Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial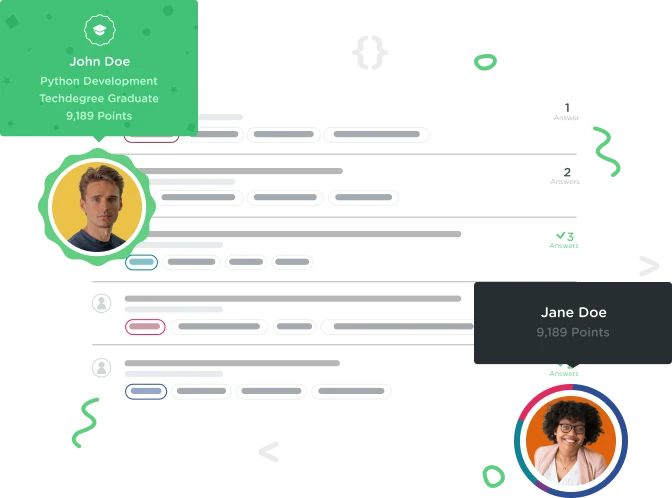
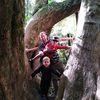
Brett McGregor
Courses Plus Student 1,903 Pointsread JSON file error: " list indices must be integers or slices, not str"
So, here is my code to read a JSON file.
import json
with open("example.json") as file:
db = json.load(file)
print(db["supplier"])
and here is the JSON file I found to work with:
[{
"_id": {
"$oid": "5968dd23fc13ae04d9000001"
},
"product_name": "sildenafil citrate",
"supplier": "Wisozk Inc",
"quantity": 261,
"unit_cost": "$10.47"
}, {
"_id": {
"$oid": "5968dd23fc13ae04d9000002"
},
"product_name": "Mountain Juniperus ashei",
"supplier": "Keebler-Hilpert",
"quantity": 292,
"unit_cost": "$8.74"
}, {
"_id": {
"$oid": "5968dd23fc13ae04d9000003"
},
"product_name": "Dextromathorphan HBr",
"supplier": "Schmitt-Weissnat",
"quantity": 211,
"unit_cost": "$20.53"
}]
Python gives me the following error:
Traceback (most recent call last):
File "C:/Python36-32/Scripts/read_JSON.py", line 5, in <module>
print(db["supplier"])
TypeError: list indices must be integers or slices, not str
My code is exactly the same as Kenneth used in the video. I guess the JSON file I am using is somehow different to the one Kenneth used in his example.
1 Answer
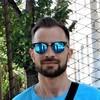
Oszkár Fehér
Treehouse Project ReviewerHi Brett. It gives you that error because when you print the "db" variable with the keyword "supplier" it looks in a dictionary
print(db["supplier"])
but "db" actually is a list, and on list you can use or integer or slices, like
db[0]
where the number indicates the position of the item but if you cal
db[0]["supplier"]
then it will look for the first item keyword "supplier" and gives you back one item. I checked for slices and i'm not sure why it gives error for slice for ex:
db[:]["supplier"]
So the solution if you want all the suppliers from the json file you have to make a for loop on "db"
items = []
for item in db:
items.append(item['supplier'])
print(items)
or
print([db_item["supplier"] for db_item in db])
And you get the desired result. I hope it helps you to understand. Happy coding
Brett McGregor
Courses Plus Student 1,903 PointsBrett McGregor
Courses Plus Student 1,903 PointsI have just noticed that my JSON file is enclosed in square brackets. I suppose that makes it a list, which explains my error.
I tried with a different JSON file and was able to return data.
I guess I need to learn more about this topic!!!