Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial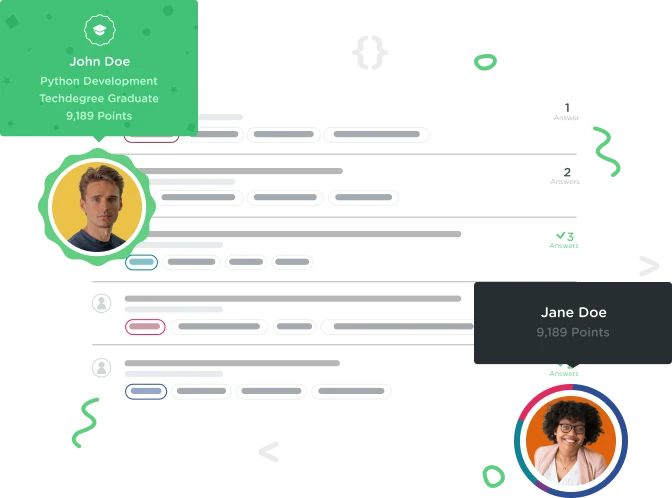

Unsubscribed User
8,838 PointsReading input in Go
I have taken the Introduction to Go
course and I am very interested.
Something not mentioned in the course is how one reads input from the stdin. How do you do this?
I know in Python you may do:
input = input("Write here > ")
print(input)
Anything similar in Go?
Thanks
1 Answer

Joe Purdy
23,237 PointsMost of the time I just use the bufio package for when I need to read input.
Here's a basic example:
reader := bufio.NewReader(os.Stdin)
fmt.Print("Write here > ")
input, _ := reader.ReadString('\n')
fmt.Println(input)
First you create a new reader from stdin using bufio.NewReader(os.Stdin)
. Then you print whatever helper text you want to use as a prompt to the user. To get the input you assign the result of the ReadString method of your new reader to a variable. Passing '\n
' as the delim argument stops reading the string when a newline is encountered, like a user pressing enter.
The ReadString method returns two values, first the string value that was read followed by an error value. Because my example uses an underscore for the error value there's no error checking, you should probably do some kind of error check/handling, but that's just a quick example.
Unsubscribed User
8,838 PointsUnsubscribed User
8,838 PointsThis makes alot of sense, thanks!
What is the difference between
io
andbufio
though, I don't get this.Joe Purdy
23,237 PointsJoe Purdy
23,237 PointsSounds like you're asking what the difference between buffered vs unbuffered I/O is. I'll do my best to explain it as I understand it.
The key difference is the use of a buffer or not when reading or writing to a file. The term "buffer" refers to a place we read or write our bytes to before we actually make a system call to put them on a physically medium, like the hard disk. Using buffers is useful when working with large files as we buffer up our data in chunks to reduce the amount of memory usage as well as the write and read calls.
For your case of reading an input string you're probably unlikely to run into a scenario where the input is large enough to benefit significantly from being read into a buffer, however in Go there isn't a cost to using buffers with the
bufio
package so it's worth using for the habit of best practices.Also you'll find that the bufio package from the standard library contains many helper methods for working with I/O whereas the io package is lower level and really only offers up the
io.Reader
andio.Writer
interfaces which then need to be implemented on your own.Hope that helps shed some light on how these packages from the standard library operate.