Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial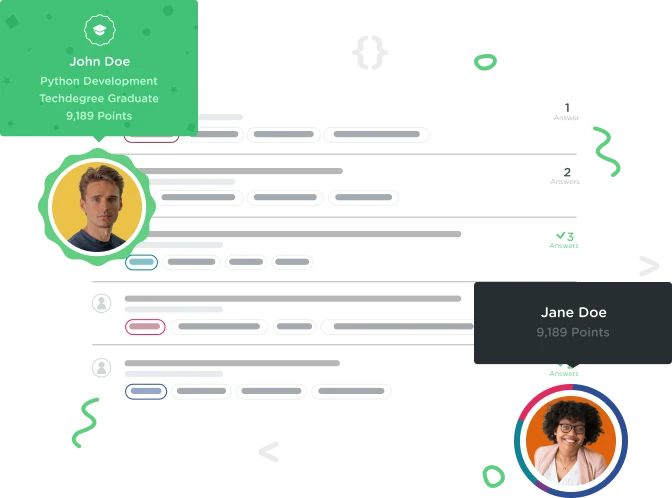
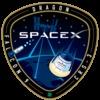
Sarantis Tutzarakis
8,480 PointsReally do not get what I am doing wrong here, perhaps missing an initialization to a method somewhere?
Cannot complete the 4th task... NullPointerException error
public class Forum {
public Forum(String topic) {
topic = topic;
}
private String topic;
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class User {
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
firstName = firstName;
lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
//add this constructor
public ForumPost(User author, String title, String description) {
author = author;
title = title;
description = description;
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
//Take the first two elements passed args
User author = new User(args[0], args[1]);
//Add the author, title and description
ForumPost post = new ForumPost(author, "Java", "Obj.");
forum.addPost(post);
}
}
1 Answer
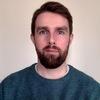
Richard Lambert
Courses Plus Student 7,180 PointsHello mate,
When initializing member variables within the constructor, prefix them with this.
in order to prevent naming conflicts (1). Also, it is best practice to declare variables at the beginning of a class definition (2).
public class Forum {
public Forum(String topic) {
topic = topic; // (1) NAMING CONFLICT
}
private String topic; // (2) DECLARE AT BEGINNING OF CLASS DEFINITION
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class User {
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
firstName = firstName; // (1) NAMING CONFLICT
lastName = lastName; // (1) NAMING CONFLICT
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
//add this constructor
public ForumPost(User author, String title, String description) {
author = author; // (1) NAMING CONFLICT
title = title; // (1) NAMING CONFLICT
description = description; // (1) NAMING CONFLICT
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
Hope this helps
andren
28,558 Pointsandren
28,558 PointsOn top of the issues Richard has pointed out (which are correct) I also notice that you have changed what the
addPost
method prints out, which is not something the challenge asks you to do and which will also cause the challenge to fail.The method is supposed to look like this:
In order to pass the challenge you have to both fix the issues Richard pointed out, and fix the
addPost
method so that it looks exactly like the one I posted above.Richard Lambert
Courses Plus Student 7,180 PointsRichard Lambert
Courses Plus Student 7,180 PointsWell spotted andren ;D