Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial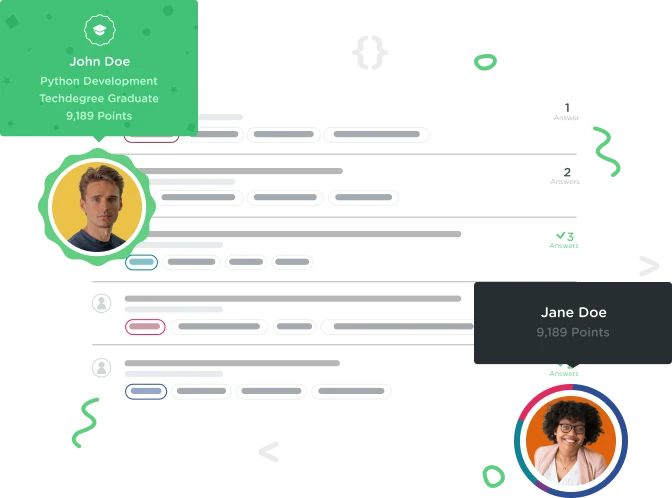

Jeriah Bowers
Front End Web Development Techdegree Graduate 20,590 PointsReact.js "Cannot read property" issue.
Ok, I keep getting the "× TypeError: Cannot read property 'props' of undefined" in the browser. I'll include the App.js and Nav.js files. I know it's probably something simple that I'm missing.
App.js
import React, { Component } from 'react';
import PropTypes from 'prop-types'
import './App.css';
import Search from './Search.js';
import Nav from './Nav.js';
import Photos from './Photos.js'
class App extends Component {
render() {
return (
<div className="main">
<Search />
<Nav name="Cats"/>
<Photos />
</div>
);
}
}
export default App;
Nav.js
import React from 'react'
import PropTypes from 'prop-types'
const Nav = function() {
return (
<div className="nav">
<ul>
<li><a href="#">{this.props.name}</a></li>
<li><a href="#">Dogs</a></li>
<li><a href="#">Birds</a></li>
</ul>
</div>
);
};
export default Nav;
Thanks in advance!!
2 Answers

Seth Kroger
56,413 PointsThe problem is that you haven't defined the variable props for either component, and by that I mean a variable named props
, not the optional definition of what types to expect with the prop-types library.
For a class extending Component, props
is passed as an argument to the constructor (only needed if you need to do something else in your own constructor, like set state or bind methods. Your App component would be good for now, but it's good to know if you need it.)
class App extends Component {
constructor (props) {
super(props);
// ...anything else you need to do here
}
For a pure functional component like Nav, props
is needed as the single argument of the function and will be referred to as props
instead of this.props
.
const Nav = function(props) {
// or with an arrow function
const Nav = props =>
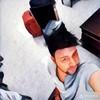
Ari Misha
19,323 PointsHiya there! In App.js file, you called three components Search, Nav with a prop called name and Photos. I see that you're passing down the props correctly to the children component. But if you look at your imports, you've been importing propTypes, and now compiler is expecting propTypes since you passed down props to children. In Nav.js, you need to construct your propTypes for the props received by functional Nav component. Do that and you're good to go. (:
~ Ari