Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial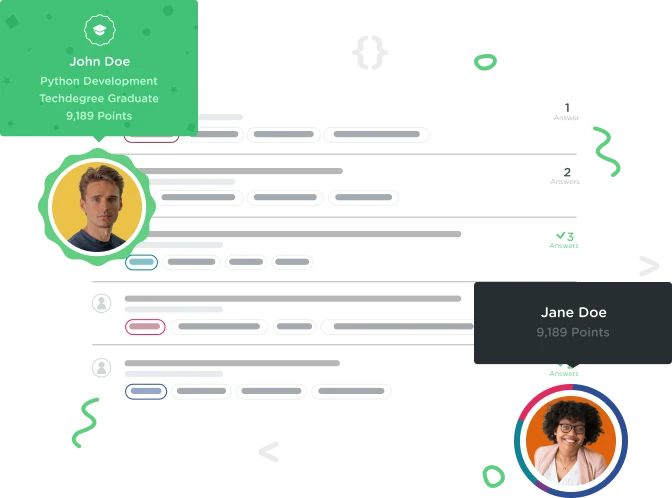
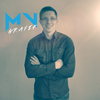
Jakub Kašpar
16,383 PointsReact - How to pass value to filter function in component?
Hello everyone,
I am trying to build a simple to-do app.
I store all my tasks in an array like this:
var TASKS = [
{
name: "Pay Bills",
completed: false,
id: 1,
},
{
name: "Go shopping",
completed: false,
id: 2,
},
{
name: "Pick up something",
completed: false,
id: 3,
},
{
name: "Drink Coffee",
completed: true,
id: 4,
},
];
and I want to list them in separated sections (todo/done) by passing boolean value as props to the listing component.
My component structure is here:
var Application = React.createClass({
propTypes: {
title: React.PropTypes.string,
},
getDefaultProps: function() {
return {
title: "Add item",
}
},
onTodoAdd: function(name) {
...
},
onRemoveTask: function(index) {
...
},
onCompletedTask: function(index) {
...
},
render: function() {
return (
<div className="to-do">
<Section title={this.props.title}>
<AddTodoForm onAdd={this.onTodoAdd} />
</Section>
<Section title="to-do">
<ListTasks initialTasks={TASKS} completedTasks={false} />
</Section>
<Section title="done">
<ListTasks initialTasks={TASKS} completedTasks={true} />
</Section>
</div>
);
}
});
and the listing component looks like this:
var ListTasks = React.createClass({
propTypes: {
initialTasks: React.PropTypes.array.isRequired,
completedTasks: React.PropTypes.bool.isRequired,
},
getInitialState: function() {
return {
tasks: this.props.initialTasks,
};
},
render: function() {
return (
<div>
{this.state.tasks
.filter(function(task, index, props) {
return task.completed === props.completedTasks;
})
.map(function(task, index) {
return(
<Task
name={task.name}
key={task.id}
completed={task.completed}
onCompleted={function() {this.onCompletedTask(index)}.bind(this)}
onRemove={function() {this.onRemoveTask(index)}.bind(this)}
/>
);
}.bind(this))}
</div>
);
}
});
but I canť pass the
completedTasks={true/false}
to the filter function with
props.completedTasks
is there anyone who can help please?
Thanks a lot.