Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial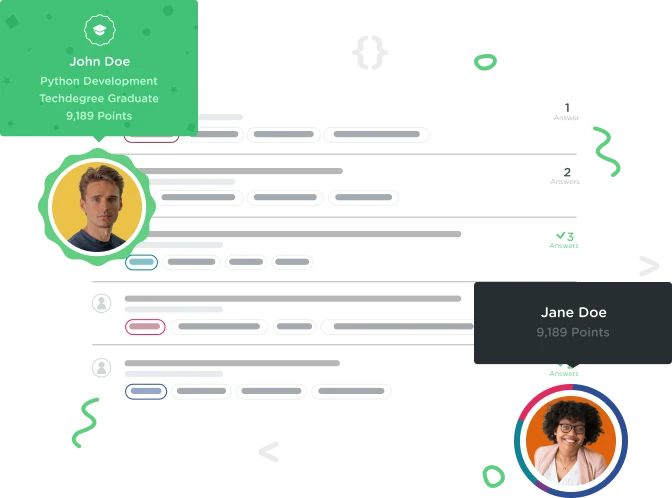

Mike Lundahl
13,510 PointsReact not running
Can't seem to get React to run! I followed your React basic course but then had to take a few steps back to learn about webpack and babel. Took a while to understand it, but eventually got it up and running. But still React wont work and I can't figure out why?
Here's my index.html
<DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Main</title>
</head>
<body>
<div id="container">Loading...</div>
<script type="text/babel" src="./test.jsx"></script>
</body>
</html>
Here's the test.jsx:
console.log('Test is loading!');
function Application() {
return (
<div>
<h1>Hello, testing React!</h1>
<p>I was rendered from the Application component!</p>
</div>
);
}
ReactDOM.render(<Application />, document.getElementById('container'));
console.log('Test loaded!');
Here's the webpack config
var nodeExternals = require('webpack-node-externals');
module.exports = {
entry: './app.js' ,
target: 'node',
externals: [nodeExternals()],
output: {
path: 'build',
filename: 'bundle.js'
},
module: {
loaders: [
{
loaders: ['react-hot', 'babel-loader'],
test: /\.jsx?$/,
exclude: /node_modules/
}
]
},
resolve: {
extensions: ['', '.js', '.jsx'],
}
};
And also the package.js file
{
"name": "test",
"version": "1.0.0",
"description": "",
"main": "app.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"build": "webpack",
"start": "webpack-dev-server --progess --inline --hot"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"bcryptjs": "^2.4.0",
"body-parser": "^1.15.2",
"express": "^4.14.0",
"mongodb": "^2.2.15",
"mongoose": "^4.7.3",
"react": "^15.4.1",
"react-dom": "^15.4.1"
},
"devDependencies": {
"babel-core": "^6.21.0",
"babel-loader": "^6.2.10",
"babel-preset-es2015": "^6.18.0",
"babel-preset-react": "^6.16.0",
"react-hot-loader": "^1.3.1",
"webpack": "^1.14.0",
"webpack-dev-server": "^1.16.2",
"webpack-node-externals": "^1.5.4"
}
}
the babelrc file
{
"presets": ["react", "es2015"]
}
Also I might add that
import React from 'react';
import ReactDOM from 'react-dom';
are in the app.js file.
Seems like the test.jsx isn't even loading.. is this babel not working correctly? Any ideas?
1 Answer

Seth Kroger
56,413 PointsYou're close to the answer. webpack will use the file listed under "entry" as the starting point of the script. You can change it to test.jsx if you like. It will still bundle up everything to the output, specified specified here as bundle.js. The important thing to realize is that it won't change the text.jsx file at all, the compiled result is included in bundle.js, and it's the bundle.js file that needs to be included in index.html, not test.jsx. Also you do need to include React and ReactDOM if you want to use it in the file, regardless.

Mike Lundahl
13,510 PointsThanks Seth!
I'm running webpack through
"start": "webpack-dev-server --progess --inline --hot"
tried specifying the bundle.js file in the index.html. Still can't get it to work for some reason.. It doesn't get passed the index.html with the "loading..." text on the screen.

Seth Kroger
56,413 PointsI'd check the DevTools console for any error messages. That's the first place I'd check if the app wasn't working.

Mike Lundahl
13,510 PointsYeah. I'm checking Chrome's console and the only thing that it puts out is
"Fetching scripts with an invalid type/language attributes is deprecated and will be removed in M56, around January 2017. See https://www.chromestatus.com/features/5760718284521472 for more details."
nothing else.

Mike Lundahl
13,510 PointsFeel like I've tried everything! Also tried creating a build and that doesn't work either.
after I created a build and start server by the regular way "node app" which sends the index.html, it for some reason doesn't find the bundle.js file... I've tried building it to a build directory as well as directly to the root. but it doesn't seem to matter.
Am I perhaps missing some bable/webpack loaders/plugins?

Seth Kroger
56,413 PointsDoes your script tag in index.html still have type="text/babel
? bundle.js should be plain JavaScript after webpack is through with it.

Mike Lundahl
13,510 PointsYeah... type="text/javascript" was used when I switched over to the bundle.js
Mike Lundahl
13,510 PointsMike Lundahl
13,510 Pointshmm.. might be a dumb question (still learning )... do webpack / babel only transpile files sourced from the entry file specified (app.js in my case)? Since my jsx test file is only sourced in the index.html file which i turn is the one the app.js server sends to the browser. Might this be the problem?