert exit code
console.printf("Sorry you must be at least 13 to use this program.\n");
System.exit(0);
}
String name = console.readLine("Enter a name: ");
String adjective = console.readLine("Enter an adjective: ");
String noun;
boolean isInvalidWord;
do
{
noun = console.readLine("Enter a noun: ");
isInvalidWord = (noun.equalsIgnoreCase("dork") || noun.equalsIgnoreCase("jerk");
if (isInvalidWord)
{
noun.equalsIgnoreCase("moneychaser")){
console.printf("That God Dern language isn't allowed. Try Again . \n\n");
}
}
while(isInvalidWord);
String adverb = console.readLine("Enter an adjective: ");
String verb = console.readLine("Enter a verb ending in -ing: ");
console.printf("Your TreeStory:\n--------------\n");
console.printf("%s is a %s %s. ", name, adjective, noun);
if (noun.equals("dork"))
{
console.printf("That God Dern language isn't allowed. Exiting. \n\n");
System.exit(0);
}
console.printf("They are always %s %s. \n", adverb, verb);
}
}
Some of the issues include missing an outer parenthesis:
isInvalidWord = (noun.equalsIgnoreCase("dork") || noun.equalsIgnoreCase("jerk");
And adding unnecessary brackets, parenthesis, and not having a semicolon:
noun.equalsIgnoreCase("moneychaser")){
And you also having an extra bracket at the end of the file. I would fix these issues first and then ask another question if you still run into problems.