Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial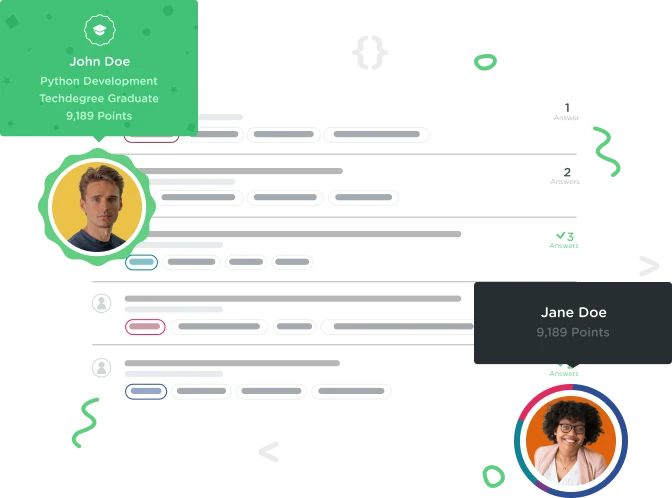
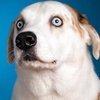
Amy Hsieh
6,023 PointsRead these codes, my answer is 1234, but it's wrong. Can anyone point out my mistakes?
What does the following code display?
$numbers = array(1,2,3,4); $total = count($numbers); $sum = 0; $output = ""; $loop = 0;
foreach($numbers as $number) { $loop = $loop + 1; if ($loop < $total) { $output = $number . $output; } }
echo $output;
?>
2 Answers
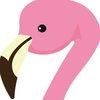
Dave StSomeWhere
19,870 PointsThe actual output is "321" not "1234"
You have 2 mistakes that pop out.
- The loop checks for less than 4, not less than or equal to 4, so it never processes the 4th array entry
- The concat is putting the current value of $number before the previous values -
$output = $number . $output;
.
If you run the following code with some extra echo
statements, hopefully you can see the inner details:
<?php
$numbers = array(1,2,3,4);
$total = count($numbers);
$sum = 0;
$output = "";
$loop = 0;
foreach($numbers as $number) {
echo "<br>In For Each, number is $number";
$loop = $loop + 1;
if ($loop < $total) {
echo "<br>In If loop is $loop";
echo "<br>In If total is $total";
echo "<br>In If, output before is $output";
$output = $number . $output;
echo "<br>In If, output after is $output";
}
}
echo "<br> <br>";
echo $output;
?>
Which produces the following output - notice the final output is "321" not "1234":
In For Each, number is 1
In If loop is 1
In If total is 4
In If, output before is
In If, output after is 1
In For Each, number is 2
In If loop is 2
In If total is 4
In If, output before is 1
In If, output after is 21
In For Each, number is 3
In If loop is 3
In If total is 4
In If, output before is 21
In If, output after is 321
In For Each, number is 4
321

James Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsHi Amy,
I'll try to break down the stages of the loop, so you can understand what is happening.
First time around the loop:
<?php
$output = ""
$total = 4;
$loop = 0 + 1; // 1
// is $loop < $total (is 1 less than 4)? Yes, so add $number to the beginning of $output (known as prepending)
// $output = "1"
// Go around the loop again.
?>
Second time around the loop:
<?php
$output = "1"
$total = 4;
$loop = 1 + 1; // 2
// Is $loop < $total (is 2 less than 4)? Yes, so prepend $number to $output,
//$output = "21"
// Go around the loop again.
?>
3rd Time around the loop
<?php
$output = "21"
$total = 4;
$loop = 2 + 1 // 3
// Is $loop < $total (is 3 less than 4)? Yes, so prepend $number to $output
// $output = "321"
// Go around the loop again.
?>
4th Time around the loop
<?php
$output = "321"
$total = 4;
$loop = 3 + 1 // 4
// Is $loop < $total (is 4 less then 4)? No, so exit the loop.
// Note: We don't output anything this time, because we have exited the loop before reaching the echo statement.
?>
Finally we echo out $output, which contains the string "321"
The tricky thing here, besides the loop itself, is the way we prepend (rather than append) $number to the $output string
If you were to change the line
$output = $number . $output;
to
$output = $output . $number
You would get "123" instead. As the number will be added to the end of the string, rather than the beginning.
I added some echo statements to the code, so you can see what is happening each time around the loop:
<?php
$numbers = array(1,2,3,4);
$total = count($numbers);
$sum = 0;
$output = "";
$loop = 0;
foreach($numbers as $number) {
$loop = $loop + 1;
if ($loop < $total) {
$output = $number . $output;
echo "\$loop = " . $loop . ", ";
echo "\$number = " . $number . ", ";
echo "\$total = " . $total . ", ";
echo "\$output = " . $output . ", ";
echo "<br/>";
}
}
echo $output;
?>
Hope this helps :)