Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial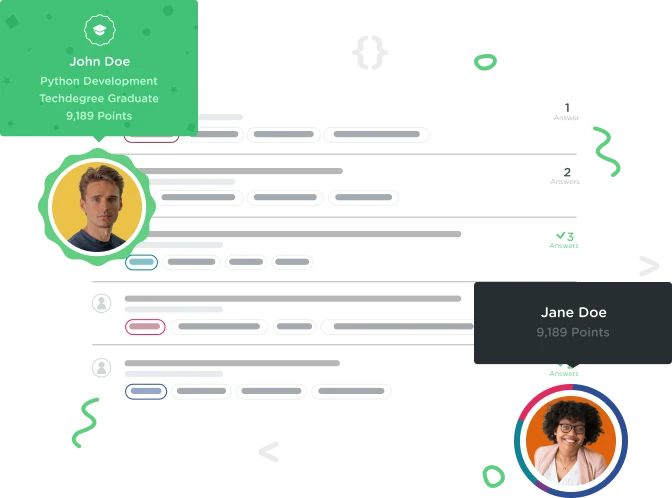
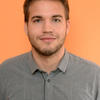
Adam Beer
11,314 PointsReact
Hi everybody! I have a problem/question.
How can I find N/A from the response? If response poster equal to N/A then load this picture: ../img/imgnotfound.jpg. Second if, the released or the plot equal to N/A then don't give anything back. Any idea how can I solve this problem? I tried use the onError img property, but it doesn't working for me. I copied my code and I make a screenshots about console response.
App.js
import React from 'react';
import Form from './components/Form';
import Movie from './components/Movie';
import './App.css';
const API_KEY = '9606ae0f';
class App extends React.Component {
state = {
all: [],
error: '',
search: ''
}
getMovies = (e) => {
e.preventDefault();
const movie_id = e.target.elements.movie_id.value;
const url = `http://www.omdbapi.com/?s=${movie_id}&apikey=${API_KEY}`;
fetch(url)
.then((res) => res.json())
.then((res) => res.Search
.map((item) => item.imdbID)
.map((id) => fetch(`http://www.omdbapi.com/?i=${id}&apikey=${API_KEY}`)))
.then((promises) => Promise.all(promises))
.then((responses) => Promise.all(responses.map((res) => res.json())))
.then(
(res) =>
this.setState({
all: res,
error: '',
})
)
.catch(
(err) => {
this.setState({
all: [],
search: movie_id,
error: 'This title is invalid, please try other title'
})
});
}
render() {
return(
<div className="content">
<Form getMovies={this.getMovies} />
<Movie
all={this.state.all}
error={this.state.error}
search={this.state.search}
/>
</div>
);
}
};
export default App;
Movie.js
import React from 'react';
import './Movie.css';
class Movie extends React.Component{
render() {
return(
<div className="container">
<div className="container_two">
{ this.props.error &&
<span className="error">
<p className="search"> { this.props.search } </p>
{ this.props.error }
</span>
}
{ this.props.all.map((param, i) => {
return <table key={i}>
<tbody key={i}>
<tr className="first" key={i}>
<td key={i}>
<div className="poster">
<a href={`http://www.imdb.com/title/${param.imdbID}`} >
<img src={param.Poster} ref={img => this.img = img} alt={param.Title} onError={() => this.img.src = '../img/imgnotfound.jpg'}/>
</a>
</div>
</td>
<td className="second">
<div>
<h4 className="title">
<a href={`http://www.imdb.com/title/${param.imdbID}`} >
{ param.Title }
</a>
</h4>
</div>
<div className="released">
<span className="released_date" >
{ param.Released && <span >Released:
<span className="released_time">{ param.Released }</span>
</span>
}
</span>
</div>
<div className="plot">
{ param.Plot }
</div>
<div className="author_box">
{ param.Director &&
<span className="author" >Director:
<span className="author_name">{ param.Director }</span>
</span>
}
</div>
<div className="actors_box">
{ param.Actors &&
<span className="actors">Actors:
<span className="actors_name">{ param.Actors }</span>
</span>
}
</div>
</td>
</tr>
</tbody>
</table>
})}
</div>
</div>
);
}
}
export default Movie;
Thank you for sharing idea. Have a nice day!
3 Answers

Zack Jackson
30,220 PointsWouldn't this work?
<img src={param.Poster === 'N/A' ? "../img/imgnotfound.jpg" : param.Poster}>
You should be able to break each piece out into components and then inject them in Movie.js. Nothing wrong with doing it all in one file and then refactoring though.
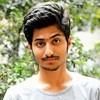
Saud Tauqeer
Courses Plus Student 8,099 PointsI cannot give you an answer on this as i'm still learning react myself. But try stackoverflow too.
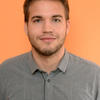
Adam Beer
11,314 PointsThanks anyway! Good learning! :)

Zack Jackson
30,220 PointsDoes the API give you a poster response if there is no poster? What do you get back from the response object? I'm guessing it's res.poster and that poster has the image url. You could first check if the .poster exists in the object with a conditional and then force display the N/A image url if it doesn't exist in the response. You kind of did something similar with the others (released, directors, actors) with the &&. Might be more concise in this case to throw a ternary into your src tag javascript code.
Also, I'd probably break down the movie class into subcomponents. There is a lot going on here for just one class.
Pretty cool project here. I might try it out for myself as a fun side project to practice. Thanks for the inspiration.
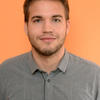
Adam Beer
11,314 PointsIf there is no poster the API response for me the Poster: N/A. If there is no released date the API response for me the Released: N/A and so on. So I want to manage the "N/A" value in the response. So Poster: N/A then load this picture: ../img/imgnotfound.jpg or if the released or the plot value equal to N/A then don't give anything back. I'm comfused now :D So I try the if statement inside then() and I get 3 true, somehow I need to use the answer but I don't know how. I think tomorrow I rewrite my whole code logic, because a don't save step by step the properties which I use, I'm just saved the whole response object and selecting properties inside Movie.js which I want to see. Now I think how I selected the bad solution for this problem. I don't know because I'm comfused.
Adam Beer
11,314 PointsAdam Beer
11,314 PointsOhhh man, thank you very match! :) I added the require() to your idea and now its working fine!
<img src={param.Poster === 'N/A' ? require("../img/imgnotfound.jpg") : param.Poster} alt={param.Title} />
I thought these logics are only running in jsx. I learned a lot from this project!
Screenshot of the project
Now this is not nice but working.I should to manage the other "N/A" values in the response and then I'll finish the project. If you made this project please upload the github repository and tag my name. I'm very interested how solve this project with other logic or aspect.
Have a nice day and thank you again! :)
Adam Beer
11,314 PointsAdam Beer
11,314 Pointshttps://teamtreehouse.com/community/i-finished-my-react-omdbapi-call-code