Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial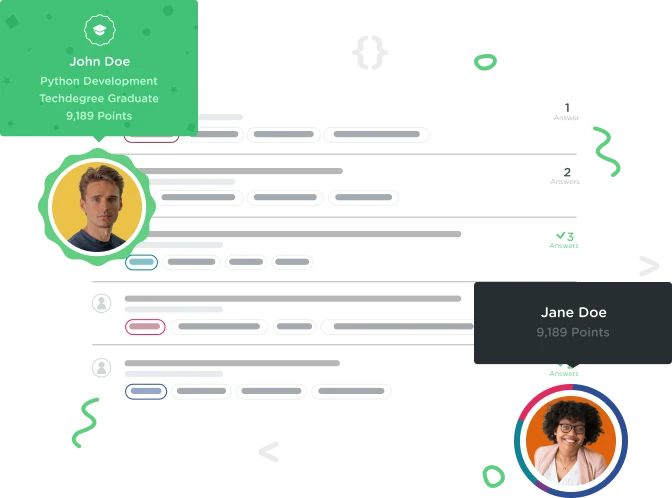
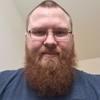
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsReact-hot-loader
Hi,
Trying to use React for my django project, and I finally got it working'ish.
Everytime I make a change to my index.jsx I get a message in the console saying something about I need to do a full reload -> when I do a full reload the react-hot-loader changes the hash which results in my .js file in the bundles folder can't be found anymore since it got a different hash >.<
I find this really annoying because that mean I have to exit the server, run a new build and start it up again every freaking time I make a small change.
I hope someone in here with more experience in react/webpack/node is able to help me.
package.json
{
"name": "my_blog",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"build": "webpack",
"start": "node server.js"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"react": "^15.6.1",
"react-dom": "^15.6.1"
},
"devDependencies": {
"babel": "^6.23.0",
"babel-core": "^6.25.0",
"babel-loader": "^7.1.1",
"babel-preset-es2015": "^6.24.1",
"babel-preset-react": "^6.24.1",
"babelify": "^7.3.0",
"react-hot-loader": "^1.3.1",
"webpack": "^3.1.0",
"webpack-bundle-tracker": "^0.2.0",
"webpack-dev-server": "^2.5.1"
}
}
webpack.config.js
var path = require("path");
var webpack = require("webpack");
var BundleTracker = require("webpack-bundle-tracker");
module.exports = {
context: __dirname,
entry: [
'webpack-dev-server/client?http://localhost:3000',
'webpack/hot/only-dev-server',
'./assets/js/index'
],
output: {
path: path.resolve('./assets/bundles/'),
filename: '[name]-[hash].js'
},
plugins: [
new webpack.HotModuleReplacementPlugin(),
new webpack.NoEmitOnErrorsPlugin(),
new BundleTracker({filename: './webpack-stats.json'})
],
module: {
loaders: [
{
test: /\.jsx?$/,
exclude: /node_modules/,
loader: 'react-hot-loader'
},
{
test: /\.jsx?$/,
exclude: /node_modules/,
loader: 'babel-loader',
query: {
'presets': ['es2015', 'react']
}
}
]
},
resolve: {
modules: ['node_modules'],
extensions: ['.jsx', '.js']
}
};
server.js
var webpack = require('webpack');
var WebpackDevServer = require('webpack-dev-server');
var config = require('./webpack.config.js');
new WebpackDevServer(webpack(config), {
publicPath: config.output.publicPath,
hot: true,
inline: true,
historyApiFallback: true
}).listen(3000, 'localhost', function (err, result) {
if (err) {
return console.log(err);
}
console.log('Listening at http://localhost:3000/');
});
index.jsx
import React from 'react';
import ReactDOM from 'react-dom';
ReactDOM.render(
<h1>Hello, World!</h1>,
document.getElementById('react-app')
);