Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial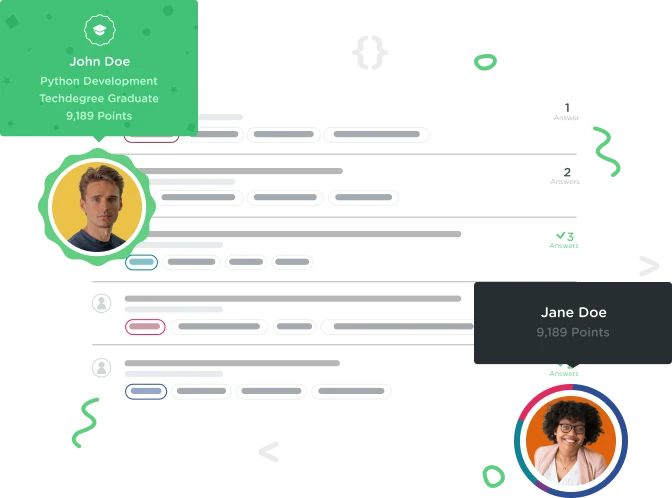
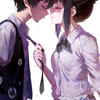
olliecee2
1,550 PointsReact-Router - HashRouter Does Not Work with 2+ Routes
In version 4.0 of 'react-router' they changed up the way hash routes work.
Now we import HashRouter from 'react-router-dom' and use <HashRouter> to do hash routing.
Currently I have a
<HashRouter>
<Route path="/" component={Home}/>
</HashRouter>
But when you add 2 or more routes with <HashRouter> it throws an error saying you can only have 1 per Router. I'm confused as to how I should made more <Route> to my Router.
<HashRouter>
<Route path="/" component={Home} />
<Route path="about" component={About} />
<Route path="teachers" component={Teachers} />
<Route path="courses" component={Courses} />
</HashRouter>
According to the React Router tutorial, I should be placing my <Route> inside of the Router.
Though, I'm getting "A <Router> may have only one child element"
According to the React Router - HashRouting API page I need to specify the entire component? I'm not sure.
3 Answers

Tomas Svojanovsky
26,680 PointsJust Tip. I had problem with rendering components. I needed to add "exact" parameter like this
<Route exact path="/" component={Home} />

Kristóf Dombi
28,047 PointsAs I'm writing this on the 5th of April 2017. This worked for me:
Add /
before the path value.
Here is how it worked for me:
import { HashRouter as Router, Route } from 'react-router-dom';
import App from './components/App';
import Home from './components/Home';
import About from './components/About';
import Courses from './components/Courses';
import Teachers from './components/Teachers';
render((
<Router>
<div>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/courses" component={Courses} />
<Route path="/teachers" component={Teachers} />
</div>
</Router>
), document.getElementById('root'));

Seth Kroger
56,413 PointsAccording to the docs the single child is a usually a <div>
and you put the <Route>
s inside it:
<HashRouter>
<div>
<Route path="/" component={Home} />
<Route path="about" component={About} />
<Route path="teachers" component={Teachers} />
<Route path="courses" component={Courses} />
</div>
</HashRouter>