Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial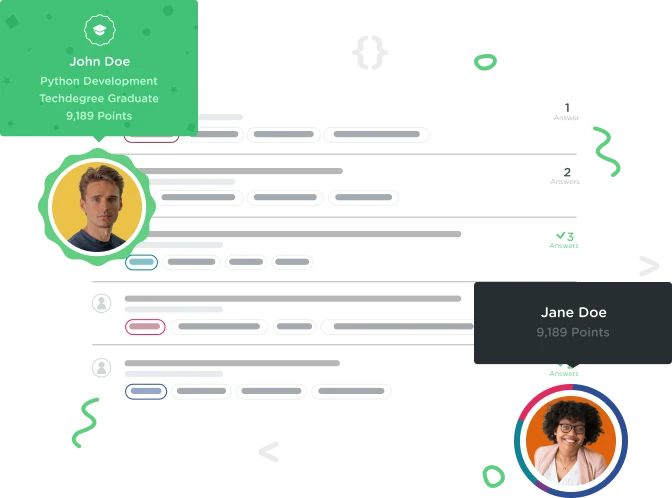
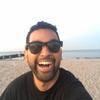
Anthony Albertorio
22,624 PointsReact Docs now recommends using function with prevState inside of setState
From what I understand, React recommends using a function inside of setState that takes in prevState and an optional props parameter.
According to the React docs "React may batch multiple setState() calls into a single update for performance. Because this.props and this.state may be updated asynchronously, you should not rely on their values for calculating the next state."
"To fix it, use a second form of setState() that accepts a function rather than an object. That function will receive the previous state as the first argument, and the props at the time the update is applied as the second argument:"
// Wrong
this.setState({
counter: this.state.counter + this.props.increment,
});
// es6 way
// Correct
this.setState((prevState, props) => ({
counter: prevState.counter + props.increment
}));
// es5 way
// Correct
this.setState(function(prevState, props) {
return {
counter: prevState.counter + props.increment
};
});
See the React docs for more information: https://reactjs.org/docs/state-and-lifecycle.html
Also, see this great post: https://medium.com/@shopsifter/using-a-function-in-setstate-instead-of-an-object-1f5cfd6e55d1
A better way could be by just updating the new state like this:
class App extends Component {
//...
newGuestSubmitHandler = e => {
e.preventDefault();
if (this.state.pendingGuest !== "") {
const newPerson = {
name: this.state.pendingGuest,
isConfirmed: false,
isEditing: false
};
this.setState(prevState => ({
guests: [newPerson, ...prevState.guests],
pendingGuest: ""
}));
}
}
//...
}
2 Answers

jay aljoe
4,113 Pointsnice to see other ways of doing stuff or thinking.
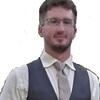
Sean Paulson
6,669 PointsNow they recommend using hooks in react. Much easier. Ill post my solution using hooks when I finish if anyone is interested.
Young Bae
18,429 PointsYoung Bae
18,429 PointsGood to know! It helps me a lot :) Thank you for posting.