Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial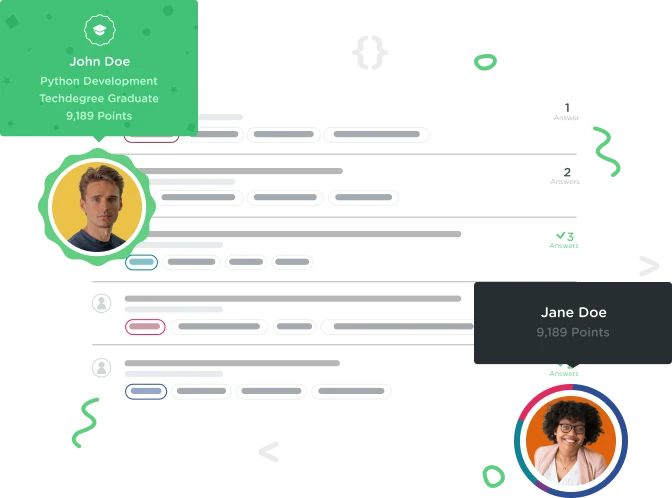
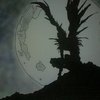
Lucas Santos
19,315 PointsReactJS Confusion
So I looked into the React library create-react-app
and noticed that they define components with ES6 syntax like so:
class myApp extends Component {
render() {
return (
<div>
<h1>Hello World</h1>
</div>
);
}
}
Now in the course we use stateful functional components with React.createClass like this:
var myApp = React.createClass({
propTypes: {
appTitle: React.PropTypes.string.isRequired,
},
render: function(){
<div>
<h1>props.appTitle</h1>
</div>
},
});
My question is what is the difference and how can we define props and/or states on the ES6 method of defining a component? I do NOT mean a default state/prop with getDefaultProps or getInitialState.
Thank you :]
1 Answer
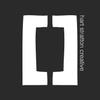
Angelica Hart Lindh
19,465 PointsHey There Lucas,
In ES2015 syntax you would use the constructor() function to setup the state for the 'React.Component'. You can read more about the constructor function in the MDN.
In my example, if you open the console you can see the props defined in the component are passed in the constructor function and this is also where you define the classes state.
Here's a quick jsFiddle example for you.
Hope that helps!
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsI see so all of the states are added in,
Interesting how it's now more like other programming languages now.
Only question I have for you is why did you have to call super(props); in the constructor?
Angelica Hart Lindh
19,465 PointsAngelica Hart Lindh
19,465 PointsThat's spot on Lucas :)
The super() function is used to call functions on an object parent. Therefore, in order to use this in the 'constructor' function you must reference the super() function first so that you are using the 'this' object of the class and not the constructor function itself. We pass 'props' into the constructor and the super in order to access them when the class is invoked.
E.g. The below example would throw an error as the super() function is called after you are using the this keyword.
Also note, you only needs to pass props into the constructor and super functions if you want to access the props there. Otherwise there is no need to pass them into those functions.
Cheers,
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsInteresting I see, so does the rule of calling the super function to get the correct reference of this apply all around ES6 classes regardless if you're using React or not?
Angelica Hart Lindh
19,465 PointsAngelica Hart Lindh
19,465 PointsExactly Lucas, it's a feature of ES2015
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsAwesome, thank you so much for taking the time to explain, appreciate it!