Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial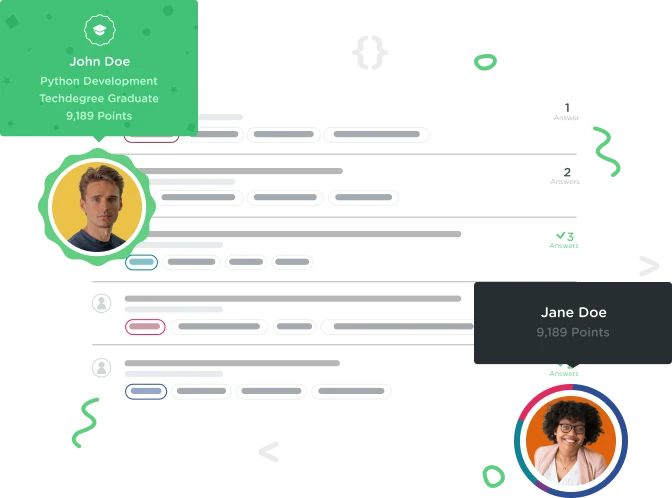

Laurence kite
11,768 PointsReact and slice etcn
I am confused as to why the state is being updated when I am making copy of the state prior to using the map array method which is not meant to change the original array either but the app reflects it is being changed by the code in the function FindHighScore?..
Here is the code:
import React, {Component} from 'react';
import Player from './Player';
import Header from './Header';
import AddPlayerForm from './AddPlayerForm';
class App extends Component {
state = {
players: [
{
name: "Guil",
score: 0,
id: 1,
isHigh: false
},
{
name: "Treasure",
score: 0,
id: 2,
isHigh: false
},
{
name: "Ashley",
score: 0,
id: 3,
isHigh: false
},
{
name: "James",
score: 0,
id: 4,
isHigh: false
}
]
};
// player id counter
prevPlayerId = 4;
handleScoreChange = (index, delta) => {
this.setState( (prevState) => ({
score: prevState.players[index].score += delta
}));
}
FindHighScore = () => {
const copyPlayers = this.state.players.slice(0);
// copy of state into copyPlayers by slice so that it is not changed..?
console.log(copyPlayers);
const maxScore = copyPlayers.reduce((total, player) =>{
//console.log(player.score);
if (total > player.score) {
return total;
} else {
return player.score;
}
}, 0)
console.log(maxScore);
console.log(copyPlayers);
let highest = copyPlayers.map((ply)=>{
if (ply.score === maxScore) {
ply.isHigh = true;
return ply;
} else {
ply.isHigh = false;
return ply;
}
})
console.log(highest);
}
handleAddPlayer = (name) => {
this.setState( (prevState)=> {
return {
players: [
...prevState.players,
{
name: name,
score: 0,
id: this.prevPlayerId += 1
}
]
}
})
}
handleRemovePlayer = (id) => {
this.setState( prevState => {
return {
players: prevState.players.filter((p) => p.id !== id)
};
});
}
render() {
//props = {tile: "Scoreboard", Players: [{name: "Guil", scoe: 0, id: 0}]}
this.FindHighScore();
return (
<div className="scoreboard">
<Header players={this.state.players} />
{/* Players list */}
{this.state.players.map( (player, index) =>
<Player
name={player.name}
score = {player.score}
id={player.id}
key={player.id.toString()}
index = {index}
changeScore = {this.handleScoreChange}
removePlayer={this.handleRemovePlayer}
highScore ={player.isHigh}
/>
)}
<AddPlayerForm addPlayer ={this.handleAddPlayer}/>
</div>
);
}
}
export default App;
// update the new plyers array via concat:
//called when handleaddplayer is used
/*handleAddPlayer = (name) => {
let newPlayer = {
name,
score: 0,
id: this.prevPlayerId += 1
};
this.setState( prevState => ({
players: prevState.players.concat(newPlayer)
}));
}
*/
//Note on React uni diretional: React flows any data changes at the top down through
// the component tree, updating each component.