Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial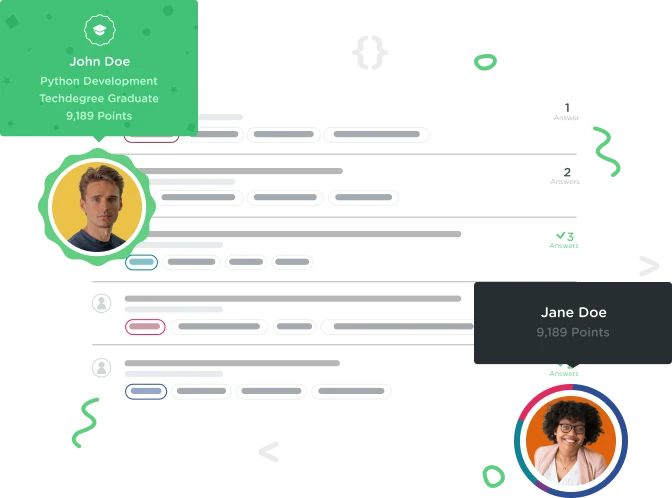

Laurence kite
11,768 Pointsreact video creating animations in react
I am confused by this code, shown below:
// Libs
import React, { Component } from 'react';
import { render } from 'react-dom';
// CSS
import './css/style.css';
// Component
class GuestList extends Component {
constructor() {
super(...arguments);
this.state = {
names: [
{ name: 'Joel' },
{ name: 'Alena' },
{ name: 'Andrew' },
{ name: 'Thera' }
]
}
}
handleChange(event) {
console.log(this.state.newName);
if(event.key == 'Enter') {
//once enter is pressed
let newName = { name: event.target.value }
let newNames = this.state.names.concat(newName);
// newName is added to array names
event.target.value = '';
this.setState({ names: newNames });
}
}
handleRemove(i) {
var newNames = this.state.names;
newNames.splice(i, 1);
this.setState({ names: newNames });
}
render() {
let guests = this.state.names.map((name, i) => (
<li key={name.name}>
{name.name}
<button onClick={this.handleRemove.bind(this, i)}>Remove</button>
</li>
));
return (
<div className="guest-list">
<h1>Guest List</h1>
<input type="text" placeholder="Invite Someone" value={this.state.newName} onKeyDown={this.handleChange.bind(this)} />
<ul>
{guests}
</ul>
</div>
);
}
}
render(
<GuestList />,
document.getElementById('root')
);
In particular this line which renders and input type of text here it says value = {this.state.newName}
I logged this out to the console and as I thought it is undefined
<input type="text" placeholder="Invite Someone" value={this.state.newName} onKeyDown={this.handleChange.bind(this)} />
I log it out in the handleChange function which at this point with no key being pressed has not even been set as a variable.
So why does not the text input box display undefined
As you type in the text box it remains undefined as I expect. In fact this.state.newName will never exist. this.sate will point to the names array.
2 Answers

Colton Ehrman
255 PointsI believe that is just how React works, if a variable is undefined it just doesn't render anything.

Laurence kite
11,768 PointsOk I was not aware thanks